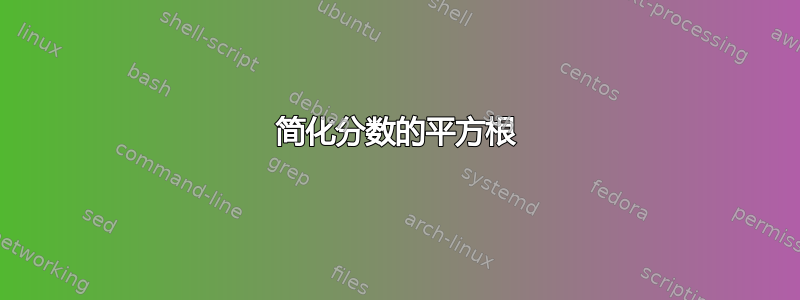
答案1
这是一个 LuaLaTeX 解决方案。有点过头了,但你可以将上述函数用于其他目的:
%!TEX program = lualatex
\documentclass{article}
\usepackage{luacode}
\begin{luacode*}
function IsPrime(d)
-- Determines whether a number is prime or not
local dummy = 0
for i = 1, math.floor(math.sqrt(d)) do
if d % i == 0 then
dummy = dummy + 1
end
end
if dummy == 1 then return true else return false end
end
--Prime factorization
--This function return two tables, the former with prime factors, the latter with their respective exponents
function PrimeFactors(d)
local dummy1 = {}
local dummy2 = {}
--Search and collect prime factors
for i= 2, math.ceil(math.sqrt(d)) do
if d%i == 0 and IsPrime(i) == true then
table.insert(dummy1,i)
end
end
--A void table means d is prime, so we add in that scenario.
if #dummy1 == 0 then table.insert(dummy1,d) end
--Now we search indices
for k,v in ipairs(dummy1) do
if (d%dummy1[k]==0) then
while (d%dummy1[k]==0) do
if dummy2[k] == nil then dummy2[k] = 0 else
dummy2[k] = dummy2[k] + 1
d = d/dummy1[k]
end
end
end
end
--We have both the factors and the indices
local dummy3 = {dummy1, dummy2}
return dummy3
end
--GCD
function GCD(a,b)
if b ~= 0 then
return GCD(b, a % b)
else
return math.abs(a)
end
end
--This multiplies factors to d so it becomes a square
function CompleteSquare(d)
local dummy = 1
for i,v in ipairs(PrimeFactors(d)[1]) do
dummy = dummy * PrimeFactors(d)[1][i]^(2*math.ceil(0.5*PrimeFactors(d)[2][i]))
end
return math.floor(dummy/d)
end
--Check
function IsSquare(d)
if math.floor(d) - (math.floor(math.sqrt(d))^2) == 0 then return true else return false end
end
--Et voilà
function rsqrt(a,b)
local A = (a/GCD(a,b))*CompleteSquare(b/GCD(a,b))
local B = (b/GCD(a,b))*CompleteSquare(b/GCD(a,b))
if IsSquare(A) == true then
tex.print( [===[\frac{]===] .. math.floor(math.sqrt(A)) .. [===[}{]===] .. math.floor(math.sqrt(B)) .. [===[}]===])
else
tex.print( [===[\frac{\sqrt{]===] .. math.floor(A) .. [===[}}{]===] .. math.floor(math.sqrt(B)) .. [===[}]===])
end
end
\end{luacode*}
\newcommand{\rsqrt}[2]{\directlua{rsqrt(#1,#2)}}
\begin{document}
%Some examples
$\rsqrt{1}{23}$, $\rsqrt{70}{45}$, $\rsqrt{50}{18}$, $\rsqrt{12}{16}$
\end{document}