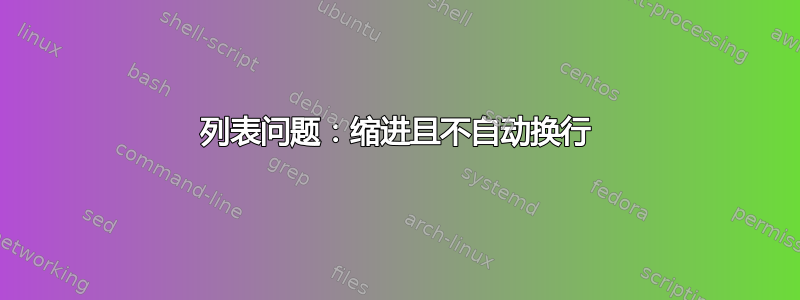
我在使用 listings 格式化 c++ 代码时遇到了一些问题。这是我的 WME:
\documentclass{standalone}
\usepackage[left=1.00in, right=1.00in, top=1.00in, bottom=1.00in]{geometry}
\usepackage{tcolorbox,listings}
\usepackage{listings}
\usepackage{tikz}
\usepackage{framed}
\usepackage{minted}
\definecolor{background}{HTML}{EEEEEE}
\definecolor{comments}{HTML}{868686}
\definecolor{darkred}{RGB}{139,0,0}
\definecolor{darkblue}{RGB}{0,0,139}
\definecolor{chartreuse}{RGB}{127,255,0}
\definecolor{drakgreen}{RGB}{0,128,0}
\definecolor{lightgray}{RGB}{238,239,240}
\lstset{
language=[LaTeX]Tex,
keywordstyle=\color{darkblue},
texcsstyle=*\color{blue},
basicstyle=\normalfont\ttfamily,
commentstyle=\color{comments}\ttfamily,
stringstyle=\rmfamily,
numbers=none,
showstringspaces=false,
breaklines=true,
frameround=ftff,
captionpos=t,
belowcaptionskip=0em,
belowskip=0em,
}
\lstdefinestyle{mystyle}{
language=C++,
extendedchars=true,
breaklines=true,
breakatwhitespace=true,
basicstyle=\ttfamily,
keywordstyle=\color{darkblue},
keywordstyle=[2]\color{blue},
keywordstyle=[3]\color{darkblue},
keywordstyle=[4]\color{drakgreen},
alsoletter = {!},
keywords=[2]{cout,cin},
}
\tcbuselibrary{listings,skins,breakable}
\newtcblisting{code}{
arc=0mm,
top=0mm,
bottom=0mm,
left=3mm,
right=0mm,
width=\textwidth,
boxrule=1pt,
colback=lightgray,
listing only,
listing options={style=mystyle},
breakable
}
\begin{document}
\begin{code}
/*
* C++ Program to Implement The Edmonds-Karp Algorithm
*/
#include<cstdio>
#include<cstdio>
#include<queue>
#include<cstring>
#include<vector>
#include<iostream>
#include<conio.h>
using namespace std;
int capacities[10][10];
int flowPassed[10][10];
vector<int> graph[10];
int parentsList[10];
int currentPathCapacity[10];
int bfs(int startNode, int endNode)
{
memset(parentsList, -1, sizeof(parentsList));
memset(currentPathCapacity, 0, sizeof(currentPathCapacity));
queue<int> q;
q.push(startNode);
parentsList[startNode] = -2;
currentPathCapacity[startNode] = 999;
while (!q.empty())
{
int currentNode = q.front();
q.pop();
for (int i = 0; i < graph[currentNode].size(); i++)
{
int to = graph[currentNode][i];
if (parentsList[to] == -1)
{
if (capacities[currentNode][to] - flowPassed[currentNode][to] > 0)
{
parentsList[to] = currentNode;
currentPathCapacity[to] = min(currentPathCapacity[currentNode],capacities[currentNode][to] - flowPassed[currentNode][to]);
if (to == endNode)
{
return currentPathCapacity[endNode];
}
q.push(to);
}
}
}
}
return 0;
}
int edmondsKarp(int startNode, int endNode)
{
int maxFlow = 0;
while (true)
{
int flow = bfs(startNode, endNode);
if (flow == 0)
{
break;
}
maxFlow += flow;
int currentNode = endNode;
while (currentNode != startNode)
{
int previousNode = parentsList[currentNode];
flowPassed[previousNode][currentNode] += flow;
flowPassed[currentNode][previousNode] -= flow;
currentNode = previousNode;
}
}
return maxFlow;
}
int main()
{
int nodesCount, edgesCount;
cout << "enter the number of nodes and edges\n";
cin >> nodesCount >> edgesCount;
int source, sink;
cout << "enter the source and sink\n";
cin >> source >> sink;
for (int edge = 0; edge < edgesCount; edge++)
{
cout << "enter the start and end vertex alongwith capacity\n";
int from, to, capacity;
cin >> from >> to >> capacity;
capacities[from][to] = capacity;
graph[from].push_back(to);
graph[to].push_back(from);
}
int maxFlow = edmondsKarp(source, sink);
cout << endl << endl << "Max Flow is:" << maxFlow << endl;
getch();
}
\end{code}
\end{document}
从输出结果可以看出两个问题,缩进过多,没有自动换行,问题到底出在哪里?
