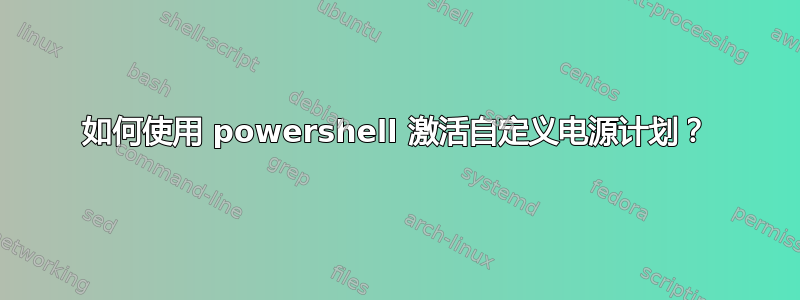
因此,有一种方法可以做到这一点,即使用powercfg /l
。然后复制 GUID 并键入powercfg /s [GUID]
。但我想知道有没有办法在不复制 GUID 的情况下做到这一点。因此,当您运行脚本时,您会立即激活自定义电源计划。我所说的自定义电源计划是终极性能。
答案1
将输出解析powercfg /l
为 PSObjects
$powerSchemes = powercfg /l | ForEach-Object {
if ($_ -match '^Power Scheme GUID:\s*([-0-9a-f]+)\s*\(([^)]+)\)\s*(\*)?') {
[PsCustomObject]@{
GUID = $matches[1]
SchemeName = $matches[2]
Active = $matches[3] -eq '*'
}
}
}
# if you want to view the schemes we've parsed into objects:
# $powerSchemes
接下来,在代码中从自定义名称中选择方案,例如:
$customScheme = $powerSchemes | Where-Object { $_.SchemeName -eq 'MyCustomPowerScheme' }
并使用以下命令将方案设置为活动方案(如果尚未激活)
if (!$customScheme.Active) {
powercfg /s $($customScheme.GUID)
}
正则表达式详细信息:
^ Assert position at the beginning of a line (at beginning of the string or after a line break character)
Power\ Scheme\ GUID: Match the characters “Power Scheme GUID:” literally
\s Match a single character that is a “whitespace character” (spaces, tabs, line breaks, etc.)
* Between zero and unlimited times, as many times as possible, giving back as needed (greedy)
( Match the regular expression below and capture its match into backreference number 1
[-0-9a-f] Match a single character present in the list below
The character “-”
A character in the range between “0” and “9”
A character in the range between “a” and “f”
+ Between one and unlimited times, as many times as possible, giving back as needed (greedy)
)
\s Match a single character that is a “whitespace character” (spaces, tabs, line breaks, etc.)
* Between zero and unlimited times, as many times as possible, giving back as needed (greedy)
\( Match the character “(” literally
( Match the regular expression below and capture its match into backreference number 2
[^)] Match any character that is NOT a “)”
+ Between one and unlimited times, as many times as possible, giving back as needed (greedy)
)
\) Match the character “)” literally
\s Match a single character that is a “whitespace character” (spaces, tabs, line breaks, etc.)
* Between zero and unlimited times, as many times as possible, giving back as needed (greedy)
( Match the regular expression below and capture its match into backreference number 3
\* Match the character “*” literally
)? Between zero and one times, as many times as possible, giving back as needed (greedy)
答案2
为了使用 PowerShell 激活自定义电源计划,您可以编写一个 PowerShell 脚本。在您需要执行的脚本中powercfg /l
,解析 GUID 的输出,然后powercfg /s
使用您之前找到的 GUID 进行调用。
如果你在编写脚本时遇到任何问题,请随时详细说明具体的编程问题https://stackoverflow.com/求助。
答案3
❖您还可以激活您的计划/方案:
$(powercfg.exe /L) -match 'Ultimate'|% {powercfg.exe /S $_.split(" ")[3]}
$(powercfg.exe /L) -match ':'|% {$_}
❖ 结果:
Power Scheme GUID: 381b4222-f694-41f0-9685-ff5bb260df2e (Balanced)
Power Scheme GUID: 8c5e7fda-e8bf-4a96-9a85-a6e23a8c635c (High performance)
Power Scheme GUID: a1841308-3541-4fab-bc81-f71556f20b4a (Power saver) *
Power Scheme GUID: e9a42b02-d5df-448d-aa00-03f14749eb61 (Ultimate Performance)
您可以通过简单地按空格过滤输出字符串来获得它,您的字符串位于包含以下内容的行的第 3 次出现中"You Plan/Scheme Name"
$(powercfg.exe /L) -match 'Ultimate'|% {$_.split(" ")[3]}
❖ 结果:
e9a42b02-d5df-448d-aa00-03f14749eb61
答案4
function Get-PowerScheme {
param (
[string]$SchemeName,
[string]$SchemeGuid,
[string]$SchemesDetails # optional
)
if (($SchemeName) -and ($SchemeGuid)) {
Write-Host "`n Error: only one criteria is permitted for searching a power scheme" -ForegroundColor "Red"
Write-Host " '-SchemeName `"$SchemeName`"' and '-SchemeGuid `"$SchemeGuid`"' arguments can't be input together ...`n" -ForegroundColor "Red"
return $Null
}
$PowerSchemeObjectArray = @()
$SearchByName = $False
if (([string]::IsNullOrWhiteSpace($SchemeGuid)) -and ($SchemeName)) { $SearchByName = $True }
$SearchForAll = $False
if (([string]::IsNullOrWhiteSpace($SchemeName)) -and ([string]::IsNullOrWhiteSpace($SchemeGuid))) { $SearchForAll = $True }
if ([string]::IsNullOrWhiteSpace($SchemesDetails)) { $SchemesDetails = powercfg.exe -LIST | Out-String }
$PowerSchemes = $SchemesDetails -split [Environment]::NewLine
foreach ($PowerScheme in $PowerSchemes) {
if ($PowerScheme -match "GUID") {
# Extract the scheme power name between "(" and ")"
$PowerSchemeName = $PowerScheme -match '\((.*?)\)'
$PowerSchemeName = $matches[1]
# Extract the scheme GUID between ":" and "(", then remove spaces
$PowerSchemeGuid = $PowerScheme -match ':\s*(.*?)\('
$PowerSchemeGuid = $matches[1].Trim()
# Check if current power scheme is active
$IsActive = $False
if ($PowerScheme[-1] -eq "*") {$IsActive = $True}
$PowerSchemeObject = [PSCustomObject]@{Name = $PowerSchemeName; GUID = $PowerSchemeGuid; IsActive = $IsActive}
$AddPowerSchemeObject = $False
if ($SearchForAll) {
$AddPowerSchemeObject = $True
} else {
if ($SearchByName) {
if ($PowerSchemeName -match "$SchemeName") {
$AddPowerSchemeObject = $True
}
} else {
if ($PowerSchemeGuid -match "$SchemeGuid") {
$AddPowerSchemeObject = $True
}
}
}
if ($AddPowerSchemeObject) { $PowerSchemeObjectArray += $PowerSchemeObject }
}
}
return $PowerSchemeObjectArray
}
$MyCustomPowerScheme = Get-PowerScheme -SchemeName "The Name of My Custom Power Plan"
$Result = powercfg.exe /SETACTIVE $MyCustomPowerScheme.GUID