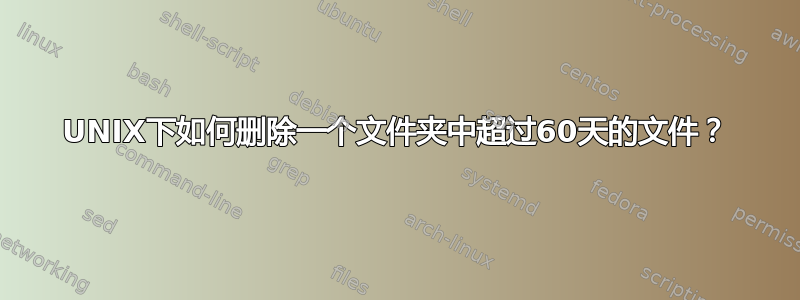
我知道如何删除修改时间超过 60 天的文件,但我想根据文件名中的时间戳删除文件。
例如,我每月每天都有以下文件,并且我有过去 3 年的这些文件。
vtm_data_12month_20140301.txt
vtm_data_12month_20140301.control
vtm_mtd_20130622.txt
vtm_mtd_20130622.control
vtm_ytd_20131031.txt
vtm_ytd_20131031.control
我想编写一个脚本,查找超过 60 天的所有文件(基于文件名),然后删除除每月最后一个文件之外的所有文件。例如,在一月份我想删除除 之外的所有内容vtm_data_12month_20140131.txt
。这里的问题是我可能会收到 1 月 30 日的文件,因此在这种情况下我不应该删除最新的文件,但我必须删除其余的文件。
请告诉我如何通过 shell 脚本实现这一点?
答案1
好的,我重新编写了这个脚本,通过向后排序,它看起来应该可以工作。它将年份和月份与前一个进行比较,如果较低,则应该是该月的最后一个条目。
#!/bin/bash
#the tac reverses the listing, so we go from newest to oldest, vital for our logic below
FILES=`ls | tac`
#create a cutoff date by taking current date minus our 60 day limit
CUTOFFDATE=`date --date="60 days ago" +%Y%m%d`
#we are setting this value to month 13 of the current year
LASTYEARMONTH=`date +%Y`13
for file in $FILES; do
#get datestamp
FILEDATE=`expr match "$file" '.*\(20[0-9][0-9][0-9][0-9][0-9][0-9]\)'`
#get year and month, ie 201410
THISYEARMONTH=${FILEDATE:0:6}
if [ ! -z $FILEDATE ] && [ $THISYEARMONTH -lt $LASTYEARMONTH ]; then
echo "$file IS THE LAST FILE OF THE MONTH. NOT DELETING"
else
#now we see if the file is older than 60 days (ie, has a LOWER unix timestamp than our cutoff)
if [ ! -z $FILEDATE ] && [ $FILEDATE -lt $CUTOFFDATE ]; then
echo "$file has a file date of $FILEDATE which is older than 60 days."
#uncomment this next line to remove
#rm $file
fi
fi
LASTYEARMONTH=$THISYEARMONTH
done
答案2
以下代码不保留每个月的最后一个文件。
#! /bin/bash
cmp_timestamp=$(date --date="60 days ago" +%Y%m%d)
while read filename; do
[[ $filename =~ _(20[0-9][0-9][01][0-9][0123][0-9])\. ]]
timestamp=${BASH_REMATCH[1]}
printf "%-40s : %s\n" "$filename" "${timestamp}"
if [ "$timestamp" -lt "$cmp_timestamp" ]; then
echo " delete this file"
: rm "$filename"
else
echo " DO NOT delete this file"
fi
echo
done <file
从man bash
:
BASH_REMATCH
An array variable whose members are assigned by the =~ binary operator to
the [[ conditional command. The element with index 0 is the portion of the
string matching the entire regular expression. The element with index n
is the portion of the string matching the nth parenthesized subexpression.
This variable is read-only.
答案3
你可以在 while 循环中尝试这个:
#!/bin/bash
A=vtm_data_12month_20140301.txt
B=`ls vtm_data_12month_20140301.txt | awk -F "_" '{print $4}' | awk -F "." '{print $1}'`
C=`date --date="60 days ago" +%Y%m%d`
if [ "$B" < "$C" ]
then
rm -fr $A
else
echo "$A is not older"
fi
答案4
下面的 python 脚本完成这项工作,可以使用变量配置应删除文件的天数days
。
#!/usr/bin/env python3
import os
import re
import datetime
days=60
delta = datetime.date.today() - datetime.timedelta(days=days)
files = [ x for x in os.listdir() if re.search('_\d{8}\.', x)]
for file in files:
date = re.search('_(\d{8})\.', file).group(1)
if datetime.datetime.strptime(date, '%Y%m%d').date() <= delta:
print('Removing file: ',file)
os.remove(file)
输出:
$ ./remove.py
Removing file: vtm_data_12month_20140301.txt
Removing file: vtm_mtd_20130622.control
Removing file: vtm_data_12month_20140301.control
Removing file: vtm_mtd_20130622.txt
Removing file: vtm_ytd_20131031.txt
Removing file: vtm_ytd_20131031.control