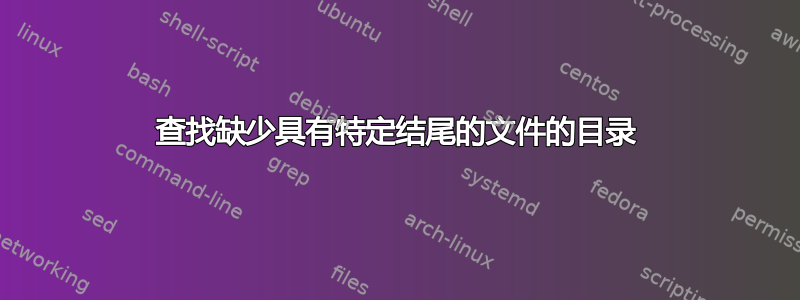
我想显示所有不包含特定文件结尾的文件的目录。因此我尝试使用以下代码:
find . -type d \! -exec test -e '{}/*.ENDING' \; -print
在这个例子中,我想显示所有不包含以 结尾的文件的目录.ENDING
,但是这不起作用。
我的错误在哪里?
答案1
以下是分三步解决的方法:
temeraire:tmp jenny$ find . -type f -name \*ENDING -exec dirname {} \; |sort -u > /tmp/ending.lst
temeraire:tmp jenny$ find . -type d |sort -u > /tmp/dirs.lst
temeraire:tmp jenny$ comm -3 /tmp/dirs.lst /tmp/ending.lst
答案2
开始了!
#!/usr/bin/env python3
import os
for curdir,dirnames,filenames in os.walk('.'):
if len(tuple(filter(lambda x: x.endswith('.ENDING'), filenames))) == 0:
print(curdir)
或者另外一种(更符合 Python 风格):
#!/usr/bin/env python3
import os
for curdir,dirnames,filenames in os.walk('.'):
# Props to Cristian Cliupitu for the better python
if not any(x.endswith('.ENDING') for x in filenames):
print(curdir)
额外 DLC 内容!
find 命令的(大部分)更正版本:
find . -type d \! -exec bash -c 'set nullglob; test -f "{}"/*.ENDING' \; -print
答案3
外壳会扩展*
,但在你的情况下,没有涉及外壳,只有测试命令执行寻找。因此,要测试其存在的文件实际上被命名为*.ENDING
。
相反,你应该使用如下方法:
find . -type d \! -execdir sh -c 'test -e {}/*.ENDING' \; -print
这将导致什扩大*.ENDING
时测试被执行。
答案4
我个人会用 perl 来做
#!/usr/bin/perl
use strict;
use warnings;
use File::Find;
#this sub is called by 'find' on each file it traverses.
sub checkdir {
#skip non directories.
return unless ( -d $File::Find::name );
#glob will return an empty array if no files math - which evaluates as 'false'
if ( not glob ( "$File::Find::name/*.ENDING" ) ) {
print "$File::Find::name does not contain any files that end with '.ENDING'\n";
}
}
#kick off the search on '.' - set a directory path or list of directories to taste.
# - you can specify multiple in a list if you so desire.
find ( \&checkdir, "." );
应该可以解决问题(适用于我的非常简单的测试用例)。