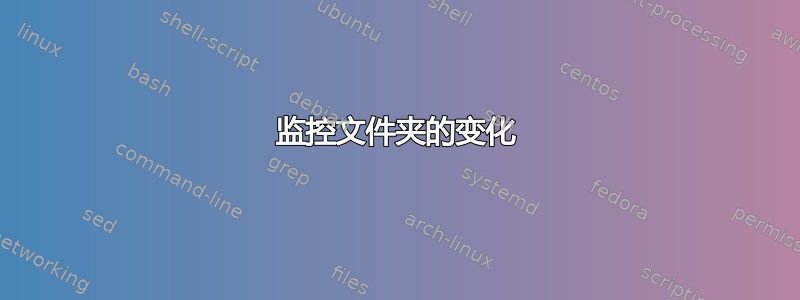
我需要一些帮助来应对一个挑战,我需要创建一个应用程序来跟踪文件夹中的任何更改,我发现本文在 Microsoft 上并尝试根据我的需求执行此操作,我需要跟踪任何更改以及哪些用户执行了这些更改。但是当我在服务器上运行它时,所有更改都是从我的用户名进行的,但我需要查看哪些用户执行了这些更改。
有人可以帮忙解决这个问题吗?
using System;
using System.IO;
namespace MyNamespace
{
class NewClassCS
{
private static string logFilePath;
private static string lastChange;
static void Main()
{
string currentDirectory = Directory.GetCurrentDirectory();
Console.WriteLine($"Monitoring folder: {currentDirectory}");
Console.WriteLine("Enter the path for the log file (or press Enter to use default 'log.txt'):");
string userInput = Console.ReadLine();
// Set default log file path in the current directory if user input is empty
logFilePath = string.IsNullOrWhiteSpace(userInput) ? Path.Combine(currentDirectory, "log.txt") : userInput;
// Check if the log file exists, create it if it doesn't
if (!File.Exists(logFilePath))
{
try
{
using (StreamWriter sw = File.CreateText(logFilePath))
{
sw.WriteLine("Log file created.");
}
}
catch (Exception ex)
{
Console.WriteLine($"Failed to create log file: {ex.Message}");
return;
}
}
using var watcher = new FileSystemWatcher(currentDirectory);
watcher.NotifyFilter = NotifyFilters.Attributes
| NotifyFilters.CreationTime
| NotifyFilters.DirectoryName
| NotifyFilters.FileName
| NotifyFilters.LastAccess
| NotifyFilters.LastWrite
| NotifyFilters.Security
| NotifyFilters.Size;
// Event handlers for file system changes
watcher.Created += OnCreated;
watcher.Deleted += OnDeleted;
watcher.Renamed += OnRenamed;
watcher.Changed += OnChanged; // Added to handle directory changes
watcher.Error += OnError;
watcher.IncludeSubdirectories = true; // Monitor subdirectories
watcher.EnableRaisingEvents = true; // Enable raising events
Console.WriteLine($"Monitoring folder: {currentDirectory}");
Console.WriteLine($"Logging changes to: {logFilePath}");
Console.WriteLine("Press enter to exit.");
Console.ReadLine(); // Wait for user input
}
private static void WriteToLogFile(string message)
{
try
{
using (StreamWriter writer = new StreamWriter(logFilePath, true))
{
string username = Environment.UserName; // Get current user's name
writer.WriteLine($"{DateTime.Now}: {username} - {message}"); // Include username in the log message
}
}
catch (Exception ex)
{
Console.WriteLine($"Failed to write to log file: {ex.Message}");
}
}
private static void OnCreated(object sender, FileSystemEventArgs e)
{
if (e.FullPath != lastChange)
{
WriteToLogFile($"Created: {e.FullPath}");
lastChange = e.FullPath;
}
}
private static void OnDeleted(object sender, FileSystemEventArgs e)
{
if (e.FullPath != lastChange)
{
WriteToLogFile($"Deleted: {e.FullPath}");
lastChange = e.FullPath;
}
}
private static void OnRenamed(object sender, RenamedEventArgs e)
{
if (e.OldFullPath != lastChange && e.FullPath != lastChange)
{
WriteToLogFile($"Renamed: {e.OldFullPath} -> {e.FullPath}");
lastChange = e.FullPath;
}
}
private static void OnChanged(object sender, FileSystemEventArgs e)
{
if (e.FullPath != lastChange)
{
WriteToLogFile($"Changed: {e.FullPath}");
lastChange = e.FullPath;
}
}
private static void OnError(object sender, ErrorEventArgs e) =>
Console.WriteLine($"Error: {e.GetException().Message}");
}
}