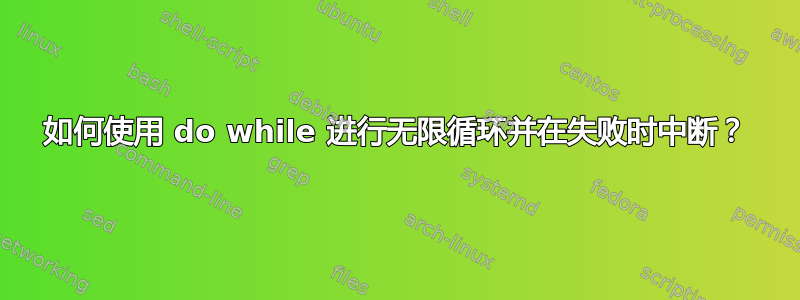
我正在尝试检查 AWS AMI 的状态并在状态为 时执行一些命令available
。下面是我实现这一目标的小脚本。
#!/usr/bin/env bash
REGION="us-east-1"
US_EAST_AMI="ami-0130c3a072f3832ff"
while :
do
AMI_STATE=$(aws ec2 describe-images --region "$REGION" --image-ids $US_EAST_AMI | jq -r .Images[].State)
[ "$AMI_STATE" == "available" ] && echo "Now the AMI is available in the $REGION region" && break
sleep 10
done
如果第一次调用成功,上面的脚本就可以正常工作。但我期待以下场景的一些东西
- 如果 的值
$AMI_STATE
等于"available"
(当前正在工作),"failed"
则应该打破循环 - 如果 的值
$AMI_STATE
等于"pending"
,则循环应继续,直到满足预期值。
答案1
您想在 的值AMI_STATE
等于pending
...时运行循环,所以就这样写。
while
AMI_STATE=$(aws ec2 describe-images --region "$REGION" --image-ids $US_EAST_AMI | jq -r .Images[].State) &&
[ "$AMI_STATE" = "pending" ]
do
sleep 10
done
case $AMI_STATE in
"") echo "Something went wrong: unable to retrieve AMI state";;
available) echo "Now the AMI is available in the $REGION region";;
failed) echo "The AMI has failed";;
*) echo "AMI in weird state: $AMI_STATE";;
esac
答案2
您可以使用一个简单的 if 构造:
#!/usr/bin/env bash
region="us-east-1"
us_east_ami="ami-0130c3a072f3832ff"
while :
do
ami_state=$(aws ec2 describe-images --region "$region" --image-ids "$us_east_ami" | jq -r .Images[].State)
if [[ $ami_state == available ]]; then
echo "Now the AMI is available in the $region region"
break
elif [[ $ami_state == failed ]]; then
echo "AMI is failed in $region region"
break
fi
sleep 10
done
case 在这里也是一个不错的选择:
#!/usr/bin/env bash
region="us-east-1"
us_east_ami="ami-0130c3a072f3832ff"
while :
do
ami_state=$(aws ec2 describe-images --region "$region" --image-ids "$us_east_ami" | jq -r .Images[].State)
case $ami_state in
available)
echo "Now the AMI is available in the $region region"
break
;;
failed)
echo "AMI is failed in $region region"
break
;;
pending) echo "AMI is still pending in $region region";;
*)
echo "AMI is in unhandled state: $ami_state"
break
;;
esac
sleep 10
done
您可以在 bash 手册中阅读有关两者的内容3.2.5.2 条件构造
或者,您可以考虑放弃无限 while 循环,转而使用直到循环:
#!/usr/bin/env bash
region="us-east-1"
us_east_ami="ami-0130c3a072f3832ff"
until [[ $ami_state == available ]]; do
if [[ $ami_state == failed ]]; then
echo "AMI is in a failed state for $region region"
break
fi
ami_state=$(aws ec2 describe-images --region "$region" --image-ids "$us_east_ami" | jq -r .Images[].State)
sleep 10
done
这将根据需要循环多次,直到状态可用。如果没有适当的错误处理,这很容易变成无限循环。 (确保除了失败之外没有任何状态可能会造成问题)