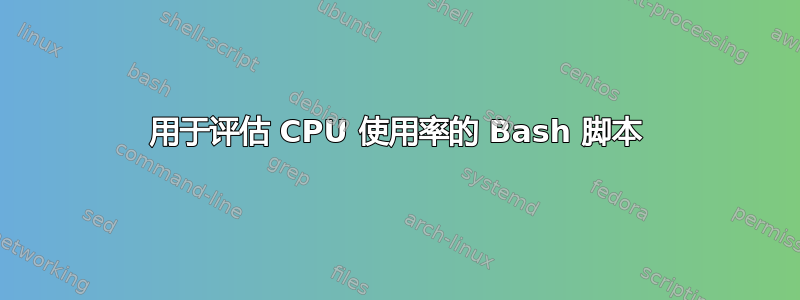
我正在尝试编写一个 BASH 脚本,它将评估并在终端中显示所有核心及其当前负载的列表。我正在使用 的输出/proc/stat
。例如:
cat /proc/stat
user nice system idle iowait irq softirq steal guest guest_nice
cpu 4705 356 584 3699 23 23 0 0 0 0
user
并通过对、nice
、system
、irq
、进行求和来评估所使用的 CPU 时间,softirq
并通过对、steal
进行求和来评估 CPU 空闲时间。然后,我将已用 CPU 时间 + CPU 空闲时间相加以获得总 CPU 时间,并将 CPU 使用时间除以总 CPU 时间。idle
iowait
这种方法的问题在于,这是自系统上次启动以来的平均 CPU 使用率。为了获得当前的使用情况,我需要检查两次/proc/stat
并使用两次检查之间的总 CPU 时间和已用 CPU 时间之间的差异,然后将结果除以它们之间的时间差(以秒为单位)。为此,我使用while
无限循环和睡眠命令。我想要以下格式的输出:
CPU: 10%
CPU0: 15%
CPU1: 5%
CPU2: 7%
CPU3: 13%
我希望所有核心的总 CPU 使用率和每个核心的 CPU 使用率在每次睡眠后自动更新。到目前为止,这是我的代码:
#!/bin/bash
PREV_CPU_USE=0
PREV_CPU_IDLE=0
PREV_EPOCH_TIME=0
# Setting the delimiter
IFS=$'\n'
while true; do
# Getting the total CPU usage
CPU_USAGE=$(head -n 1 /proc/stat)
# Getting the Linux Epoch time in seconds
EPOCH_TIME=$(date +%s)
# Splitting the /proc/stat output
IFS=" " read -ra USAGE_ARRAY <<< "$CPU_USAGE"
# Calculating the used CPU time, CPU idle time and CPU total time
CPU_USE=$((USAGE_ARRAY[1] + USAGE_ARRAY[2] + USAGE_ARRAY[3] + USAGE_ARRAY[6] + USAGE_ARRAY[7] + USAGE_ARRAY[8] ))
CPU_IDLE=$((USAGE_ARRAY[4] + USAGE_ARRAY[5]))
# Calculating the differences
DIFF_USE=$((CPU_USE - PREV_CPU_USE))
DIFF_IDLE=$((CPU_IDLE - PREV_CPU_IDLE))
DIFF_TOTAL=$((DIFF_USE + DIFF_IDLE))
DIFF_TIME=$((EPOCH_TIME - PREV_EPOCH_TIME))
#Printing the line and ommiting the trailing new line and using carrier trailer to go to the beginning of the line
echo -en "${USAGE_ARRAY[0]} Usage: $((DIFF_USE*100/(DIFF_TOTAL*DIFF_TIME)))% \\r\\n"
echo -en "${USAGE_ARRAY[0]} Idle: $((DIFF_IDLE*100/(DIFF_TOTAL*DIFF_TIME)))% \\r"
# Assigning the old values to the PREV_* values
PREV_CPU_USE=$CPU_USE
PREV_CPU_IDLE=$CPU_IDLE
PREV_EPOCH_TIME=$EPOCH_TIME
# Sleep for one second
sleep 1
done
在这里,我简化了脚本,实际上仅在两行不同的行上打印当前的 CPU 使用率和空闲 CPU 时间,但即使cpu Idle
保留在一行上,也会cpu Usage
添加新行,例如:
cpu Usage: 0%
cpu Usage: 0%
cpu Usage: 0%
cpu Idle: 99%
是否可以选择cpu Usage
在脚本的整个持续时间内仅在一行上?
答案1
我认为您希望cpu Usage
每次都将字符串显示在同一行上,并立即跟随该cpu Idle
行。要实现此目的,您可以使用tput
( el
)clr_eol
终端功能删除行,并使用cuu
( parm_up_cursor
) 向上移动 n 行。您可以在 中阅读有关终端功能的信息man terminfo
。你的脚本看起来像这样:
#!/bin/bash
PREV_CPU_USE=0
PREV_CPU_IDLE=0
PREV_EPOCH_TIME=0
# Setting the delimiter
IFS=$'\n'
counter=0
while true; do
# Getting the total CPU usage
CPU_USAGE=$(head -n 1 /proc/stat)
# Getting the Linux Epoch time in seconds
EPOCH_TIME=$(date +%s)
# Splitting the /proc/stat output
IFS=" " read -ra USAGE_ARRAY <<< "$CPU_USAGE"
# Calculating the used CPU time, CPU idle time and CPU total time
CPU_USE=$((USAGE_ARRAY[1] + USAGE_ARRAY[2] + USAGE_ARRAY[3] + USAGE_ARRAY[6] + USAGE_ARRAY[7] + USAGE_ARRAY[8] ))
CPU_IDLE=$((USAGE_ARRAY[4] + USAGE_ARRAY[5]))
# Calculating the differences
DIFF_USE=$((CPU_USE - PREV_CPU_USE))
DIFF_IDLE=$((CPU_IDLE - PREV_CPU_IDLE))
DIFF_TOTAL=$((DIFF_USE + DIFF_IDLE))
DIFF_TIME=$((EPOCH_TIME - PREV_EPOCH_TIME))
printf "\r%s%s Usage: %d (counter = %d)\n" "$(tput el)" "${USAGE_ARRAY[0]}" "$((DIFF_USE*100/(DIFF_TOTAL*DIFF_TIME)))" "$counter"
printf "\r%s%s Idle: %d (counter = %d)" "$(tput el)" "${USAGE_ARRAY[0]}" "$((DIFF_IDLE*100/(DIFF_TOTAL*DIFF_TIME)))" "$counter"
counter=$((counter + 1))
tput cuu 1
# Assigning the old values to the PREV_* values
PREV_CPU_USE=$CPU_USE
PREV_CPU_IDLE=$CPU_IDLE
PREV_EPOCH_TIME=$EPOCH_TIME
# Sleep for one second
sleep 1
done
我添加了一个额外的counter
变量用于调试目的。每次打印后它都会递增,以通知用户旧行已被屏幕上的新行替换。
echo
我还用替换了您的呼叫,printf
因为它更便携。