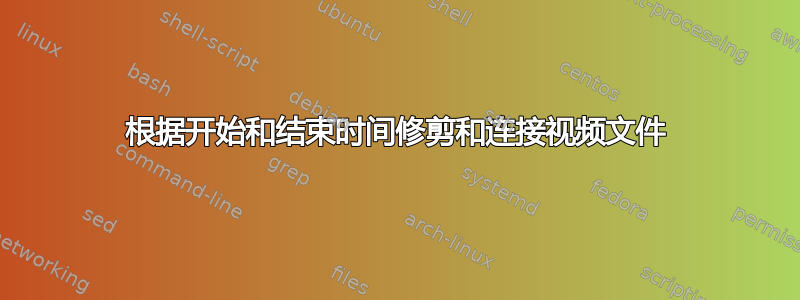
我将有'n'个视频文件,每个文件时长为1分钟(带有时间戳)(比如说我从12.00.00开始录制并在1.00.00停止,然后将生成60个视频文件)基于输入:(开始时间(比如说12.00.59)和结束时间(比如说12.02.39),我需要修剪和合并文件。开始和结束可以在同一个1分钟视频(单个文件中),也可以在不同的文件中
我曾尝试简单地使用 system() 函数来调用 ffmpeg API
#include <iostream>
#include <string.h>
#include <cstddef>
#include <unistd.h>
#include <stdio.h>
#include <stdlib.h>
using namespace std;
int main(int argc,char** argv)
{
if((argc < 3) || (argc >3))
{
cout << "Execute in provided format" << "\n";
cout << "binary $start_time(xx:xx:xx)as xxxxxx $end_time(xx:xx:xx)as xxxxxx (i.e)without ':'" << "\n";
cout << "For Example for 18.30.10 18.35.14 input as 183010 183514" << "\n";
return 0;
}
uint32_t start_time =(uint32_t)atoi(argv[1]);
uint32_t end_time =(uint32_t)atoi(argv[2]);
uint32_t total_time = ((end_time/100) - (start_time/100));
std::string file_path("/home/event_clip/test/vfiles/");
file_path += std::to_string((start_time/100));
file_path += ".mp4";
const char* fname = file_path.c_str();
cout << fname << "\n";
FILE *file = fopen(file_path.c_str(), "r+");
if(file == NULL){
cout << "video file not yet created";
}else{
if(total_time < 1){
cout << "Req video is in single file" << "\n";
cout << "Trim is enough" << "\n";
char buf[64];
snprintf(buf, sizeof(buf), "ffmpeg -t 00:00:%u -i %u.mp4 -ss 00:00:%u trim.mp4 -y",end_time%100,(start_time/100),start_time%100);
system(buf);
}else{
FILE *concat_fp ;
concat_fp = fopen("/home/event_clip/test/vfiles/concat_files.txt","w");
fclose(concat_fp);
concat_fp = fopen("/home/event_clip/test/vfiles/concat_files.txt","a+");
cout << "Required video is in " << total_time+1 << " files" << "\n";
cout << "Trim and merge has to be done" << "\n";
/* Trim start file */
char startbuf[64];
snprintf(startbuf, sizeof(startbuf), "ffmpeg -t 00:01:00 -i %u.mp4 -ss 00:00:%u concatstart.mp4 -y",(start_time/100),start_time%100);
system(startbuf);
fprintf(concat_fp,"file './concatstart.mp4'\n");
/* Trim end file */
char endbuf[64];
snprintf(endbuf, sizeof(endbuf), "ffmpeg -t 00:00:%u -i %u.mp4 -ss 00:00:00 concatend.mp4 -y",end_time%100,(start_time/100));
system(endbuf);
if(total_time > 2){
for(int i = 1; i < (total_time+1); i++){
fprintf(concat_fp,"file './%u.mp4'\n",((start_time/100)+i));
}
}
fprintf(concat_fp,"file './concatend.mp4'\n");
fclose(concat_fp);
char concatbuf[67];
snprintf(concatbuf, sizeof(concatbuf), "ffmpeg -f concat -safe 0 -i concat_files.txt -c copy concat.mp4 -y");
system(concatbuf);
}
}
}
有人能帮助我使用 libav 添加 ffmpeg 'C' API 吗