答案1
好的,这是您的代码的整理版本:
- 如果您使用列表排版,则不需要像 minted 这样的包。
- 您的代码使用空格缩进,因此源中的每个空格都会转换为输出中的一个空格。只需运行搜索和替换即可“修复”过多的空格。如果您使用“真正的”制表符,您可能需要指定
tabsize
。 - 如果您想显示可破坏行为和固定宽度(因为它会根据内容调整页面大小),使用独立类不是一个好主意。
- 您仅指定了
breaklines,breakatwhitespace=true
。因此,您的长行调用min
将无法正确中断,因为没有空格来中断。您可能需要在逗号后添加一个空格。
代码:
\documentclass{article}
\usepackage[left=1.00in, right=1.00in, top=1.00in, bottom=1.00in]{geometry}
\usepackage[listings,skins,breakable]{tcolorbox}
\definecolor{darkred}{RGB}{139,0,0}
\definecolor{darkblue}{RGB}{0,0,139}
\definecolor{darkgreen}{RGB}{0,128,0}
\definecolor{lightgray}{RGB}{238,239,240}
\lstdefinestyle{mystyle}{
language=C++,
extendedchars=true,
breaklines=true,
breakatwhitespace=true,
basicstyle=\ttfamily,
keywordstyle=\color{darkblue},
keywordstyle=[2]\color{blue},
keywordstyle=[3]\color{darkblue},
keywordstyle=[4]\color{darkgreen},
alsoletter = {!},
tabsize=2,
keywords=[2]{cout,cin},
}
\newtcblisting{code}{
arc=0mm,
top=0mm,
bottom=0mm,
left=3mm,
right=0mm,
width=\textwidth,
boxrule=1pt,
colback=lightgray,
listing only,
listing options={style=mystyle},
breakable
}
\begin{document}
\begin{code}
/*
* C++ Program to Implement The Edmonds-Karp Algorithm
*/
#include<cstdio>
#include<cstdio>
#include<queue>
#include<cstring>
#include<vector>
#include<iostream>
#include<conio.h>
using namespace std;
int capacities[10][10];
int flowPassed[10][10];
vector<int> graph[10];
int parentsList[10];
int currentPathCapacity[10];
int bfs(int startNode, int endNode)
{
memset(parentsList, -1, sizeof(parentsList));
memset(currentPathCapacity, 0, sizeof(currentPathCapacity));
queue<int> q;
q.push(startNode);
parentsList[startNode] = -2;
currentPathCapacity[startNode] = 999;
while (!q.empty())
{
int currentNode = q.front();
q.pop();
for (int i = 0; i < graph[currentNode].size(); i++)
{
int to = graph[currentNode][i];
if (parentsList[to] == -1)
{
if (capacities[currentNode][to] - flowPassed[currentNode][to] > 0)
{
parentsList[to] = currentNode;
currentPathCapacity[to] = min(currentPathCapacity[currentNode], capacities[currentNode][to] - flowPassed[currentNode][to]);
if (to == endNode)
{
return currentPathCapacity[endNode];
}
q.push(to);
}
}
}
}
return 0;
}
int edmondsKarp(int startNode, int endNode)
{
int maxFlow = 0;
while (true)
{
int flow = bfs(startNode, endNode);
if (flow == 0)
{
break;
}
maxFlow += flow;
int currentNode = endNode;
while (currentNode != startNode)
{
int previousNode = parentsList[currentNode];
flowPassed[previousNode][currentNode] += flow;
flowPassed[currentNode][previousNode] -= flow;
currentNode = previousNode;
}
}
return maxFlow;
}
int main()
{
int nodesCount, edgesCount;
cout << "enter the number of nodes and edges\n";
cin >> nodesCount >> edgesCount;
int source, sink;
cout << "enter the source and sink\n";
cin >> source >> sink;
for (int edge = 0; edge < edgesCount; edge++)
{
cout << "enter the start and end vertex alongwith capacity\n";
int from, to, capacity;
cin >> from >> to >> capacity;
capacities[from][to] = capacity;
graph[from].push_back(to);
graph[to].push_back(from);
}
int maxFlow = edmondsKarp(source, sink);
cout << endl << endl << "Max Flow is:" << maxFlow << endl;
getch();
}
\end{code}
\end{document}