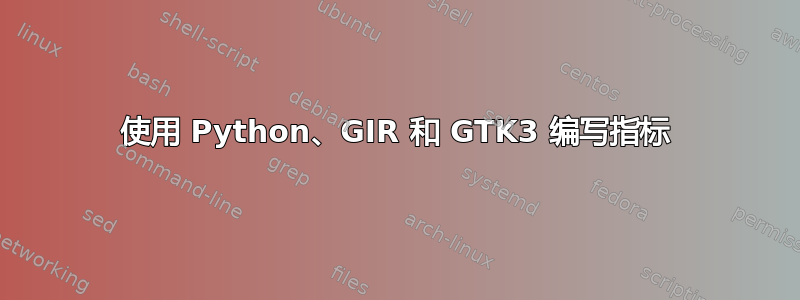
我正在编写一个需要使用指示器的应用程序。我过去曾使用 PyGTK 和 GTK2 完成此操作,并参考了此文档:https://wiki.ubuntu.com/DesktopExperienceTeam/ApplicationIndicators#Python_version
但是,这只适用于 PyGTK 和 GTK2。从那时起,情况发生了变化,我需要找到一些好的文档、教程或好的例子来了解它的工作原理。
此外,前面提到的文档根本没有描述如何向指标添加子菜单。我希望有人能对此进行一些说明,以及如何使用同一工具与类别指标集成。
谢谢。
答案1
这这是我的 gtk3 和 appindicator 的试用代码,它创建了一个指标格氏膏。
基本上,
from gi.repository import AppIndicator3 as AppIndicator
为了使用包提供的 gtk3 应用程序的 appindicator gir1.2-appindicator3
。
这里是应用指标3文档。
pygtk将被 Gtk3 弃用,你必须经历GObject 自省使用 Python 开发 Gtk3 应用程序的路线。您可以参考PyGObject 文档。 代替
import pygtk, gtk, gdk, gobject, pango
你应该做的
from gi.repository import Gtk, Gdk, Pango, GObject
为了研究工作代码,你可以查看卡扎姆从 gtk2 移至 gtk3,并使用appindicator3。
还有一个包gir1.2-appindicator
似乎与使用相同,python-appindicator
因为它们都为 gtk2 应用程序提供用法:
from gi.repository import AppIndicator
或者
import appindicator
一些信息这篇博文也一样。
答案2
这是一个非常简单的脚手架应用程序,它有一个配置窗口、一个主窗口和一个应用程序指示器。
#!/usr/bin/env python3.3
from gi.repository import Gtk
from gi.repository import AppIndicator3 as appindicator
class MyIndicator:
def __init__(self, root):
self.app = root
self.ind = appindicator.Indicator.new(
self.app.name,
"indicator-messages",
appindicator.IndicatorCategory.APPLICATION_STATUS)
self.ind.set_status (appindicator.IndicatorStatus.ACTIVE)
self.menu = Gtk.Menu()
item = Gtk.MenuItem()
item.set_label("Main Window")
item.connect("activate", self.app.main_win.cb_show, '')
self.menu.append(item)
item = Gtk.MenuItem()
item.set_label("Configuration")
item.connect("activate", self.app.conf_win.cb_show, '')
self.menu.append(item)
item = Gtk.MenuItem()
item.set_label("Exit")
item.connect("activate", self.cb_exit, '')
self.menu.append(item)
self.menu.show_all()
self.ind.set_menu(self.menu)
def cb_exit(self, w, data):
Gtk.main_quit()
class MyConfigWin(Gtk.Window):
def __init__(self, root):
super().__init__()
self.app = root
self.set_title(self.app.name + ' Config Window')
def cb_show(self, w, data):
self.show()
class MyMainWin(Gtk.Window):
def __init__(self, root):
super().__init__()
self.app = root
self.set_title(self.app.name)
def cb_show(self, w, data):
self.show()
class MyApp(Gtk.Application):
def __init__(self, app_name):
super().__init__()
self.name = app_name
self.main_win = MyMainWin(self)
self.conf_win = MyConfigWin(self)
self.indicator = MyIndicator(self)
def run(self):
Gtk.main()
if __name__ == '__main__':
app = MyApp('Scaffold')
app.run()
答案3
以防有人觉得它有用,我用 Python、GIR 和 GTK3 制作了一个最小的应用程序指示器。它每隔几秒从 /proc/cpuinfo 读取 CPU 速度并显示它们。
答案4
下面是读取 CPU 温度的示例。在脚本目录中复制一个名为 temp-icon.png/svg 的图标
from gi.repository import Gtk, GLib
from gi.repository import AppIndicator3 as appindicator
import os
def cb_exit(w, data):
Gtk.main_quit()
def cb_readcputemp(ind_app):
# get CPU temp
fileh = open(
'/sys/devices/platform/thinkpad_hwmon/subsystem/devices/coretemp.0/temp1_input',
'r')
ind_app.set_label(fileh.read(2), '')
fileh.close()
return 1
ind_app = appindicator.Indicator.new_with_path (
"cputemp-indicator",
"temp-icon",
appindicator.IndicatorCategory.APPLICATION_STATUS,
os.path.dirname(os.path.realpath(__file__)))
ind_app.set_status (appindicator.IndicatorStatus.ACTIVE)
# create a menu
menu = Gtk.Menu()
menu_items = Gtk.MenuItem("Exit")
menu.append(menu_items)
menu_items.connect("activate", cb_exit, '')
menu_items.show()
ind_app.set_menu(menu)
GLib.timeout_add(500, cb_readcputemp, ind_app)
Gtk.main()