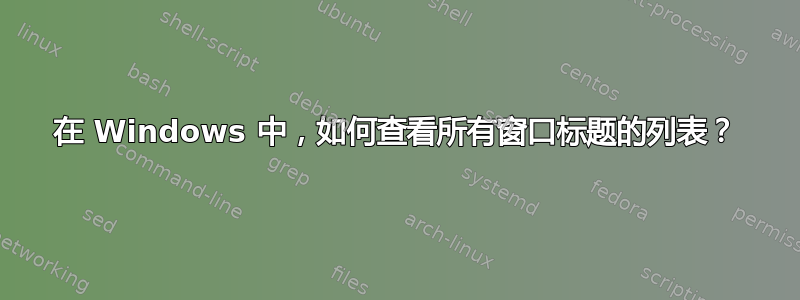
在 Windows 中,如何查看所有打开的 Windows 的所有标题列表。
(最好使用一些像句柄一样的唯一标识符)
答案1
使用枚举窗口()Windows API 函数。它将为屏幕上的所有顶层窗口调用传递给它的应用程序定义的回调函数参数,并将每个窗口的句柄传递给它,直到回调返回FALSE
。
下面是我编写的一个简单的 Python 2.x 控制台程序,它使用该函数(以及其他一些函数来确定哪些窗口可能在桌面上实际可见 - 枚举的许多“顶级”窗口是EnumWindows()
不可见的 - 来完成你想要的。它利用了win32gui
pywin32 的扩展包来访问 Windows API。这也可以在更低、更直接的级别上使用内置模块来实现ctypes
,但在我看来,PyWin32 是一种更高级、更方便的方法。
您可以将输出重定向到文本文件,或者可以修改程序以使用几种不同的可用 GUI 模块在窗口或对话框中显示列表,包括名为的 tk/tcl 接口模块Tkinter
,它是该语言的标准配置。
import sys
import win32gui
def callback(hwnd, strings):
if win32gui.IsWindowVisible(hwnd):
window_title = win32gui.GetWindowText(hwnd)
left, top, right, bottom = win32gui.GetWindowRect(hwnd)
if window_title and right-left and bottom-top:
strings.append('0x{:08x}: "{}"'.format(hwnd, window_title))
return True
def main():
win_list = [] # list of strings containing win handles and window titles
win32gui.EnumWindows(callback, win_list) # populate list
for window in win_list: # print results
print window
sys.exit(0)
if __name__ == '__main__':
main()
答案2
我从以下位置获取了 PowerShell 脚本使用 PowerShell 函数和回调函数枚举所有 Windows 窗口并稍加修改。
它打印窗口名称和句柄。
<#
.Synopsis
Enumerieren der vorhandenen Fenster
#>
$TypeDef = @"
using System;
using System.Text;
using System.Collections.Generic;
using System.Runtime.InteropServices;
namespace Api
{
public class WinStruct
{
public string WinTitle {get; set; }
public int WinHwnd { get; set; }
}
public class ApiDef
{
private delegate bool CallBackPtr(int hwnd, int lParam);
private static CallBackPtr callBackPtr = Callback;
private static List<WinStruct> _WinStructList = new List<WinStruct>();
[DllImport("User32.dll")]
[return: MarshalAs(UnmanagedType.Bool)]
private static extern bool EnumWindows(CallBackPtr lpEnumFunc, IntPtr lParam);
[DllImport("user32.dll", CharSet = CharSet.Auto, SetLastError = true)]
static extern int GetWindowText(IntPtr hWnd, StringBuilder lpString, int nMaxCount);
private static bool Callback(int hWnd, int lparam)
{
StringBuilder sb = new StringBuilder(256);
int res = GetWindowText((IntPtr)hWnd, sb, 256);
_WinStructList.Add(new WinStruct { WinHwnd = hWnd, WinTitle = sb.ToString() });
return true;
}
public static List<WinStruct> GetWindows()
{
_WinStructList = new List<WinStruct>();
EnumWindows(callBackPtr, IntPtr.Zero);
return _WinStructList;
}
}
}
"@
Add-Type -TypeDefinition $TypeDef -Language CSharpVersion3
[Api.Apidef]::GetWindows() | Where-Object { $_.WinTitle -ne "" } | Sort-Object -Property WinTitle | Select-Object WinTitle,@{Name="Handle"; Expression={"{0:X0}" -f $_.WinHwnd}}