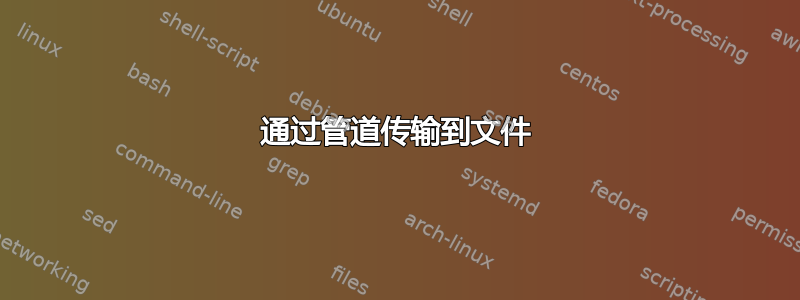
我在理解文件描述符是什么以及我是否需要一个文件描述符方面遇到了一些困惑!我正在尝试在 Node.js 中生成一个进程,并将其输出直接写入输出文件。
我知道我的问题是特定于 unix 系列范围之外的语言的,但我相信我的问题来自于我不了解有关系统而不是语言的某些事情。
这是我从脚本中调用的函数。我以为我可以这样称呼它
require('child_process').spawn('./my_script.bash 1>out.txt')
但运气不好!我知道脚本正在执行并且正在运行。
答案1
spawn 命令返回一个可读流,因此您需要将其通过管道传输到可写流才能执行一些有用的操作。
通过管道传输到文件
// the filed module makes simplifies working with the filesystem
var filed = require('filed')
var path = require('path')
var spawn = require('spawn')
var outputPath = path.join(__dirname, 'out.txt')
// filed is smart enough to create a writable stream that we can pipe to
var writableStream = filed(outputPath)
var cmd = path.join(__dirname, 'my_script.bash')
var args = [] // you can option pass arguments to your spawned process
var child = spawn(cmd, args)
// child.stdout and child.stderr are both streams so they will emit data events
// streams can be piped to other streams
child.stdout.pipe(writableStream)
child.stderr.pipe(writableStream)
child.on('error', function (err) {
console.log('an error occurred')
console.dir(err)
})
// code will be the exit code of your spawned process. 0 on success, a positive integer on error
child.on('close', function (code) {
if (code !== 0) {
console.dir('spawned process exited with error code', code)
return
}
console.dir('spawned process completed correctly at wrote to file at path', outputPath)
})
您需要安装归档模块才能运行上面的示例
npm install filed
通过管道传输到 stdout 和 stderr
process.stdout 和 process.stderr 都是可写流,因此您也可以将生成的命令的输出直接通过管道传输到控制台
var path = require('path')
var spawn = require('spawn')
var cmd = path.join(__dirname, 'my_script.bash')
var args = [] // you can option pass arguments to your spawned process
var child = spawn(cmd, args)
// child is a stream so it will emit events
child.stderr.pipe(process.stderr)
child.stdout.pipe(process.stderr)
child.on('error', function (err) {
console.log('an error occurred')
console.dir(err)
})
// code will be the exit code of your spawned process. 0 on success, a positive integer on error
child.on('close', function (code) {
if (code !== 0) {
console.dir('spawned process exited with error code', code)
return
}
console.dir('spawned process completed correctly at wrote to file at path', outputPath)
})