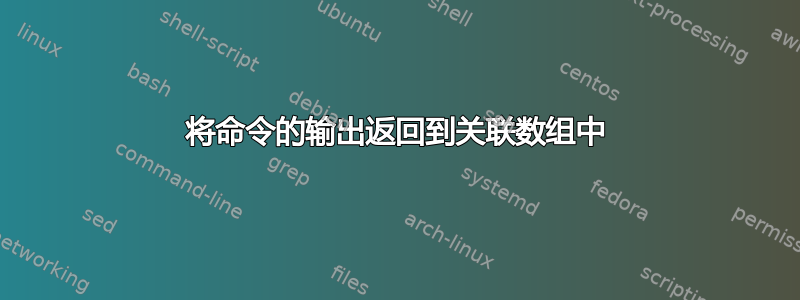
我需要将命令的输出放入关联数组中。
例如:
dig mx +short google.com
将返回:
20 alt1.aspmx.l.google.com.
40 alt3.aspmx.l.google.com.
50 alt4.aspmx.l.google.com.
10 aspmx.l.google.com.
30 alt2.aspmx.l.google.com.
如何使用优先级 (10,20,...) 作为键和记录 (aspmx.l.google.com.) 作为值来创建关联数组?
答案1
以下是将该数据读入 bash 关联数组的一种方法:
代码:
#!/usr/bin/env bash
declare -A hosts
while IFS=" " read -r priority host ; do
hosts["$priority"]="$host"
done < <(dig mx +short google.com)
for priority in "${!hosts[@]}" ; do
echo "$priority -> ${hosts[$priority]}"
done
输出:
20 -> alt1.aspmx.l.google.com.
10 -> aspmx.l.google.com.
50 -> alt4.aspmx.l.google.com.
40 -> alt3.aspmx.l.google.com.
30 -> alt2.aspmx.l.google.com.
答案2
declare -A mx
eval $(dig mx +short google.com | sed "s/'//g; s/^/mx[/; s/ /]='/; s/$/';/")
首先声明一个名为 的关联数组mx
,然后执行dig
并用于sed
将输出转换为关联数组赋值,然后eval
将其转换为当前 shell。该sed
命令去掉所有单引号,然后将变量赋值包装起来,使用单引号引用该值。
答案3
首先,我认为 Stephen Rauch 的解决方案(以及切普纳的评论)很棒。但当谈到关联数组,我几乎总是在 Python 中使用字典,不是因为我不喜欢 Bash,而是因为(在我看来)它们在更高级别的语言中更容易使用。
下面是 Python 中的用法示例。
#!/usr/bin/env python3
import subprocess
import os
import json
digDict = {}
tmpOut = open("tmpOut", "w")
output = subprocess.call(['dig', 'mx', '+short', 'google.com'], stdout=tmpOut)
# Fill the dictionary with the values we want
with open("tmpOut") as infile:
for line in infile:
digDict[line.split()[0]] = line.split()[1]
os.remove("tmpOut")
# Sort the dictionary by key
print("Sorted dictionary:")
for key in sorted(digDict):
print(key + " -> " + digDict[key])
# Get a specific value based on key
print("Access value associated with key '10' (digDict[\"10\"]):")
print(digDict["10"])
# "Pretty print" the dictionary in json format
print("json format:")
print(json.dumps(digDict, sort_keys=True, indent=4))
# Saved the dictionary to file in json format
with open("digDict.json", "w") as fp:
json.dump(digDict, fp, sort_keys=True, indent=4)
exit(0)
执行:
./myDig.py
Sorted dictionary:
10 -> aspmx.l.google.com.
20 -> alt1.aspmx.l.google.com.
30 -> alt2.aspmx.l.google.com.
40 -> alt3.aspmx.l.google.com.
50 -> alt4.aspmx.l.google.com.
Access value associated with key '10' (digDict["10"]):
aspmx.l.google.com.
json format:
{
"10": "aspmx.l.google.com.",
"20": "alt1.aspmx.l.google.com.",
"30": "alt2.aspmx.l.google.com.",
"40": "alt3.aspmx.l.google.com.",
"50": "alt4.aspmx.l.google.com."
}
cat digDict.json
:
{
"10": "aspmx.l.google.com.",
"20": "alt1.aspmx.l.google.com.",
"30": "alt2.aspmx.l.google.com.",
"40": "alt3.aspmx.l.google.com.",
"50": "alt4.aspmx.l.google.com."
}
再次;上面的 Bash 解决方案是伟大的(并由我投票)。这只是 Python 中的另一个例子,仅此而已。
答案4
您可以使用内部字段分隔符将输入分隔成行
IFS="\n" read -ra ADDR <<< "$IN"