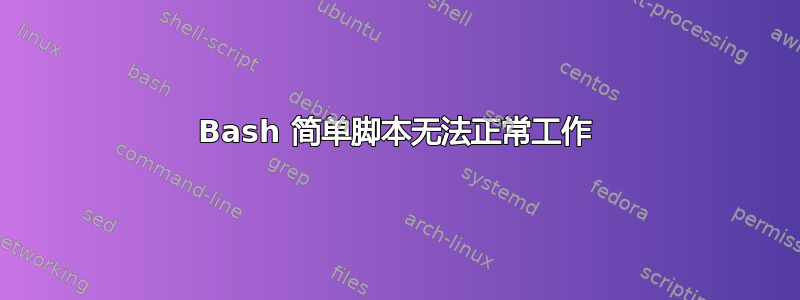
我正在尝试使用此 bash 代码来获取 Raspberry Pi 的温度,转换为华氏温度并显示华氏温度和摄氏度,然后保存为文本文件:
#!/bin/bash
echo ""
cpu="$(/opt/vc/bin/vcgencmd measure_temp)"
far=$((cpu/1000))
far2=$((far*9))
far3=$((far2/5))
far4=$((far3+32))
echo "CPU => $((cpu/1000))' C or $((far4))' F"
echo ""
echo "Pi temp $far4 degrees F" > /var/www/html/pitemp.txt
但是,当我运行它时,华氏度可以,但为什么不显示摄氏度?
pi@raspberrypi:~ $ nano checkTemp.sh
pi@raspberrypi:~ $ ./checkTemp.sh
./checkTemp.sh: line 3: temp=40.0'C: command not found
CPU => 0' C or 32' F
pi@raspberrypi:~ $ /opt/vc/bin/vcgencmd measure_temp
temp=40.0'C
我检查了 cpu 和 far 的值,但它似乎不起作用。有什么建议吗?[已解决]
#!/usr/bin/env bash
echo ""
cpu="$(/opt/vc/bin/vcgencmd measure_temp | sed -E 's/.*=([0-9.]*).*/\1/')"
## convert to Fahrenheit
far=$( echo "$cpu * 9/5 + 32" | bc -l)
## Remove extra decimal digits
printf "CPU => %.2f' C or %.2f' F\n" "$cpu" "$far"
echo ""
printf "Pi temp %.2f degrees F\n" "$cpu" > /var/www/html/pitemp.txt
echo "C:$cpu F:$far"
这个新代码正在工作,但是华氏温度似乎有问题,因为输出是:
pi@raspberrypi:~ $ nano checkTemp.sh
pi@raspberrypi:~ $ ./checkTemp.sh
./checkTemp.sh: line 5: bc: command not found
CPU => 43.00' C or 0.00' F
C:43.0 F:
pi@raspberrypi:~ $ /opt/vc/bin/vcgencmd measure_temp
temp=43.0'C
pi@raspberrypi:~ $
答案1
你的$cpu
变量成立temp=40.0'C
。这不是一个数字,您不能将其除或乘,您需要首先提取实际的数值。所以你需要出去40.0
。您可以通过以下方式执行此操作:
cpu="$(/opt/vc/bin/vcgencmd measure_temp | sed -E 's/.*=([0-9.]*).*/\1/')"
下一个问题是 bash 无法进行浮点运算。所以它不能处理非整数值。您需要另一个工具,例如bc
:
far=$(echo "$cpu/1000" | bc -l)
当然,由于你的CPU温度永远不会高于(或远远接近)1000摄氏度,所以这种划分不可能是正确的。将摄氏度转换为华氏度的公式似乎是:
T(°F) = T(°C) × 9/5 + 32
您可以一步完成此操作。将所有这些放在一起,这应该是脚本的工作版本:
#!/usr/bin/env bash
echo ""
cpu="$(/opt/vc/bin/vcgencmd measure_temp | sed -E 's/.*=([0-9.]*).*/\1/')"
## convert to Fahrenheit
far=$( echo "$cpu * 9/5 + 32" | bc -l)
## Remove extra decimal digits
printf "CPU => %.2f °C or %.2f °F\n" "$cpu" "$far"
echo ""
printf "Pi temp %.2f degrees F\n" "$far" > /var/www/html/pitemp.txt
如果你没有bc
,你可以使用这个:
#!/usr/bin/env bash
echo ""
cpu="$(/opt/vc/bin/vcgencmd measure_temp | sed -E 's/.*=([0-9.]*).*/\1/')"
## convert to Fahrenheit
far=$( perl -le 'print $ARGV[0] * 9/5 + 32' "$cpu")
## Remove extra decimal digits
printf "CPU => %.2f °C or %.2f °F\n" "$cpu" "$far"
echo ""
printf "Pi temp %.2f degrees F\n" "$far" > /var/www/html/pitemp.txt