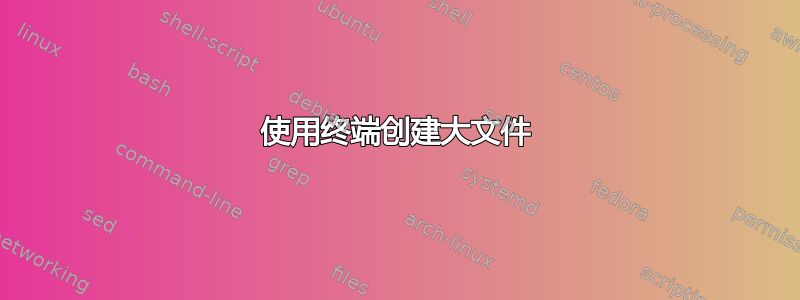
我想制作一个非常大的文件用于测试,该文件应包含唯一单词(严格不重复)。它可以是 GB、TB 等。我可以用终端执行此操作吗?
我在用Ubuntu 16.04(Xenial Xerus)。
答案1
创建无限数量的单词,保证唯一
下面的脚本将从字母表中的字符生成保证唯一的单词。任何固定长度的字符的问题在于,它将产生一个有限的一组可能性,限制文件的大小。
因此我使用了python
's permutations
,它可以产生有限数量的唯一单词。然而使用完所有组合后,我们只需重新开始,打印单词 2、然后 3、four、n
times 等,每个值n
都会创建一个新的唯一单词。因此,我们有一个生成器可以生成 100% 肯定唯一的单词。
剧本:
import itertools
import string
ab = [c for c in string.ascii_lowercase]
t = 1
while True:
for n in range(1, len(ab)+1):
words = itertools.permutations(ab, n)
for word in words:
print(t*("".join(word)))
t += 1
如何使用
- 只需将脚本复制到一个空文件中,然后将其保存为
unique_generator.py
通过命令运行:
python3 /path/to/unique_generator.py > /path/to/bigfile.txt
笔记
该脚本会生成不同长度的唯一单词。如果您愿意,可以通过更改以下行来设置起始长度或最大长度:
for n in range(1, len(ab)+1)
(替换范围的开始),并更改:
while True:
变成(例如):
while t < 10:
在最后一种情况下,单词的长度最多是字母表的 10 倍。
结束进程
- 从终端运行时,只需按Ctrl+C
否则:
kill $(pgrep -f /path/to/unique_generator.py)
应该可以完成这个工作。
答案2
要获取充满随机单词的大文件,请使用以下命令:
cat /dev/urandom | head -c 1000000 | tr -dc "A-Za-z0-9\n" | sort | uniq
这将创建一个文件,每行都有一个独特的单词和随机文本字符串。您可以通过将 1000 变大或变小来增加文件的大小。每个计数大约等于一个字节。
为了使单词之间用空格分隔,只需将它们传递回去即可tr "\n" " "
。
cat /dev/urandom | head -c 1000000 | tr -dc "A-Za-z0-9\n" | sort | uniq | tr "\n" " "
这也避免了与 shell 循环相关的性能问题。
答案3
制作随机字符行的最简单单行代码:
while true; do echo $RANDOM | base64 >> BIGFILE.txt ; done
或者:
while true; do echo $RANDOM | sha512sum >> BIGFILE.txt ; done
为了更好的唯一性,您可以使用/dev/urandom
:
cat /dev/urandom | base64
当文件达到所需大小时,使用 Ctrl+C 终止命令
还请考虑随机字符串/密码生成器:
因此,其中一个答案可以调整为:
while true; do openssl rand -base64 20 ; done
如果你不想使用标点符号和数字,而只使用字母,那么我们可以用来tr
纠正:
while true; do openssl rand -base64 20 | tr -d '[[:digit:]][[:punct:]]' ; done
为了获得额外的随机性,您可以使用 来打乱生成的字符串的字符shuf
。
while true; do openssl rand -base64 20 | fold -w1 | shuf | tr -d '\n' ; done
等等等等。您甚至可以将输出传递给另一组base64
或sha256sum
命令,以使它们更加随机。
对于那些喜欢除 shell 之外的其他语言的人来说,这里有一个 python 单行命令:
python -c $'import string,random;i = [i for i in string.uppercase + string.lowercase + string.digits];\nwhile not random.shuffle(i): print "".join(i)'
答案4
您还可以/proc/sys/kernel/random/uuid
在 Linux 中使用来生成UUID,应保证其唯一:
[~]$ for i in {1..10}; do cat /proc/sys/kernel/random/uuid; done
c8072c40-32f5-4f14-8794-c3ab68e1a0f5
2f2630d8-0e17-4cba-8e62-586ee23f0ebb
97606886-f227-46f6-827a-141b0db57c59
5ffea57c-c3bf-4ba6-8c08-8a1b29ee8f6c
2b90f797-2def-4433-ae71-6f404db944fc
fcb793e9-6102-472d-a7a0-7bf5204dbee5
d84e2877-6804-4bed-85f0-0a551234425a
3d9445ca-335c-4960-83d5-6cb1bef8b9eb
913bce71-5c20-47f7-a22e-277be6856a57
8f232541-f8c1-46ba-b57a-0d11314c3483
您可以删除 - 字符tr -d
,然后将其重定向到文件:
[~]$ for i in {1..10000}; do cat /proc/sys/kernel/random/uuid | tr -d '-' ; done > /tmp/words
[~]$ sort /tmp/words | uniq | wc -l
10000