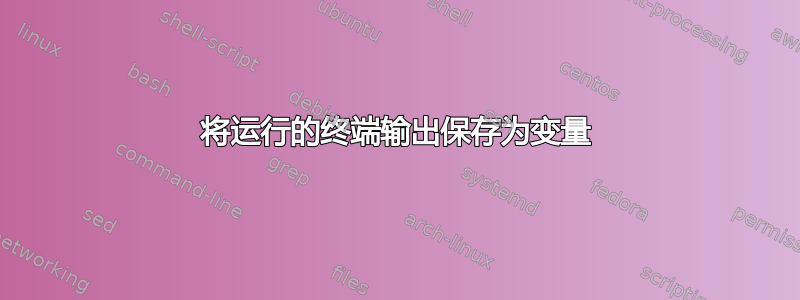
我正在运行一个使用 package.json 的 python 脚本pyserial
。我使用一块板来控制电机旋转,并通过 USB 端口连接。
该板已经编程,可以根据给定的命令旋转电机。以下是一些示例:
- 输入:检查电机状态的命令:H010100
输出 :
{
.."beambreak": [0,0,0,0,0],
.."prox": [0.003,0.003],
.."T1": [0,0],
.."T2": [0,0],
.."MT": [-1,-1]
.."test_switch": 0,
.}
- 输入:电机旋转一次的命令:H010101
输出:{“旋转”:“成功”}
任务:在“while”循环中,如何每1分钟发送命令(例如H010101),检查输出消息(例如{“Rotate”:“Successful”})并根据输出消息条件发送下一个命令。
问题:当我运行代码时,“可以设置”输出消息出现在 Linux 终端/IDE 控制台中,但我不知道将该消息保存为变量并将其应用到循环条件中。我的意思是,检查消息是否是相同的消息,等待 1 分钟并再次发送 H010101 命令?
我还尝试将文件保存在 *.log 或 *.txt 但不起作用示例:
$ python test.py >> *.txt
$ python test.py > *.log
这是我的代码:
import time
import serial
# configure the serial connections
ser = serial.Serial(
port='/dev/ttyUSB0',
baudrate=115200,
parity=serial.PARITY_NONE,
stopbits=serial.STOPBITS_ONE,
bytesize=serial.EIGHTBITS
)
while True :
print(' Check Status')
ser.write('H010000\n'.encode())
status_cmd = ser.readline()
print(status_cmd)
if status_cmd === "test_switch: 0 " : # i can't save the message as variable from running terminal
time.sleep(5)
# Command to rotate motor
ser.write('H010101\n'.encode())
# read respond of give command
reading = ser.readline()
print(reading)
if reading == {"Drop":"Successful"} : # i can't save the message as variable from running terminal
time.sleep(60)
# rotate motor
ser.write('H010101\n'.encode())
# read respond of give command
reading = ser.readline()
print(reading)
答案1
您可以做的第一件事是将函数封装到方法中(如果您愿意,也可以使用类)
检查状态
def check_status(ser):
print('Check Status')
ser.write('H010000\n'.encode())
current_status = ser.readline().decode('utf-8')
return current_status
旋转电机
def rotate_motor(ser):
print('Rotating ..')
ser.write('H010101\n'.encode())
rotation_status = ser.readline().decode('utf-8')
return rotation_status
您还需要导入 json 以将响应加载为 dict
例如
>>> import json
>>> rotation_status='{"Drop":"Successful"}'
>>> json.loads(rotation_status)
{'Drop': 'Successful'}
现在您已准备好这些代码片段,您可以调用它们以根据结果连续运行操作
while True:
status = json.loads(check_status(ser))
if status['test_switch'] == 0:
time.sleep(5)
is_rotated = json.loads(rotate_motor(ser))
if is_rotated['Drop'] == 'Successful':
time.sleep(60)
else:
print('Something went wrong')
raise Exception(is_rotated)