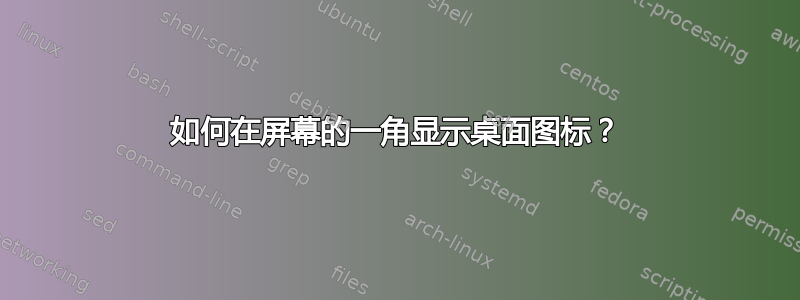
我知道如何在启动器中放置“显示桌面”图标,但我想将其放在角落里。
原因是,在启动器中我必须“小心地”单击它,而如果它在角落,我就可以更“积极”地单击它。
编辑:我已经测试了答案中的某些解决方案,但没有一个能实现我的目标,可能是因为我没有准确地说出来:我想要一个角落里的显示桌面图标(或者不显示,我真的不在乎,如果我只需要将指针移动到角落),这样当我选择它时,就会显示桌面,然后我能行得通在桌面上,例如复制一些文件到那里,打开一个终端等等,然后我就可以回去所有窗口都以相同的图标最小化。
@Serg 的解决方案有两个问题:1)图标已修复,但不在角落。2)它不允许我返回到我最小化的窗口。
@Heynnema 的解决方案的主要问题是图标只是“显示”了桌面,但我实际上无法对其进行操作:如果我右键单击某个空白处以打开终端,则该空间可能并不是真正“空的”,因为如果我在那里打开了一个窗口,则显示的右键单击选项是该窗口在最小化之前的一个选项。
@GautamVashisht 的解决方案似乎与 Heynnema 相同,因为每当我在 CCSM 中激活它时,Hot Corners 就会被激活。
答案1
免责声明:我是这个指标的作者,它是针对这个特定问题编写的
介绍
Ubuntu 默认没有移动“显示桌面”图标的选项 - 它必须存在于启动器上。您也可以让它显示在 Alt+Tab 菜单中。但是,可以创建一个小的指示器小程序,它将位于顶部面板中,这非常接近您将图标放置在屏幕角落的要求。这个答案正好提供了这一点
