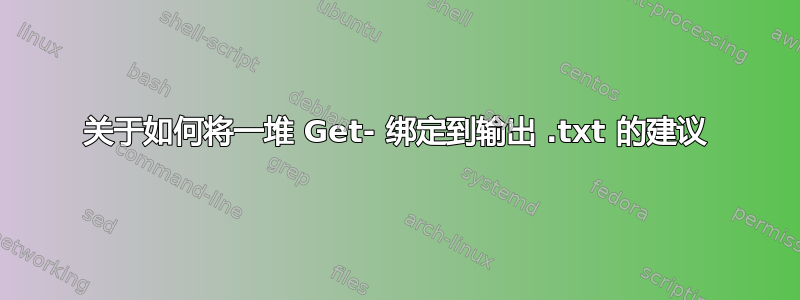
仍在学习 PS 的使用方法,并寻求一些建议。我一直在编写脚本来查找 C 是否小于或等于 20GB,并列出常见位置(如文档和下载)并测量它们的大小。现在一切都已整理好,我想将它检查的每个文件夹放入一个 output.txt 中,但不确定如何操作。我想以某种方式将所有 Get-Childitems 绑定在一起,但不知道从哪里开始。这是我目前得到的结果。
#Check size of C and if its < or = 20GB
$FreeSpace = Get-PSDrive -Name 'c' | Select-Object Free
Write-Host "Free Space On C Left"
$TotalFreeSpace = $FreeSpace.free
$num = $TotalFreeSpace
if ($num -lt 1TB) {
$num = $num / 1GB
$num = "{0:n2}" -f $num
"$num GB"
}
$condition = $TotalFreeSpace
if ( $condition -le 20 )
{
Write-Output "The C Drive's free space is > or = 20GB"
}
#File Location Measures
$UserName = $env:UserName
Write-Host "Desktop Folder Size"
$Desktop = Get-Childitem C:\LOCAL\$UserName\Desktop | Measure-Object -Sum Length
$num = $Desktop.sum
if ($num -lt 1TB) {
$num = $num / 1GB
$num = "{0:n2}" -f $num
"$num GB"
}
Write-Host "My Documents Folder Size"
$Documents = Get-Childitem C:\LOCAL\$UserName\'My Documents' | Measure-Object -Sum Length
$num = $Documents.sum
if ($num -lt 1TB) {
$num = $num / 1GB
$num = "{0:n2}" -f $num
"$num GB"
}
Write-Host "Downloads Folder Size"
$Downloads = Get-Childitem C:\Users\$UserName\Downloads | Measure-Object -Sum Length
$num = $Downloads.sum
if ($num -lt 1TB) {
$num = $num / 1GB
$num = "{0:n2}" -f $num
"$num GB"
}
Write-Host "Picture Folder Size"
$Pictures = Get-Childitem C:\LOCAL\$UserName\'My Documents'\'My Pictures' | Measure-Object -Sum Length
$num = $Pictures.sum
if ($num -lt 1TB) {
$num = $num / 1GB
$num = "{0:n2}" -f $num
"$num GB"
}
Write-Host "Music Folder Size"
$Music = Get-Childitem C:\LOCAL\$UserName\'My Documents'\'My Music' | Measure-Object -Sum Length
$num = $Music.sum
if ($num -lt 1TB) {
$num = $num / 1GB
$num = "{0:n2}" -f $num
"$num GB"
}
Write-Host "Videos Folder Size"
$Videos = Get-Childitem C:\LOCAL\$UserName\'My Documents'\'My Videos' | Measure-Object -Sum Length
$num = $Videos.sum
if ($num -lt 1TB) {
$num = $num / 1GB
$num = "{0:n2}" -f $num
"$num GB"
}
Write-Host "Recycle Bin Size"
($RecycleBin = Get-ChildItem -LiteralPath 'C:\$Recycle.Bin' -File -Force -Recurse -ErrorAction SilentlyContinue |
Measure-Object -Property Length -Sum).Sum
$num = $RecycleBin.sum
if ($num -lt 1TB) {
$num = $num / 1GB
$num = "{0:n2}" -f $num
"$num GB"
}
| Out-File -Path C:\LOCAL\$UserName\Desktop\$UserName'CDriveFullOutput'.txt
#Thanks to Lee_Dailey for Dev help!
答案1
我注意到的第一件事是,你正在计算文件的大小直接地文件夹内有,但子文件夹中没有存储任何内容。您只能在回收站上使用递归。
其次,您在每个步骤中都重复代码来格式化尺寸,因此我建议为其创建一个小辅助函数,以使代码更加清晰。
然后,您对文件夹路径进行硬编码,我认为有更好的选择,最后,如果您的目标是将 7 个格式化的数字输出到一个文件,为什么不从收集的信息中创建一个 CSV 文件,这样您就有标题来告诉您每个数字代表什么。
请尝试以下操作:
function Format-ByteSize {
# helper function to format a given size in bytes
[CmdletBinding()]
param(
[Parameter(Mandatory = $true, ValueFromPipeline = $true)]
[ValidateRange(0, [double]::MaxValue)]
[double]$SizeInBytes
)
$units = "Bytes", "KB", "MB", "GB", "TB", "PB"
$index = 0
while ($SizeInBytes -gt 1024 -and $index -le $units.length) {
$SizeInBytes /= 1024
$index++
}
if ($index) { '{0:N2} {1}' -f $SizeInBytes, $units[$index] }
else { "$SizeInBytes Bytes" }
}
#Check size of C and if its < or = 20GB
$FreeSpace = (Get-PSDrive -Name 'C').Free
if ($FreeSpace -le (20 * 1GB)) {
Write-Warning "The C Drive's free space is less or equal to 20GB"
