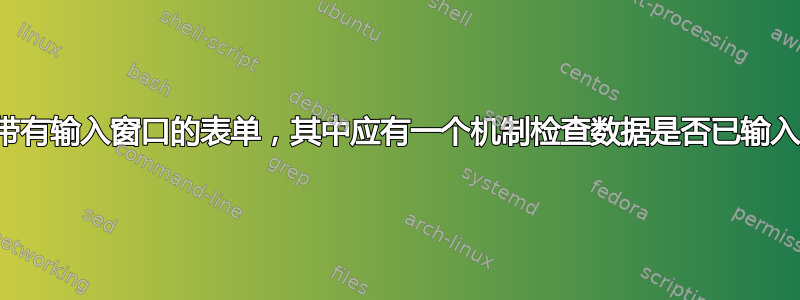
我需要它在用户按下 Enter 或 Exit 时不关闭,以便强制用户输入所有三个框。我是 powershell 新手,所以我可能听起来很尴尬,但我找不到任何地方的好解决方案。第一个文本框用于用户名第二个 Dropbox 用于国家/地区第三个 Dropbox 用于城市
还需要每个框的标题
function GenerateForm {
#region Import the Assemblies
[reflection.assembly]::loadwithpartialname("System.Windows.Forms") | Out-Null
[reflection.assembly]::loadwithpartialname("System.Drawing") | Out-Null
#endregion
#region Generated Form Objects
$form1 = New-Object System.Windows.Forms.Form
$button1 = New-Object System.Windows.Forms.Button
$button2 = New-Object System.Windows.Forms.Button
$textBox2 = New-Object System.Windows.Forms.TextBox
$textBox1 = New-Object System.Windows.Forms.TextBox
$comboBox1 = New-Object System.Windows.Forms.ComboBox
$InitialFormWindowState = New-Object System.Windows.Forms.FormWindowState
#endregion Generated Form Objects
#region $handler_comboBox1_SelectedIndexChanged
$handler_comboBox1_SelectedIndexChanged=
{
#TODO: Place custom script here
try{
if ($comboBox1.Text.Length -gt 0)
{
$form1.Text = $comboBox1.Text;
}
}catch{} #in case no items are available
}
#endregion $handler_comboBox1_SelectedIndexChanged
#region $handler_button1_Click
$handler_button1_Click=
{
#TODO: Place custom script here
try{
#How to add one item
#$textBox1.Text
if ($textBox1.Text.Length -gt 0 -and `
$textBox2.Text.Length -eq 0)
{
$comboBox1.Items.Add($textBox1.Text);
Write-Host "Added " $textBox1.Text "from `$textBox1";
}
#$textBox2.Text
elseif ($textBox1.Text.Length -eq 0 -and `
$textBox2.Text.Length -gt 0)
{
$comboBox1.Items.Add($textBox2.Text);
Write-Host "Added " $textBox2.Text "from `$textBox2";
}
#$textBox1.Text and $textBox2.Text
elseif ($textBox1.Text.Length -gt 0 -and `
$textBox2.Text.Length -gt 0)
{
$comboBox1.Items.AddRange(($textBox1.Text, $textBox2.Text));
Write-Host "Added " $textBox1.Text "from `$textBox1";
Write-Host "Added " $textBox2.Text "from `$textBox2";
}
else
{
#Nothing to add
#$textBox1.Text.Length -eq 0
#$textBox2.Text.Length -eq 0
}
#At last, if the user typed something
if ($comboBox1.Text.Length -gt 0)
{
$comboBox1.Items.Add($comboBox1.Text);
Write-Host "Added " $comboBox1.Text "from `$comboBox1";
}
}catch{} #for example, no data in the both textboxes
}
#endregion $handler_button1_Click
#region $handler_button2_Click
$handler_button2_Click=
{
#TODO: Place custom script here
try{
#Clean up the combo box
$comboBox1.Items.Clear();
$comboBox1.Text = "";
}catch{}
}
#endregion $handler_button2_Click
$OnLoadForm_StateCorrection=
{#Correct the initial state of the form to prevent the .Net maximized form issue
$form1.WindowState = $InitialFormWindowState
}
#----------------------------------------------
#region Generated Form Code
#region $form1
$form1.Text = "Input Form"
$form1.Name = "form1"
$form1.DataBindings.DefaultDataSourceUpdateMode = 0
$System_Drawing_Size = New-Object System.Drawing.Size
$System_Drawing_Size.Width = 282
$System_Drawing_Size.Height = 220
$form1.ClientSize = $System_Drawing_Size
#endregion $form1
#region $button1
$button1.TabIndex = 3
$button1.Name = "Enter"
$System_Drawing_Size = New-Object System.Drawing.Size
$System_Drawing_Size.Width = 70
$System_Drawing_Size.Height = 30
$button1.Size = $System_Drawing_Size
$button1.UseVisualStyleBackColor = $True
$button1.Text = "Enter"
$System_Drawing_Point = New-Object System.Drawing.Point
$System_Drawing_Point.X = 195
$System_Drawing_Point.Y = 160
$button1.Location = $System_Drawing_Point
$button1.DataBindings.DefaultDataSourceUpdateMode = 0
$button1.add_Click($handler_button1_Click)
$form1.Controls.Add($button1)
#endregion $button1
#region $button2
$button2.TabIndex = 4
$button2.Name = "Exit"
$System_Drawing_Size = New-Object System.Drawing.Size
$System_Drawing_Size.Width = 70
$System_Drawing_Size.Height = 30
$button2.Size = $System_Drawing_Size
$button2.UseVisualStyleBackColor = $True
$button2.Text = "Exit"
$System_Drawing_Point = New-Object System.Drawing.Point
$System_Drawing_Point.X = 15
$System_Drawing_Point.Y = 160
$button2.Location = $System_Drawing_Point
$button2.DataBindings.DefaultDataSourceUpdateMode = 0
$button2.add_Click($handler_button2_Click)
