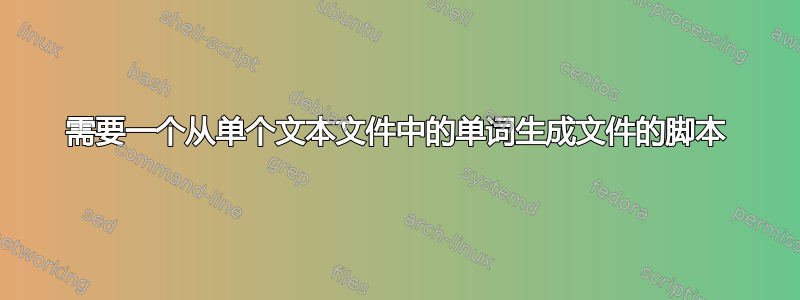
我有以下场景,我缺乏 Linux 知识并没有多大帮助。
我有一个包含 YML 值的文本文件,内容如下:
coolregion:
min: {z: -99613.0, y: 45.0, x: -99805.0}
flags: {vehicle-place: allow}
max: {z: 100387.0, y: 127.0, x: 100195.0}
priority: 0
type: cuboid
owners:
groups: [jacob, eithan, michael]
members:
groups: [jack, noah]
niceregion:
min: {z: 544.0, y: 6.0, x: 184.0}
flags: {}
max: {z: 556.0, y: 13.0, x: 197.0}
priority: 0
type: cuboid
owners:
groups: [noah]
members:
groups: [logan, lucas, jack]
我想为每个名称及其所属区域生成文件。
例如noah.txt
将包含coolregion, niceregion
而jacob.txt
将仅包含coolregion
。
我非常了解正则表达式,所以如果您只向我指出正确的方向(即只需要正则表达式即可完成的脚本),我也会很高兴。
如果重要的话,我的 Linux 版本是“Debian GNU/Linux 5.0”。
答案1
这是一个 awk 解决方案。我不知道 YML,所以您可能需要摆弄正则表达式(例如,区域标记可以缩进吗?)。请注意,该print data >filename
构造会在第一次到达给定文件名时创建或截断文件,然后附加到该文件。
<input.yml awk '
/^[^ :]+: *$/ {sub(/: *$/,""); region=$0} # start of region
/^ *groups:/ { # owner or member list
sub(/^[^:]*: *\[/, ""); sub(/\].*/, ""); # extract bracketed names
split($0, names, / *, */); # split comma-separated list
for (n in names) # iterate over names
print region >names[n] ".txt"; # write or append to name file
}'
不要太认真,但这里有一个 sed 和 shell 解决方案。
<input.yml sed -n -e '/^[^ ]/ h' \
-e '/^ \+groups:/ {' \
-e 'G' \
-e 's/^[^:]*: *\[\(.*\)\]\n\(.*\):/\2,\1/' \
-e 's/, \+/,/g' \
-e 'p' -e '}' | (
IFS=,; set -f
while read -r region names; do
for name in $names; do
echo "$region" >>"$name.txt"
done
done
)
答案2
一个“perl”解决方案:
脚本:
use warnings;
use strict;
die "Usage: perl $0 file\n" unless @ARGV == 1;
my (%hash, $region);
open my $fh, "<", $ARGV[0] or die "Cannot open file $ARGV[0]: $!\n";
while ( my $line = <$fh> ) {
## Get region, characters until first ':' without spaces at the beginning.
$region = $1 if $line =~ /^([^:\s]+)/;
## Get names with a regex and save them as keys of a hash, values will be
## regions.
if ( $line =~ /^\s*(?i:groups):\s*\[([^\]]*)\]\s*$/ ) {
my @names = split /,\s*/, $1;
for my $name ( @names ) {
push @{ $hash{ $name } }, $region;
}
}
}
## Read names (keys of the hash), open a file for each one and write regions on it.
for my $name ( sort keys %hash ) {
my $outfile = $name . ".txt";
open my $ofh, ">", $outfile or do { warn "Cannot open $outfile: $!\n"; next };
print $ofh join( ", ", @{ $hash{ $name } } ), "\n";
close $ofh or warn "Cannot close $outfile\n";
}
close $fh or warn "Cannot close $ARGV[0]\n";
执行:
$ perl script.pl infile