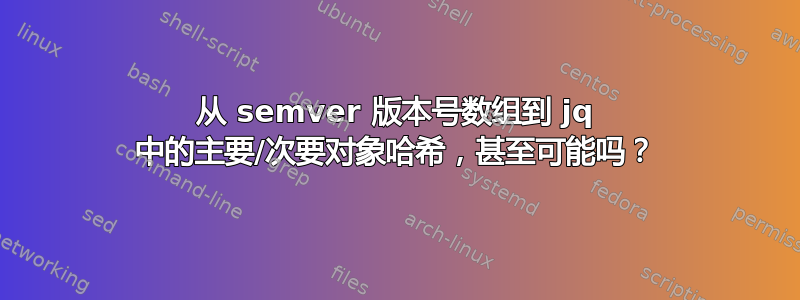
输入
[
"8.1.1",
"7.4.33",
"7.4.5",
"8.2.6"
]
输出
{
"7": "7.4.33",
"7.4": "7.4.33",
"8": "8.2.6",
"8.1": "8.1.1",
"8.2": "8.2.6"
}
输出是一个对象,其中:
- 一个键等于主要的存在(即
8
),值为最好的版本对于那个专业 - 一个键等于主要次要存在(即
7.4
),值为最好的版本对于该专业/辅修
经过大量的试验和错误,现在我只能提取专业并简化为数组。任何帮助深表感谢!
echo "[\"8.1.1\",\"7.4.33\",\"7.4.5\",\"8.2.6\"]" | jq -n 'reduce input[] as $version ({}; .[($version | split(".")[0])] += [$version])'
哪个输出:
{
"8": [
"8.1.1",
"8.2.6"
],
"7": [
"7.4.33",
"7.4.5"
]
}
编辑:作为参考并避免歧义,对于那些能够理解 PHP 的人来说,这是一个执行相同操作的 CLI 脚本:
#!/usr/bin/env php
<?php
array_shift($argv);
usort($argv, 'version_compare');
echo json_encode(
array_reduce(
array_reverse($argv),
function (array $prev, $version) use($argv) {
[$major, $minor] = explode('.', $version, 3);
$majors = array_filter($argv, function ($v) use ($major) {
return substr($v, 0, 1) === $major;
});
$minors = array_filter($argv, function ($v) use ($major, $minor) {
return substr($v, 0, 3) === "$major.$minor";
});
return $prev + [
$major => end($majors),
"$major.$minor" => end($minors),
];
},
[]
),
JSON_FORCE_OBJECT
);
答案1
可能是这样的:
jq -c '
map(split(".") | map(tonumber)) | # transform the version number strings
# to arrays of numbers so we can
# sort them.
sort | # those arrays of numbers are sorted numerically in effect
# conveniently achieving a semver sort
map(map(tostring)) | # convert the numbers in the arrays back to
# string so we can manipulate them as such more
# easily.
map({
(.[0]) : join("."),
(.[0] + "." + .[1]) : join(".")
}) | # create a {"x":"x.y.z","x.y":"x.y.z"} object for each of
# those arrays sorted in ascending version number
add # adding those objects means merging them, with the last one
# for a given key taking precedence.
' file.json
但是,尽管这样做jq
是一个有趣的大脑扭曲练习,但我认为我会使用带有 JSON 模块的通用编程语言(例如 perl/ruby/python)来解决此类问题,其中算法和代码要容易得多提出、理解并保持前进。例如,在perl
:
perl -MJSON -MSort::Versions -l -0777 -ne '
$j = decode_json($_);
for (sort versioncmp @$j) {
@p = split/\./;
$max{$p[0]} = $_;
$max{"$p[0].$p[1]"} = $_
}
print encode_json(\%max)' file.json
(这些JSON
和Sort::Versions
模块可能不会默认安装在您的系统上,但话又说回来,jq
很少有)。
或者甚至因为这里只有数字 和.
,用一些基本的 awk 来完成它(以及sort
用于版本排序的 GNU 和grep
用于提取版本号的 GNU):
grep -Po '[\d.]+' file.json |
sort -V |
awk -F. '
{
max[$1] = $0
max[$1"."$2] = $0
}
END {
printf "{"
for (i in max) {
printf c"\"%s\":\"%s\"", i, max[i]
c = ","
}
print "}"
}'