}
# get the sizes of the folders of interest.
$desktopPath = [Environment]::GetFolderPath("Desktop")
$DesktopSize = (Get-Childitem -Path $desktopPath -File -Recurse | Measure-Object -Sum Length).Sum
# seeing that the 'My Pictures', 'My Music' and 'My Videos' folders are INSIDE the 'My Documents' folder, you may
# want to think about adding the -Recurse switch to this path or not..
$DocumentSize = (Get-Childitem -Path ([Environment]::GetFolderPath("MyDocuments")) -File | Measure-Object -Sum Length).Sum
# If you've recursed the 'My Documents' folder, the below sizes will be included there
$PicturesSize = (Get-Childitem -Path ([Environment]::GetFolderPath("MyPictures")) -File -Recurse | Measure-Object -Sum Length).Sum
$MusicSize = (Get-Childitem -Path ([Environment]::GetFolderPath("MyMusic")) -File -Recurse | Measure-Object -Sum Length).Sum
$VideoSize = (Get-Childitem -Path ([Environment]::GetFolderPath("MyVideos")) -File -Recurse | Measure-Object -Sum Length).Sum
# the Downloads folder path is more tricky to find
$downloadsPath = (New-Object -ComObject Shell.Application).NameSpace('shell:Downloads').Self.Path
$DownloadSize = (Get-Childitem -Path $downloadsPath -File -Recurse | Measure-Object -Sum Length).Sum
# finally the recycle bin on the C drive
$RecycleBin = (Get-ChildItem -LiteralPath 'C:\$Recycle.Bin' -File -Force -Recurse -ErrorAction SilentlyContinue |
Measure-Object -Property Length -Sum).Sum
# now put all this info in an **object** so you can save as CSV file with headers
$result = [PsCustomObject]@{
FreeSpace = Format-ByteSize $FreeSpace
Desktop = Format-ByteSize $DesktopSize
MyDocuments = Format-ByteSize $DocumentSize
MyPictures = Format-ByteSize $PicturesSize
MyVideos = Format-ByteSize $MusicSize
Downloads = Format-ByteSize $DownloadSize
RecycleBin = Format-ByteSize $RecycleBin
}
# output to console screen
$result
#output to structured CSV file you can open in Excel
$result | Export-Csv -Path (Join-Path -Path $desktopPath -ChildPath 'CDriveFullOutput.csv') -NoTypeInformation -UseCulture
在屏幕上,这将输出如下内容:
FreeSpace : 119,49 GB
Desktop : 12,23 KB
MyDocuments : 26,99 MB
MyPictures : 1,88 GB
MyVideos : 119,90 GB
Downloads : 573,88 MB
RecycleBin : 537 Bytes
答案2
再次感谢大家,终于搞清楚了数组以及该助手的工作原理,真是太好了。必须重写一堆旧的硬件测量方法,哈哈
function Format-ByteSize {
# helper function to format a given size in bytes
[CmdletBinding()]
param(
[Parameter(Mandatory = $true, ValueFromPipeline = $true)]
[ValidateRange(0, [double]::MaxValue)]
[double]$SizeInBytes
)
$units = "Bytes", "KB", "MB", "GB", "TB", "PB"
$index = 0
while ($SizeInBytes -gt 1024 -and $index -le $units.length) {
$SizeInBytes /= 1024
$index++
}
if ($index) { '{0:N2} {1}' -f $SizeInBytes, $units[$index] }
else { "$SizeInBytes Bytes" }
}
$PCName = $env:computername
$UserName = $env:UserName
$FreeSpace = (Get-PSDrive -Name 'C').Free
if ($FreeSpace -le (20 * 1GB)) {
$Desktop = (Get-Childitem C:\LOCAL\$UserName\Desktop -File -Force -Recurse | Measure-Object -Sum Length).Sum
$Documents = (Get-ChildItem -Path C:\LOCAL\$UserName\'My Documents' -File -Force -Recurse | Measure-Object -Sum Length).Sum
$Downloads = (Get-Childitem C:\Users\$UserName\Downloads -File -Force -Recurse | Measure-Object -Sum Length).Sum
$Pictures = (Get-Childitem C:\LOCAL\$UserName\'My Documents'\'My Pictures' -File -Force -Recurse | Measure-Object -Sum Length).Sum
$Music = (Get-Childitem C:\LOCAL\$UserName\'My Documents'\'My Music' -File -Force -Recurse | Measure-Object -Sum Length).Sum
$Videos = (Get-Childitem C:\LOCAL\$UserName\'My Documents'\'My Videos' -File -Force -Recurse | Measure-Object -Sum Length).Sum
$RecycleBin = (Get-ChildItem -LiteralPath 'C:\$Recycle.Bin' -File -Force -Recurse -ErrorAction SilentlyContinue | Measure-Object -Sum Length).Sum
$Aray = [PsCustomObject]@{
FreeSpace = Format-ByteSize $FreeSpace
Desktop = Format-ByteSize $Desktop
Documents = Format-ByteSize $Documents
Downloads = Format-ByteSize $Downloads
Pictures = Format-ByteSize $Pictures
Music = Format-ByteSize $Music
Videos = Format-ByteSize $Videos
RecycleBin = Format-ByteSize $RecycleBin
}
$Aray | Export-Csv -Path (Join-Path -Path "\\HAWA-COL04\Support_Tools\Scripts\PS\Local Scripts\FullC\ScriptOutput" -ChildPath $PCName-$UserName'-FullCDrive'.csv)
}
感谢 Lee_Dailey 和 Theo 的开发帮助!