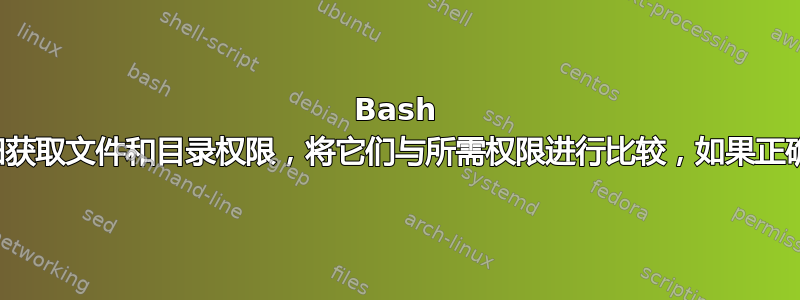
我正在尝试编写一个脚本来检索一组文件和目录的权限。然后检查各个权限是否设置正确。
如果权限设置不正确,那么我希望它能够回显哪个目录或文件组权限设置不正确。
到目前为止,我已经使用“find”递归查找某个目录内的所有文件和目录,然后执行 stat 返回当前权限。然后,从返回的权限列表中,我使用 if-then 语句检查是否有任何文件或目录具有与预期不同的权限。这是通过 != 运算符并使用模式匹配来完成的。因此,所有文件的权限应设置为 444,目录的权限应设置为 555,如果没有返回,则权限错误。
for site in $(echo /var/www/*)
do
permcheckfile=$(find $site -type f -exec stat -c '%a' '{}' +)
permcheckdir=$(find $site -type d -exec stat -c '%a' '{}' +)
if [[ $permcheckfile != *444 ]]
then
echo "$site file permissions are wrong"
else
echo "$site file permissions are correct"
fi
if [[ $permcheckdir != *555 ]]
then
echo "$site directory permissions are wrong"
else
echo "$site directory permissions are correct"
fi
done
上面的脚本发现的问题是有时它会返回误报,我不知道为什么。
有谁知道我哪里出错了?有没有更好的方法来实现我想要实现的目标?任何帮助或建议将不胜感激。感谢您的时间和帮助
答案1
您需要循环 permcheckfile 和 permcheckdir 数组。
for site in $(echo /var/www/*)
do
for file in $(find $site -type f -exec stat -c '%a' '{}' +)
do
if [[ $file != *444 ]]
then
echo "$site/$file permissions are wrong"
else
echo "$site/$file permissions are correct"
fi
done
for dir in $(find $site -type d -exec stat -c '%a' '{}' +)
do
if [[ $dir != *555 ]]
then
echo "$site directory permissions are wrong"
else
echo "$site directory permissions are correct"
fi
done
done
答案2
您只需调用一次“find”即可实现所需的所有功能,如下所示。它可以进一步优化,但代价是清晰度。
#!/bin/sh
p=$(type -P printf)
site='/var/www'
cd "$site" && \
find . \
\( -type f -perm 444 -exec $p "$site/%s file permissions are correct.\n" {} + \) -o \
\( -type f ! -perm 444 -exec $p "$site/%s file permissions are wrong.\n" {} + \) -o \
\( -type d ! -name . ! -perm 555 -exec $p "$site/%s directory permissions are wrong.\n" {} + \) -o \
\( -type d ! -name . -perm 555 -exec $p "$site/%s directory permissions are correct.\n" {} + \)