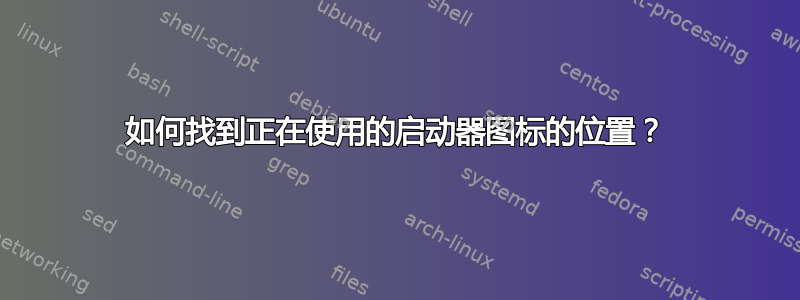
我的桌面上有一个启动器,我想手动添加另一个具有相同图标的启动器。
当我转到现有启动器的首选项并单击图标时,它不会将我带到存储图标的文件夹,而只是带到我的主文件夹。
我如何才能找出启动器使用的图标在系统中的位置?
答案1
大多数情况下,图标将从您当前的图标主题中选择,而不是被称为绝对路径。
打开 Gedit
将启动器拖到 Gedit 窗口中
寻找
Icon
定义:Icon=gnome-panel-launcher
然后你就可以找到图标某处,取决于/usr/share/icons
您的主题。
下面是一个快速的 Python 脚本,可以为您找到正确的图标路径:
import gtk
print "enter the icon name (case sensitive):"
icon_name = raw_input(">>> ")
icon_theme = gtk.icon_theme_get_default()
icon = icon_theme.lookup_icon(icon_name, 48, 0)
if icon:
print icon.get_filename()
else:
print "not found"
将其保存在某处并运行python /path/to/script.py
。
它看上去会像这样:
stefano@lenovo:~$ python test.py
enter the icon name (case sensitive):
>>> gtk-execute
/usr/share/icons/Humanity/actions/48/gtk-execute.svg
或者,您也可以四处寻找,/usr/share/icons
直到找到所需的图标。
更简单:您只需复制并粘贴启动器并更改名称和命令即可
编辑2018
上述脚本的更新版本:
#!/usr/bin/env python3
import gi
gi.require_version('Gtk', '3.0')
from gi.repository import Gtk
icon_name = input("Icon name (case sensitive): ")
icon_theme = Gtk.IconTheme.get_default()
icon = icon_theme.lookup_icon(icon_name, 48, 0)
if icon:
print(icon.get_filename())
else:
print("not found")
答案2
更多信息。
普通启动器实际上是 /usr/share/applications/ 中的 .desktop 文件。
例如:/usr/share/applications/usb-creator-gtk.desktop
(看https://specifications.freedesktop.org/desktop-entry-spec/desktop-entry-spec-latest.html)
每个桌面文件都有一行指定图标,例如:
Icon=usb-creator-gtk
当没有路径(和文件扩展名)(如本例)时,表示在 /usr/share/icons/ 中的某处找到该图标,并且运行时使用的图标取决于当前主题,在某些情况下还取决于显示上下文(大小)。
从桌面文件中知道图标名称(不带扩展名),可以按如下方式找到它/它们:
$ find . -name "usb-creator-gtk*"
./hicolor/scalable/apps/usb-creator-gtk.svg
./Humanity/apps/32/usb-creator-gtk.svg
./Humanity/apps/16/usb-creator-gtk.svg
./Humanity/apps/22/usb-creator-gtk.svg
./Humanity/apps/24/usb-creator-gtk.svg
./Humanity/apps/64/usb-creator-gtk.svg
./Humanity/apps/48/usb-creator-gtk.svg
答案3
#!/usr/bin/env python3
from gi.repository import Gtk
icon_name = input("Icon name (case sensitive): ")
if icon_name:
theme = Gtk.IconTheme.get_default()
found_icons = set()
for res in range(0, 512, 2):
icon = theme.lookup_icon(icon_name, res, 0)
if icon:
found_icons.add(icon.get_filename())
if found_icons:
print("\n".join(found_icons))
else:
print(icon_name, "was not found")
将以上内容保存到文件中并使用 运行python3 /path/to/file
。
之间的差异斯蒂法诺·帕拉佐原来的剧本是这样的:
- 这将找到图标的所有分辨率(不只是 48)