用法
使用方法非常简单。将代码保存在您的~/bin
文件夹中,例如对我来说就是/home/serg/bin/show_desktop_indicator
。为了使其每次登录 Ubuntu 时都打开,请在 Dash 中搜索“启动应用程序”,打开该应用程序,然后将指示器的完整路径添加为新命令。
您还可以下载zip 文件夹,其中包含来自项目 Github 页面的指标
本质上,它会最小化所有打开的窗口。有两种方法可以实现此目的。一种是,您可以单击指示器图标,然后单击“显示桌面”菜单项,或者使用鼠标中键单击图标本身。
代码
也可在Github
#!/usr/bin/env python3
# -*- coding: utf-8 -*-
#
# Author: Serg Kolo , contact: [email protected]
# Date: November 5th, 2016
# Purpose: appindicator for minimizing all windows
# Written for: http://askubuntu.com/q/846067/295286
# Tested on: Ubuntu 16.04 LTS
#
#
# Licensed under The MIT License (MIT).
# See included LICENSE file or the notice below.
#
# Copyright © 2016 Sergiy Kolodyazhnyy
#
# Permission is hereby granted, free of charge, to any person obtaining a copy
# of this software and associated documentation files (the "Software"), to deal
# in the Software without restriction, including without limitation the rights
# to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
# copies of the Software, and to permit persons to whom the Software is
# furnished to do so, subject to the following conditions:
#
# The above copyright notice and this permission notice shall be included
# in all copies or substantial portions of the Software.
#
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
# IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
# FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
# AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
# LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
# OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
# SOFTWARE.
import gi
gi.require_version('AppIndicator3', '0.1')
gi.require_version('Notify', '0.7')
from gi.repository import GLib as glib
from gi.repository import AppIndicator3 as appindicator
from gi.repository import Gtk as gtk
from gi.repository import Gdk
class ShowDesktop(object):
def __init__(self):
self.app = appindicator.Indicator.new(
'files-indicator', "user-desktop",
appindicator.IndicatorCategory.OTHER
)
self.app.set_status(appindicator.IndicatorStatus.ACTIVE)
self.make_menu()
def add_menu_item(self, menu_obj, item_type, image, label, action, args):
""" dynamic function that can add menu items depending on
the item type and other arguments"""
menu_item, icon = None, None
if item_type is gtk.ImageMenuItem and label:
menu_item = gtk.ImageMenuItem.new_with_label(label)
menu_item.set_always_show_image(True)
if '/' in image:
icon = gtk.Image.new_from_file(image)
else:
icon = gtk.Image.new_from_icon_name(image, 48)
menu_item.set_image(icon)
elif item_type is gtk.ImageMenuItem and not label:
menu_item = gtk.ImageMenuItem()
menu_item.set_always_show_image(True)
if '/' in image:
icon = gtk.Image.new_from_file(image)
else:
icon = gtk.Image.new_from_icon_name(image, 16)
menu_item.set_image(icon)
elif item_type is gtk.MenuItem:
menu_item = gtk.MenuItem(label)
elif item_type is gtk.SeparatorMenuItem:
menu_item = gtk.SeparatorMenuItem()
if action:
menu_item.connect('activate', action, *args)
menu_obj.append(menu_item)
menu_item.show()
def make_menu(self):
self.app_menu = gtk.Menu()
content = [self.app_menu,gtk.MenuItem,
None,'Show Desktop',
self.show_desktop,[None]
]
self.add_menu_item(*content)
last = None
for i in self.app_menu.get_children():
last = i
self.app.set_secondary_activate_target(last)
content = [self.app_menu,gtk.ImageMenuItem,
'exit','Quit',
self.quit,[None]
]
self.add_menu_item(*content)
self.app.set_menu(self.app_menu)
def show_desktop(self,*args):
screen = Gdk.Screen.get_default()
for w in screen.get_window_stack():
w.iconify()
w.process_all_updates()
def run(self):
""" Launches the indicator """
try:
gtk.main()
except KeyboardInterrupt:
pass
def quit(self, *args):
""" closes indicator """
gtk.main_quit()
def main():
""" defines program entry point """
indicator = ShowDesktop()
indicator.run()
if __name__ == '__main__':
try:
main()
except KeyboardInterrupt:
gtk.main_quit()
指示器正在运行:
Ubuntu Kylin 图标主题:
答案2
答案3
目前 Ubuntu 中还没有具体的方法来移动显示桌面启动器中的图标移到角落。但是,您可以使用一些替代方法(解决方法)来访问桌面屏幕!下面列出了其中一些方法:-
1.)Alt + Tab 快捷键
使用Alt + Tab快捷键。它主要用于切换应用程序。当您按下Alt + Tab键,左侧出现的第一个图标是显示桌面图标。您可以标签通过此图标可以访问您的桌面屏幕。
2.)超级+D或者Ctrl + Alt + D快捷键
在 Unity Desktop 中,您可以使用默认 超级+D或者Ctrl + Alt + D快捷键来访问您的桌面屏幕。
3.)使用热角
为此,首先通过 Ubuntu 软件中心或sudo apt-get install unity-tweak-tool
在终端中输入来安装 Unity Tweak Tool。然后打开/启动 Unity Tweak Tool 并单击热角在下面窗口管理器.然后在一般的部分,打开热角及以下行为部分,选择切换桌面在您想要使用的热点角旁边的框中。然后,只要您将光标悬停在该角落,您就会看到您的桌面屏幕。