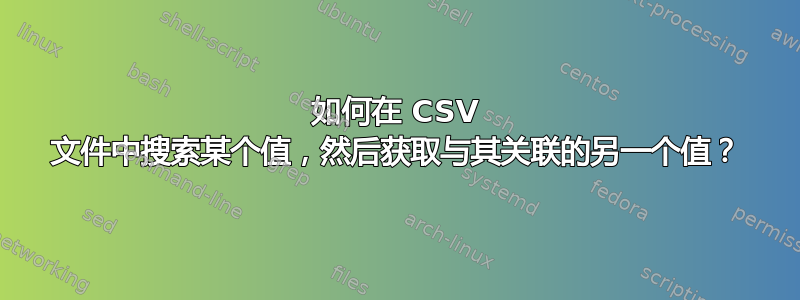
我的文件.csv
445,aLabel
446,anotherLabel
447,aThirdLabel
在 bash 脚本中,我想在 myfile.csv 中搜索“anotherLabel”是否存在(以不区分大小写的方式)并获取值 446。此外,如果“anotherLabel”不存在,我想添加它到最后,将前一行的标记加 1。
我从以下声明开始if
:
if grep -Fiq anotherLabel myfile.csv; then
#get the value of field 1 (446 in this example) and store it in a variable
else
#increment the last value of field 1 present in the file and store it in a variable
#and append "448,anotherLabel" to myfile.csv
fi
我不确定使用 grep 验证标签是否在文件中是否是解决此问题的最佳方法,或者是否有使用 sed 或 awk 的更简单的方法。
答案1
试试这个:
awk -F',' '{ if ($2 == "anotherLabel") { print $1 } }' myfile.csv
答案2
使用grep
和tail
:
search="anotherLabel"
file=myfile.csv
if value=$(grep -Pio -m1 "^[0-9]+(?=,$search$)" "$file"); then
echo "do something with $value"
elif lastvalue=$(tail -n1 "$file" | grep -o '^[0-9]\+'); then
# append lastvalue + 1 and search string
echo "$((++lastvalue)),$search" >> "$file"
else
# handle error
echo "error. no integer value in last line of \"$file\" found." >&2
fi
第一个grep
使用以下选项:
-P
启用 Perl 兼容的正则表达式 (PCRE) 以使用正向前瞻(见下文)。-i
忽略模式中的大小写-o
只打印该行的匹配部分-m1
第一场比赛后停止
第一个正则表达式^[0-9]+(?=,$search$)
使用正向先行(?=pattern)
来匹配后跟的数字,
和不带逗号的搜索字符串,并且搜索字符串是匹配本身的一部分。与选项组合时,-o
仅打印匹配的部分(数字)。
答案3
如果你想在纯 bash 中执行此操作:
file="myfile.csv"
seek="anotherLabel"
while IFS=, read id label; do
if [[ $label == "$seek" ]]; then
myid=$id
break
fi
lastid=$id
done < "$file"
if [[ -z $myid ]]; then
myid=$((lastid + 1))
echo "$myid,$seek" >> "$file"
fi
echo "$seek id is: $myid"
答案4
以下 awk 代码可以满足您的要求:
#!/bin/bash
filetosearch=myfile.csv
searchString=${1:-anotherLabel}
awk -F',' -v pat="$searchString" '
BEGIN{patl=tolower(pat);flag=0};
{prev=$1}(tolower($0)==patl){flag=1;exit}
END{
if(flag){
print prev
}else{
printf("%s%s%s\n", prev+1,FS,pat) >> ARGV[1] # use ARGIND in gawk.
print prev+1
}
}' "${filetosearch}"
"${searchString}"
搜索与整行完全匹配的字符串(更改tolower($0)==patl
为tolower($0)~patl
更宽松地匹配)并报告在哪个索引处找到它。如果字符串不匹配,则将其添加(附加)到所使用的文件中,其索引比文件的最后一个索引大 1。
例子:
$ ./script aLabel
445
$ ./script anotherLabel
446
$ ./script MissingLabel
450
$ cat myfile.csv
445,aLabel
446,anotherLabel
447,aThirdLabel
448,dhdhdhdhdhd
449,anotherLabel4646
450,MissingLabel