$form1.Controls.Add($button2)
#endregion $button2
#region $textBox1
$System_Drawing_Size = New-Object System.Drawing.Size
$System_Drawing_Size.Width = 250
$System_Drawing_Size.Height = 20
$textBox1.Size = $System_Drawing_Size
$textBox1.DataBindings.DefaultDataSourceUpdateMode = 0
$textBox1.Name = "textBox1"
$System_Drawing_Point = New-Object System.Drawing.Point
$System_Drawing_Point.X = 15
$System_Drawing_Point.Y = 70
$textBox1.Location = $System_Drawing_Point
$textBox1.TabIndex = 1
$form1.Controls.Add($textBox1)
#endregion $textBox1
#region $textBox2
$System_Drawing_Size = New-Object System.Drawing.Size
$System_Drawing_Size.Width = 250
$System_Drawing_Size.Height = 20
$textBox2.Size = $System_Drawing_Size
$textBox2.DataBindings.DefaultDataSourceUpdateMode = 0
$textBox2.Name = "textBox2"
$System_Drawing_Point = New-Object System.Drawing.Point
$System_Drawing_Point.X = 15
$System_Drawing_Point.Y = 120
$textBox2.Location = $System_Drawing_Point
$textBox2.TabIndex = 2
$form1.Controls.Add($textBox2)
#endregion $textBox2
#region $comboBox1
$comboBox1.FormattingEnabled = $True
$System_Drawing_Size = New-Object System.Drawing.Size
$System_Drawing_Size.Width = 250
$System_Drawing_Size.Height = 21
$comboBox1.Size = $System_Drawing_Size
$comboBox1.DataBindings.DefaultDataSourceUpdateMode = 0
$comboBox1.Name = "comboBox1"
$System_Drawing_Point = New-Object System.Drawing.Point
$System_Drawing_Point.X = 15
$System_Drawing_Point.Y = 20
$comboBox1.Location = $System_Drawing_Point
$comboBox1.TabIndex = 0
$comboBox1.add_SelectedIndexChanged($handler_comboBox1_SelectedIndexChanged)
$comboBox1.DropDownStyle =
[System.Windows.Forms.ComboBoxStyle]::DropDown;
$comboBox1.Sorted = $true;
$comboBox1.AutoCompleteCustomSource.Add("System.Windows.Forms");
$comboBox1.AutoCompleteCustomSource.AddRange(("System.Data", "Microsoft"));
$comboBox1.AutoCompleteMode =
[System.Windows.Forms.AutoCompleteMode]::SuggestAppend;
$comboBox1.AutoCompleteSource =
[System.Windows.Forms.AutoCompleteSource]::CustomSource;
$form1.Controls.Add($comboBox1)
#endregion $comboBox1
#endregion Generated Form Code
#Save the initial state of the form
$InitialFormWindowState = $form1.WindowState
#Init the OnLoad event to correct the initial state of the form
$form1.add_Load($OnLoadForm_StateCorrection)
#Show the Form
$form1.ShowDialog()| Out-Null
} #End Function
#Call the Function
GenerateForm
答案1
以下是我在评论中尝试推荐的 q&d(快速而粗略)方法。
如果您尝试通过 WinForm“X”、Windows“Alt+F4”或甚至 TaskMgrs 的结束任务关闭表单,它会向您发出警告并重新启动以便用户重试。
Add-Type -AssemblyName System.Windows.Forms
Add-Type -AssemblyName System.Drawing
[System.Windows.Forms.Application]::EnableVisualStyles()
$form = New-Object System.Windows.Forms.Form
$form.Text = 'New user GUI'
$form.Size = New-Object System.Drawing.Size(300,200)
$form.StartPosition = 'CenterScreen'
$OKButton = New-Object System.Windows.Forms.Button
$OKButton.Location = New-Object System.Drawing.Point(75,120)
$OKButton.Size = New-Object System.Drawing.Size(75,23)
$OKButton.Text = 'OK'
$OKButton.DialogResult = [System.Windows.Forms.DialogResult]::OK
$OKButton.Enabled = $false
$form.AcceptButton = $OKButton
$form.Controls.Add($OKButton)
$label = New-Object System.Windows.Forms.Label
$label.Location = New-Object System.Drawing.Point(10,20)
$label.Size = New-Object System.Drawing.Size(280,20)
$label.Text = 'Enter some stuff'
$form.Controls.Add($label)
$textBox = New-Object System.Windows.Forms.TextBox
$textBox.Location = New-Object System.Drawing.Point(10,40)
$textBox.Size = New-Object System.Drawing.Size(260,20)
$form.Controls.Add($textBox)
$form.Topmost = $True
$form.Add_Shown({$textBox.Select()})
$textbox.Add_KeyDown(
{$OKButton.Enabled = $True}
)
do
{
$result = $form.ShowDialog()
if ([string]::IsNullOrEmpty($textbox.text))
{{[System.Windows.Forms.MessageBox]::Show('Please enter stuff, because I cannot proceed until you do.', 'Warning Message',1)}}
}
until(![string]::IsNullOrEmpty($textbox.text))
if ($result -eq [System.Windows.Forms.DialogResult]::OK)
{($UserInput = $textBox.Text)}
***笔记:
不过我明白你的目的。这真的不是一个好的用户体验。用户应该始终能够退出他们正在使用的任何应用程序以继续执行其他任务。***
我可以向你保证,这个用例会激怒很多人。