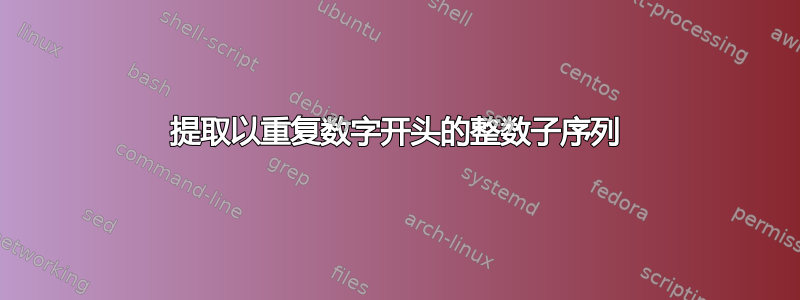
我有一个包含单列整数的文件。我想从此文件中提取所有连续子序列(即以连续顺序出现的子序列)的列表,这些子序列连续两次以相同的数字开头,长度为 12 个整数(包括重叠子序列)。
此外,文件中的任何非整数行都应被忽略/删除,并且如果任何序列在达到 12 个整数之前到达输入末尾,则仍应输出缩短的序列。
例如,假设我的输入文件包含以下数据:
1
junk
1
1
2
3
4
4
5
6
7
8
9
10
11
12
13
14
15
15
16
那么该解决方案应产生以下输出:
1 1 1 2 3 4 4 5 6 7 8 9
1 1 2 3 4 4 5 6 7 8 9 10
4 4 5 6 7 8 9 10 11 12 13 14
15 15 16
请注意,该junk
行和空行被忽略,因此前三1
行被视为连续的。
答案1
这是一个可以执行您想要的操作的 Python 脚本:
#!/usr/bin/env python2
# -*- coding: ascii -*-
"""extract_subsequences.py"""
import sys
import re
# Open the file
with open(sys.argv[1]) as file_handle:
# Read the data from the file
# Remove white-space and ignore non-integers
numbers = [
line.strip()
for line in file_handle.readlines()
if re.match("^\d+$", line)
]
# Set a lower bound so that we can output multiple lists
lower_bound = 0
while lower_bound < len(numbers)-1:
# Find the "start index" where the same number
# occurs twice at consecutive locations
start_index = -1
for i in range(lower_bound, len(numbers)-1):
if numbers[i] == numbers[i+1]:
start_index = i
break
# If a "start index" is found, print out the two rows
# values and the next 10 rows as well
if start_index >= lower_bound:
upper_bound = min(start_index+12, len(numbers))
print(' '.join(numbers[start_index:upper_bound]))
# Update the lower bound
lower_bound = start_index + 1
# If no "start index" is found then we're done
else:
break
假设您的数据位于名为data.txt
.然后你可以像这样运行这个脚本:
python extract_subsequences.py data.txt
假设您的输入文件data.txt
如下所示:
1
1
1
2
3
4
5
6
7
8
9
10
11
12
那么你的输出将如下所示:
1 1 1 2 3 4 5 6 7 8 9 10
1 1 2 3 4 5 6 7 8 9 10 11
要将输出保存到文件,请使用输出重定向:
python extract_subsequences.py data.txt > output.txt
答案2
AWK
方法:
只考虑第一次遇到的2个相同的连续数字,即适合多次提取,但不考虑2个相同的连续数字可能进入处理后的切片下的后续10个数字序列的情况。
awk 'NR==n && $1==v{ print v ORS $1 > "file"++c; tail=n+11; next }
{ v=$1; n=NR+1 }NR<tail{ print > "file"c }' file
答案3
第一个变体-O(n)
awk '
/^[0-9]+$/{
arr[cnt++] = $0;
}
END {
for(i = 1; i < cnt; i++) {
if(arr[i] != arr[i - 1])
continue;
last_element = i + 11;
for(j = i - 1; j < cnt && j < last_element; j++) {
printf arr[j] " ";
}
print "";
}
}' input.txt
第二种变体 - O(n * n)
awk '
BEGIN {
cnt = 0;
}
/^[0-9]+$/{
if(prev == $0) {
arr[cnt] = prev;
cnt_arr[cnt]++;
cnt++;
}
for(i = 0; i < cnt; i++) {
if(cnt_arr[i] < 12) {
arr[i] = arr[i] " " $0;
cnt_arr[i]++;
}
}
prev = $0;
}
END {
for(i = 0; i < cnt; i++)
print arr[i];
}' input.txt
输出
1 1 1 2 3 4 4 5 6 7 8 9
1 1 2 3 4 4 5 6 7 8 9 10
4 4 5 6 7 8 9 10 11 12 13 14
15 15 16