- 用途
gi.repository
代替Gtk
- 使用 Python 3 而不是 2
- 在其他方面略有调整
答案4
已将此作为 unix.stackexchange 上问题的奖励添加,并意识到在原始线程中分享它也不错...所以
这是另一个修改版本,它结合了 Stefano Palazzo 的 2018 版本和 kiri 版本的一些元素以及我自己的更改(脚本顶部的一些注释文档)。
#!/usr/bin/env python3
# ==========================================================================================
# This script is for looking up an icon file path based on the icon name from a *.desktop file.
# Parts of it are based on snippets provided by Stefano Palazzo and kiri on askubuntu.com
# https://askubuntu.com/questions/52430/how-can-i-find-the-location-of-an-icon-of-a-launcher-in-use
# ==========================================================================================
# The original version(s) simply prompted the user for the icon name.
# However, I have modified this version in the following ways:
# 1. Added ability to pass specific size as arg (e.g. --size=22 or --size=48, etc)
# 2. Added ability to pass icon names as arg (any other arg besides --size)
# Note: if --size is used with multiple icon names, then it is assummed
# that all of the icons in the search will be for the same size
# 3. Like kiri's version, I removed the hard-coded size of 48x48 and default to all sizes
# 4. Unlike kiri's version, you can optionally still search for a specific size (using #1)
# 5. Performance improvements from kiri's version (he was checking every even number from
# 0 to 500 -- so 250 iterations. I base mine off the values that actually existing under
# /etc/share/icons/hicolor - of which there were 17. So his is more flexible but
# mine should be quicker and more forgiving in terms of HDD wear and tear)
# ==========================================================================================
import gi
import sys
import array as arr
gi.require_version('Gtk', '3.0')
from gi.repository import Gtk
def resolveIconPath( iconName, iconSize = -1 ):
"This takes a freedesktop.org icon name and prints the GTK 3.0 resolved file location."
iconTheme = Gtk.IconTheme.get_default()
# if looking up a specific size
if iconSize >= 16:
msgTemplate = "iconname: \"" + iconName + "\" (size: " + str(iconSize) + "): "
iconFile = iconTheme.lookup_icon(iconName, iconSize, 0)
if iconFile:
print(msgTemplate + iconFile.get_filename() + "\n")
else:
print("W:" + msgTemplate + " No matching path(s) found.\n")
else:
# otherwise, look up *all* sizes that can be found
sep="===================================================================="
msgTemplate = sep + "\niconname: \"" + iconName + "\":\n" + sep
foundIconsList = list()
for resolution in [16, 20, 22, 24, 28, 32, 36, 48, 64, 72, 96, 128, 192, 256, 480, 512, 1024]:
iconFile = iconTheme.lookup_icon(iconName, resolution, 0)
if iconFile:
filePath=str(iconFile.get_filename())
if not (filePath in foundIconsList):
foundIconsList.append(iconFile.get_filename())
if foundIconsList:
print(msgTemplate + "\n"+ "\n".join(foundIconsList)+ "\n")
else:
print("W: iconname: \"" + iconName + "\": No matching path(s) found.\n")
return
# get the total number of args passed (excluding first arg which is the script name)
argumentsLen = len(sys.argv) - 1
# define a list for storing all passed icon names
iconNamesList = []
# loop through passed args, if we have any and handle appropriately
showHelp=False
size=-1
if argumentsLen > 0:
for i in range(1, len(sys.argv)):
arg=str(sys.argv[i])
#print(i, "arg: " + arg)
if arg.startswith('--size=') or arg.startswith('-s=') or arg.startswith('-S='):
tmpSize=(arg.split("=",2))[1]
if len(tmpSize) > 0 and tmpSize.isnumeric():
size=int(tmpSize)
else:
print("Invalid size '" + tmpSize + "'; Expected --size=xx where xx is a positive integer.")
elif arg == '--help' or arg == '-h':
print(str(sys.argv[0]) + " [OPTIONS] [ICON_NAME]\n")
print("Takes a freedesktop.org/GNOME icon name, as commonly appears in a *.desktop file,")
print("and performs a lookup to determine matching filesystem path(s). By default, this")
print("path resolution is determined for all available icon sizes. However, a specific")
print("size can be used by providing one of the options below.\n")
print("OPTIONS:")
print(" -s=n, --size=n Restricts path resolution to icons matching a specific size.")
print(" The value n must be a positive integer correspending to icon size.")
print(" When using this option with multiple passed icon names, the size")
print(" restrictions are applied to *all* of the resolved icons. Querying")
print(" different sizes for different icons is only possible via the use of")
print(" multiple calls or by parsing the default output.\n")
print(" -h, --help Display this help page and exit.\n")
exit()
else:
iconNamesList.append(arg)
# if no icon names were passed on command line, then prompt user
if len(iconNamesList) == 0:
iconNamesList.append(input("Icon name (case sensitive): "))
#print("size: " + str(size))
#print("iconNamesList: ")
if len(iconNamesList) > 0:
for iconName in iconNamesList:
if size < 16:
# find all sizes (on my system, 16x16 was the smallest size icons under hicolor)
resolveIconPath(iconName)
else:
# use custom size
resolveIconPath(iconName, size)
用法:
# view help
get-icon-path.py --help
# lookup all matching icons
get-icon-path.py firefox
# lookup all matching icons for a specific size (16x16)
get-icon-path.py --size=16 firefox
# lookup specific size for multiple icons in one command
get-icon-path.py --size=16 firefox gimp
# install to system for frequent usage
sudo cp get-icon-path.py /usr/bin/get-icon-path.py
sudo chown root:root /usr/bin/get-icon-path.py;
sudo chmod 755 /usr/bin/get-icon-path.py;
test ! -f /usr/bin/icon && ln -s /usr/bin/get-icon-path.py /usr/bin/icon;