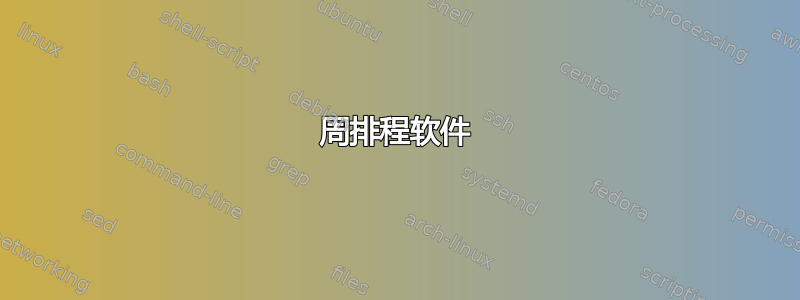
我正在寻找一款可以设置 52 周任务计划并在任务到期时收到提醒的软件。任务类型包括特定类型的服务器维护或特定服务器组的 Windows 更新。
举个例子:
Fred 需要安排时间每三个月更新一次 IIS Web 服务器池和 Apache Web 服务器池。他创建了一个条目并将其设置为每三个月重复一次。在预定事件发生前两周,他收到了有关该事件的电子邮件提醒。一周后,安全团队收到了一封电子邮件提醒,要求更新服务器。
任何帮助都将不胜感激。不确定这对超级用户来说是否是一个好问题。
答案1
Outlook 有一个非常方便的任务功能可供您使用。
答案2
这是我最终使用的方法...虽然不理想,但效果还不错。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Microsoft.Exchange.WebServices.Data;
using Microsoft.Exchange.WebServices.Autodiscover;
using System.Net;
namespace program
{
class Program
{
static void Main(string[] args)
{
// Connect to Office 365 Exchange
ExchangeService service = new ExchangeService();
NetworkCredential ncNetworkCredential = new NetworkCredential("user@example", "password", "");
service.Credentials = ncNetworkCredential;
service.AutodiscoverUrl("user@example", delegate { return true; });
// Connect to shared mailbox and get the folder id of the calendar
Mailbox mb = new Mailbox("[email protected]");
FolderId fid = new FolderId(WellKnownFolderName.Calendar, mb);
// Create a string to hold all of the email content...
String emailBody = "";
emailBody += "<p style=\"font-size:10pt; color: #AAA;text-align:center;\">Please do not reply to this email.</p>";
// Create a weekly calendar view...
CalendarView view = new CalendarView(DateTime.Now.AddDays(1).AddHours(-7),DateTime.Now.AddDays(8));
FindItemsResults<Appointment> results = service.FindAppointments(fid, view);
// Look through the appointments of the week.
for (int i = 0; i < results.Items.Count; i++)
{
try
{
// Add text to the email body.
emailBody += "<p>";
emailBody += "Task: " + results.Items[i].Subject.ToString() + "<br />";
emailBody += "Resource: " + results.Items[i].Location.ToString() + "<br />";
emailBody += "Date: " + results.Items[i].Start.ToShortDateString() + " " + results.Items[i].Start.ToShortTimeString() + "<br />";
emailBody += "</p>";
}
catch
{
// Add text to the email body.
emailBody += "<p>";
emailBody += "Task: " + results.Items[i].Subject.ToString() + "<br />";
//emailBody += "Resource: " + results.Items[i].Location.ToString() + "<br />";
emailBody += "Date: " + results.Items[i].Start.ToShortDateString() + " " + results.Items[i].Start.ToShortTimeString() + "<br />";
emailBody += "</p>";
}
}
// Line referencing the calendar.
emailBody += "<p style=\"color: red;text-align:center;\">For more information on tasks check the \"calendar\"</p>";
// Create a new email message.
EmailMessage email = new EmailMessage(service);
email.ToRecipients.Add("[email protected]");
email.Subject = "Scheduled Tasks (" + DateTime.Now.ToShortDateString() + ")";
email.Body = emailBody;
try
{
email.Send();
}
catch (Exception e)
{
Console.WriteLine("Error: " + e);
}
}
}
}