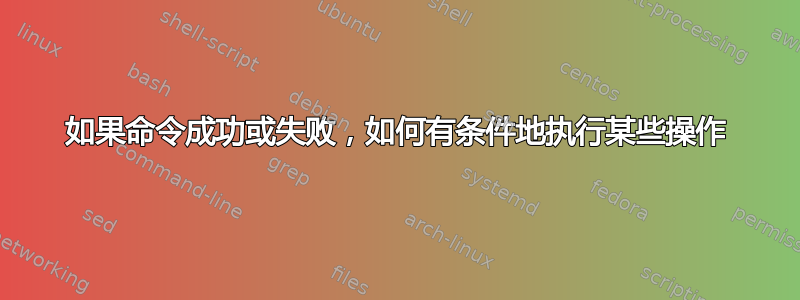
我怎样才能在 bash 中做这样的事情?
if "`command` returns any error";
then
echo "Returned an error"
else
echo "Proceed..."
fi
答案1
如果命令成功或失败,如何有条件地执行某些操作
这正是 bashif
语句的作用:
if command ; then
echo "Command succeeded"
else
echo "Command failed"
fi
从评论中添加信息:您不在这种情况下需要使用[
...语法。本身就是一个命令,几乎等同于.它可能是 中最常用的命令,这可能会导致假设它是 shell 语法的一部分。但如果您想测试命令是否成功,请直接将命令本身与 一起使用,如上所示。]
[
test
if
if
答案2
对于您希望在 shell 命令起作用时发生的小事情,您可以使用以下&&
结构:
rm -rf somedir && trace_output "Removed the directory"
同样,对于您希望在 shell 命令失败时发生的小事情,您可以使用||
:
rm -rf somedir || exit_on_error "Failed to remove the directory"
或两者
rm -rf somedir && trace_output "Removed the directory" || exit_on_error "Failed to remove the directory"
对这些结构做太多事情可能是不明智的,但它们有时可以使控制流程更加清晰。
答案3
检查 的值$?
,其中包含执行最近命令/函数的结果:
#!/bin/bash
echo "this will work"
RESULT=$?
if [ $RESULT -eq 0 ]; then
echo success
else
echo failed
fi
if [ $RESULT == 0 ]; then
echo success 2
else
echo failed 2
fi
答案4
应该注意的是,if...then...fi
and &&
/||
方法的类型处理我们想要测试的命令返回的退出状态(成功时为 0 );但是,如果命令失败或无法处理输入,某些命令不会返回非零退出状态。这意味着通常的if
和&&
/||
方法不适用于这些特定命令。
例如,在 Linux 上,file
如果 GNU 收到不存在的文件作为参数并且find
无法找到用户指定的文件,则它仍然以 0 退出。
$ find . -name "not_existing_file"
$ echo $?
0
$ file ./not_existing_file
./not_existing_file: cannot open `./not_existing_file' (No such file or directory)
$ echo $?
0
在这种情况下,我们处理这种情况的一种可能的方法是读取stderr
/stdin
消息,例如由命令返回的消息file
,或解析命令的输出,如find
.为此目的,case
可以使用声明。
$ file ./doesntexist | while IFS= read -r output; do
> case "$output" in
> *"No such file or directory"*) printf "%s\n" "This will show up if failed";;
> *) printf "%s\n" "This will show up if succeeded" ;;
> esac
> done
This will show up if failed
$ find . -name "doesn'texist" | if ! read IFS= out; then echo "File not found"; fi
File not found