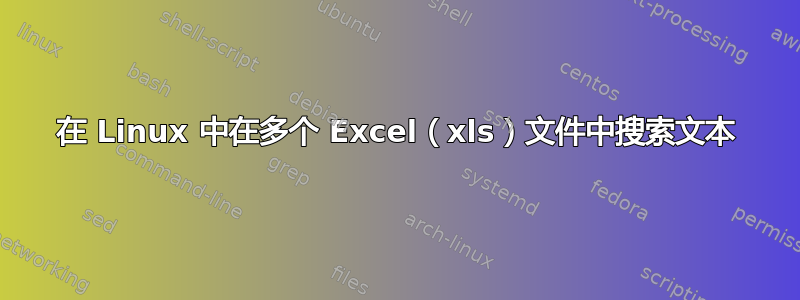
我正在使用 Ubuntu Linux,但找不到在多个 excel ( xls
) 文件中查找文本的方法。我希望通过命令行执行此操作,但也欢迎使用其他替代方法。
答案1
我使用 ssconvert 工具(来自 Gnumeric)制作了一个。您需要先安装 Gnumeric:
sudo apt-get install gnumeric
您可以将内容保存为脚本并调用:
./script-find-in-xls.sh text
脚本的内容。
#!/bin/sh
# $1 - text to find
if [ -z "$1" ]
then
echo 'Please, the text is mandatory'
exit 1
fi
rm -rf /tmp/xls-csv/
mkdir /tmp/xls-csv/
cd /tmp/xls-csv/
cp /location/of/excel-files/*.xls /tmp/xls-csv/
for f in *.xls; do
ssconvert -S --import-encoding=ISO8859-1 ./"$f" ./"${f%.xls}.csv"
done
cat *.csv.* > all-xls-content.txt
rm *.csv.*
if cat all-xls-content.txt | egrep --color $1; then
echo 'found'
else
echo 'not found'
fi
该脚本将所有 xls 文件转换为 csv 文件,将 csv 文件合并到一个文件中,然后使用它egrep
来查找文本。
该代码并不完美,但可以完成工作。
答案2
我也遇到了同样的问题。运行此脚本:
python search.py /home/user/directory string
您可以在这里找到我的解决方案:
#!/usr/bin/python
import os, sys
import xlrd
def find(path, word):
l = []
d = os.listdir(path)
for file in d:
filename = str(path) +'/' + str(file)
print ('Finding in %s' %file)
if filename.endswith('.xlsx'):
wb = xlrd.open_workbook(filename)
ws = wb.sheet_by_index(0)
for i, row in enumerate(range(ws.nrows)):
for j, col in enumerate(range(ws.ncols)):
if str(word) in str(ws.cell_value(i, j)):
l.append((file,row,col))
if l:
print ('Word %s found %d times in:' %(word,len(l)))
for fn, row, col in l:
print ('File: %s, row: %s ,column: %s' %(fn,row,col))
else:
print ('Word %s not found' %word)
if __name__ == "__main__":
try:
find (sys.argv[1],sys.argv[2])
except IndexError:
print('\tExecute: python searchpy <path> <word>')
print('\tEg: python searchpy /home/user/files/ Fox')
你可以从 Github 中提取:searb-in-xlsx
答案3
如果您同意安装索引应用程序,那么这可能是一个答案。
我个人使用回忆,设置和安装都很简单。其默认界面是 GUI,但也可以在 CLI 中使用它:
recoll -q <search terms>
您可以设置一个计划任务来更新其索引,或者手动启动它recollindex
(在这两种情况下,您都可以将索引限制在您选择的路径上)。
答案4
修改 Andre Araujo 的答案以使用 openpyxl,因为 xlrd 无法再打开 xslx 文件:
#!/usr/bin/python
import os, sys
import openpyxl
def find(path, word):
l = []
d = os.listdir(path)
for file in d:
filename = str(path) + "/" + str(file)
print("Finding %s in %s" % (word, file))
if filename.endswith(".xlsx"):
wb = openpyxl.load_workbook(filename=filename)
ws = wb.active
for row in ws.rows:
for cell in row:
if cell.value and str(word) in cell.value:
l.append((file, cell))
if l:
print("Word %s found %d times in:" % (word, len(l)))
for fn, cell in l:
print("File: %s, row: %s ,column: %s" % (fn, cell.row, cell.column))
else:
print("Word %s not found" % word)
if __name__ == "__main__":
try:
find(sys.argv[1], sys.argv[2])
except IndexError:
print("\tExecute: python searchpy <path> <word>")
print("\tEg: python searchpy /home/user/files/ Fox")