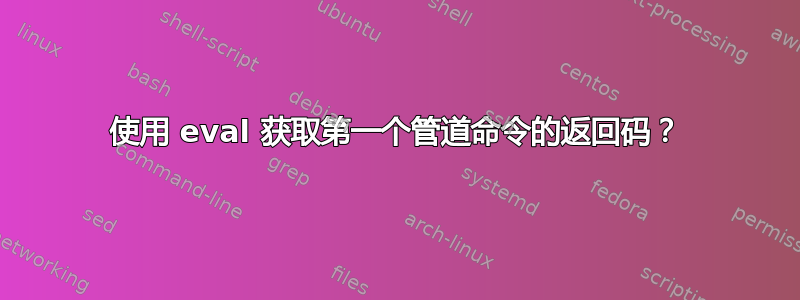
我希望执行不同的命令并随后检查返回代码,然后再继续脚本中的后续步骤。同时我还希望使用 tee 命令将执行命令的输出记录到文件中。例子:
#set non-existing folder
local_path="~/njn"
log_path_file="test.log"
cmd="ls -l ${local_path} | tee -a ${log_path_file}";
eval ${cmd}
returncode=$?
echo "execution result: ${returncode}" | tee -a ${log_path_file};
if [ ${returncode} -eq 0 ]; then
echo "success" | tee -a ${log_path_file}
else
echo "not success" | tee -a ${log_path_file}
fi
返回码是 0,它应该 > 0
我希望 returncode 变量具有所执行命令的实际返回(在本例中为 ls -l 命令)。
我看到有一个解决方案,使用文件将命令的输出写入其中,然后从中读取返回代码(这里),但我正在寻找更优雅的解决方案。
答案1
ls -l
在这种特殊情况下,直接执行退出状态并采取行动会更容易:
if ls -l "$local_path"; then
echo 'success'
else
printf 'failure (code %d)\n' "$?"
fi | tee -a "$log_path_file"
在bash
shell 中,您还可以研究数组中的值PIPESTATUS
:
$ false | true | true | false | false
$ printf '%s\n' "${PIPESTATUS[@]}"
1
0
0
1
1
在你的情况下:
ls -l "$local_path" | tee -a "$log_path_file"
ls_status=${PIPESTATUS[0]}
if [ "$ls_status" -eq 0 ]; then
echo 'success'
else
printf 'failure (code %d)\n' "$ls_status"
fi | tee -a "$log_path_file"
答案2
您可以使用一个函数,该函数将命令作为参数并将tee
输出(stdout 和 stderr)发送到日志文件。
local_path="~/njn"
log_path_file="test.log"
function log_cmd ()
{
{
"$@"
returncode=$?
if [ "$returncode" -eq 0 ]; then
echo "[successfully executed \"$@\"]"
else
echo "[failed to execute \"$@\", exit code: ${returncode}]"
fi
return $returncode
} 2>&1 | tee -a "$log_path_file"
# return exit code of first command in pipeline
return ${PIPESTATUS[0]}
}
log_cmd ls -l "$local_path"
log_cmd echo "hello world"
答案3
经过一些额外的测试,我发现这个代码开关包装得很好,并返回执行的命令返回代码。 @Freddy 发布的代码已接近完成。返回码在函数内部导出,但不导出到函数外部。
shopt -s lastpipe 的使用取自此页面:Bash FAQ 条目#24:“我在循环中设置变量。为什么它们在循环终止后突然消失?或者,为什么我不能通过管道读取数据?”
这是最终的工作解决方案:
#!/bin/bash
log_path_file="./logs/test.log"
exe_cmd()
{
echo "`date +%Y-%m-%d---%r` [Info]: Command to execute: $@" | tee -a ${log_path_file};
echo "" | tee -a ${log_path_file};
echo "" | tee -a ${log_path_file};
set +m
shopt -s lastpipe
cmdResult=0
{
"$@"
returncode=$?
# save result code
cmdResult=${returncode}
if [ "$returncode" -eq 0 ]; then
echo "`date +%Y-%m-%d---%r` [Info]: successfully executed \"$@\""
else
echo "`date +%Y-%m-%d---%r` [Info]: failed to execute \"$@\", exit code: ${returncode}"
fi
} 2>&1 | tee -a "$log_path_file"
echo "`date +%Y-%m-%d---%r` [Info]: cmdResult result ${#cmdResult[@]}"
return ${#cmdResult[@]};
}
cmd="scp some_user@$some_host:some_path/* a_local_path/sub_path";
exe_cmd ${cmd}
returncode=$?
echo "`date +%Y-%m-%d---%r` [Info]: scp execution result: ${returncode}" | tee -a ${log_path_file};