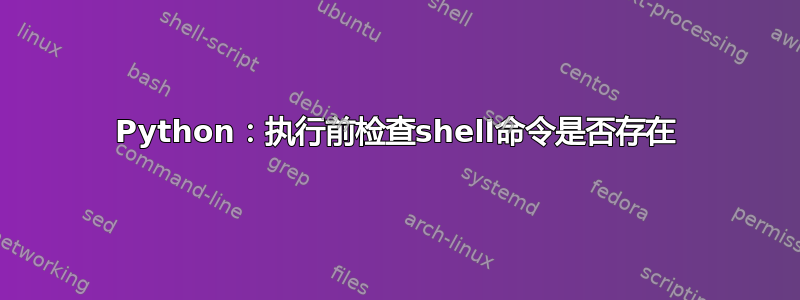
我正在尝试找到一种方法来在执行 shell 命令之前检查它是否存在。
例如,我将执行命令 ack-grep。因此,我尝试执行以下操作:
导入子进程
从子进程导入 PIPE
cmd_grep = subprocess.Popen(["ack-grep", "--no-color", "--max-count=1", "--no-group", "def run_main", "../cgedit/"], stdout=PIPE, stderr=PIPE)
如果我执行
cmd_grep.stderr.读取()
我收到类似输出的 ''。但我的路径上没有命令 ack-grep。那么,为什么 Popen 没有将错误消息放在我的 .stderr 变量上?
此外,有没有更简单的方法来完成我想做的事情?
答案1
您可以使用子进程Python 3 下的模块或命令Python 2 的模块如下:
状态,结果 = 子进程.getstatusoutput(“ls -al”)
状态,结果 = 命令.getstatusoutput("ls -al")
然后测试的值status
。
来自网站的示例:
>>> 导入子进程 >>> 子进程.getstatusoutput('ls /bin/ls') (0,'/bin/ls') >>> 子进程.getstatusoutput('cat /bin/junk') (256,‘cat:/bin/junk:没有此文件或目录’) >>> 子进程.getstatusoutput('/bin/junk') (256,‘sh:/bin/junk:未找到’)
答案2
您不能以某种方式使用“which”命令吗?which 命令会自动在路径中查找应用程序。我认为您只需要调用此命令并传递要查找的命令的名称,然后解析结果。
答案3
这应该对你有用:
import subprocess
from subprocess import PIPE
import shutil
cmd = ["ack-grep", "--no-color", "--max-count=1", "--no-group", "def run_main", "../cgedit/"]
path_to_cmd = shutil.which(cmd[0])
if path_to_cmd is None:
print(f"There is no {cmd[0]} on the path!")
else:
cmd_grep = subprocess.Popen(cmd, stdout=PIPE, stderr=PIPE)
思考过程........我用这个解决方案来开始:
import subprocess
status, response = subprocess.getstatusoutput('which ack-grep')
print(f'Exitcode: {status}, Output: {response}')
但这只会导致 Linux 系统做出特定响应。
import shutil
shutil.which(cmd[0])
当调用“ack-grep”时返回可执行文件的路径,如果没有调用任何内容则返回 None。
答案4
对于这种情况,我使用:
def find_program(prog_filename, error_on_missing=False):
bdirs = ['$HOME/Environment/local/bin/',
'$HOME/bin/',
'/share/apps/bin/',
'/usr/local/bin/',
'/usr/bin/']
paths_tried = []
for d in bdirs:
p = os.path.expandvars(os.path.join(d, prog_filename))
paths_tried.append(p)
if os.path.exists(p):
return p
if error_on_missing:
raise Exception("*** ERROR: '%s' not found on:\n %s\n" % (prog_filename, "\n ".join(paths_tried)))
else:
return None
然后你可以做类似的事情:
grep_path = find_program('ack_grep', False)
if grep_path is None:
# default to standard system grep
grep_path = 'grep'