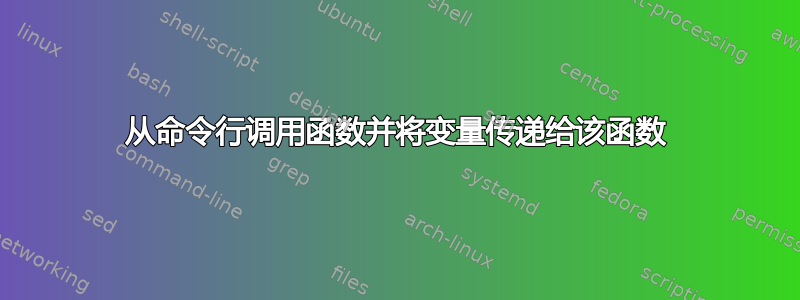
我是 Bash 脚本的新手。我想创建一个脚本,在其中使用变量执行特定函数。
例如
#!/bin/bash
fun1(){
echo "Hello $name"
}
fun2(){
echo "Bye $name"
}
我怎样才能执行它bash myscript.sh --fun1 --name xyz
答案1
不需要命令行解析工具,您可以自己解析:这并不复杂,您将学到很多东西。
命令的参数是 $1、$2、...$# 是参数的数量。shift
移动所有参数:$1 的内容被删除,然后将 $2 放入 $1 中,将 $3 放入 $2 中,等等...
#!/bin/bash
fun1(){
echo "Hello $name"
}
fun2(){
echo "Bye $name"
}
while [ $# -gt 0 ] ; do
case $1 in
'--fun1') fun2exec="fun1" ;;
'--fun2') fun2exec="fun2" ;;
'--name') name="$2" ; shift ;;
*) echo "argument error"; exit 1 ;;
esac
shift
done
if [ -z "$fun2exec" ] ; then
echo "unspecified function"
exit 2
fi
if [ -z "$name" ] ; then
echo "unspecified name"
exit 3
fi
$fun2exec
exit 0
答案2
首先,你需要将长选项转换为短选项如何在 bash-getopts 内置函数中使用长选项。然后评估调用函数和传递变量的简短选项:
#!/bin/bash
fun1(){
echo "Hello $name"
}
fun2(){
echo "Bye $name"
}
for arg in "$@"; do
shift
case "$arg" in
'--fun1') set -- "$@" '-f' ;;
'--fun2') set -- "$@" '-g' ;;
'--name') set -- "$@" '-n' ;;
*) set -- "$@" "$arg" ;;
esac
done
exec_fun1=false
exec_fun2=false
while getopts "n:fg" opt ; do
case "$opt" in
n) name=${OPTARG} ;;
f) exec_fun1=true ;;
g) exec_fun2=true ;;
esac
done
if $exec_fun1; then fun1 $name ; fi
if $exec_fun2; then fun2 $name ; fi
如果你的脚本中有很多函数,那么这种方法就不太方便了。那么让它可调用会更容易,如下所示
bash myscript.sh --fun fun_name --name xyz