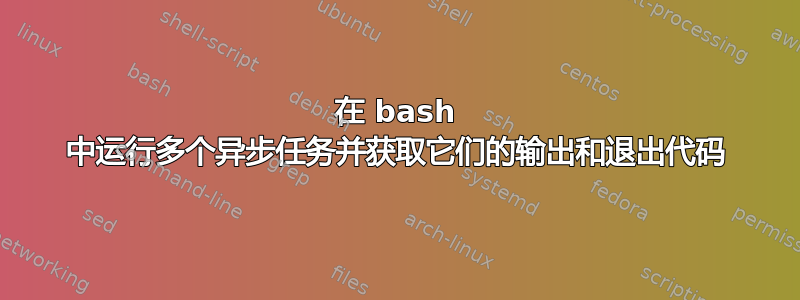
我必须异步运行一堆命令,一旦其中一个命令完成,我就需要根据其退出代码和输出执行操作。请注意,我无法预测这些任务在我的实际用例中将运行多长时间。
为了解决这个问题,我最终得到了以下算法:
For each task to be run:
Run the task asynchronously;
Append the task to the list of running tasks.
End For.
While there still are tasks in the list of running tasks:
For each task in the list of running tasks:
If the task has ended:
Retrieve the task's exit code and output;
Remove the task from the list of running tasks.
End If.
End For
End While.
这给了我以下 bash 脚本:
1 #!/bin/bash
2
3 # bg.sh
4
5 # Executing commands asynchronously, retrieving their exit codes and outputs upon completion.
6
7 asynch_cmds=
8
9 echo -e "Asynchronous commands:\nPID FD"
10
11 for i in {1..10}; do
12 exec {fd}< <(sleep $(( i * 2 )) && echo $RANDOM && exit $i) # Dummy asynchronous task, standard output's stream is redirected to the current shell
13 asynch_cmds+="$!:$fd " # Append the task's PID and FD to the list of running tasks
14
15 echo "$! $fd"
16 done
17
18 echo -e "\nExit codes and outputs:\nPID FD EXIT OUTPUT"
19
20 while [[ ${#asynch_cmds} -gt 0 ]]; do # While the list of running tasks isn't empty
21
22 for asynch_cmd in $asynch_cmds; do # For each to in thhe list
23
24 pid=${asynch_cmd%:*} # Task's PID
25 fd=${asynch_cmd#*:} # Task's FD
26
27 if ! kill -0 $pid 2>/dev/null; then # If the task ended
28
29 wait $pid # Retrieving the task's exit code
30 echo -n "$pid $fd $? "
31
32 echo "$(cat <&$fd)" # Retrieving the task's output
33
34 asynch_cmds=${asynch_cmds/$asynch_cmd /} # Removing the task from the list
35 fi
36 done
37 done
输出告诉我wait
尝试检索每个任务的退出代码失败,预计最后一个运行:
Asynchronous commands:
PID FD
4348 10
4349 11
4351 12
4353 13
4355 14
4357 15
4359 16
4361 17
4363 18
4365 19
Exit codes and outputs:
PID FD EXIT OUTPUT
./bg.sh: line 29: wait: pid 4348 is not a child of this shell
4348 10 127 16010
./bg.sh: line 29: wait: pid 4349 is not a child of this shell
4349 11 127 8341
./bg.sh: line 29: wait: pid 4351 is not a child of this shell
4351 12 127 13814
./bg.sh: line 29: wait: pid 4353 is not a child of this shell
4353 13 127 3775
./bg.sh: line 29: wait: pid 4355 is not a child of this shell
4355 14 127 2309
./bg.sh: line 29: wait: pid 4357 is not a child of this shell
4357 15 127 32203
./bg.sh: line 29: wait: pid 4359 is not a child of this shell
4359 16 127 5907
./bg.sh: line 29: wait: pid 4361 is not a child of this shell
4361 17 127 31849
./bg.sh: line 29: wait: pid 4363 is not a child of this shell
4363 18 127 28920
4365 19 10 28810
命令的输出已完美检索,但我不明白此is not a child of this shell
错误来自何处。我肯定做错了什么,因为wait
我能够获取要异步运行的最后一个命令的退出代码。
有人知道这个错误是从哪里来的吗?我对这个问题的解决方案有缺陷吗,还是我误解了 bash 的行为?
答案1
出现此错误信息的原因是,您尝试等待的特定 PID 的进程已经完成了从未作为异步进程运行。exec …
结果为何会$!
被填充我不得而知。您可以使用无法到达的 PID:
$ wait $(($(cat /proc/sys/kernel/pid_max) + 1))
bash: wait: pid 32769 is not a child of this shell