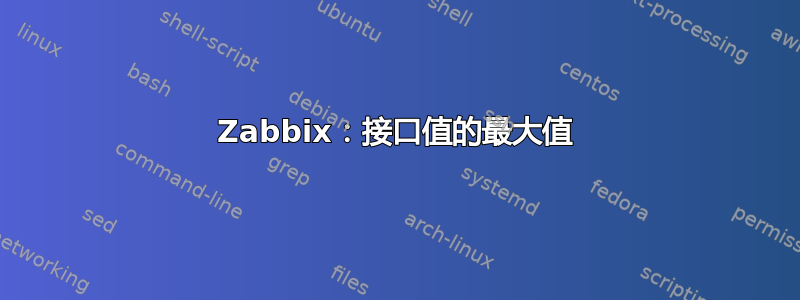
我使用“模板 SMNP 接口”来监控交换机。
它给了我类似的键:ifOutOctets[16]
我想要一个覆盖所有端口的物品:
MaxOutOctets = max(ifOutOctets[*])
我可以在图表中使用它。
我读过了 https://www.zabbix.com/documentation/2.2/manual/config/items/itemtypes/calculated 但我似乎无法正确理解语法。
答案1
不幸的是,您所寻求的功能目前还无法实现,但是有一个功能请求:ZBXNEXT-1829。 要求ZBXNEXT-1420和ZBXNEXT-1521也相关。
答案2
解决方法(其他人可能会发现这很有帮助):
#!/usr/bin/perl
# This program reads off traffic from a switch by SNMP.
# It compares the values with last run and returns the value of the port with the biggest change
#
# Usage:
# snmp_max_io 192.168.1.1
#
# (C) 2014-09-29 Ole Tange
# License: GPLv3
my $switch = shift;
my ($last,$this) = update_cache_file($switch);
my %delta = delta($last,$this);
my $max = max(values %delta);
print "$max\n";
`logger $0 $switch returned $max`;
sub delta {
my ($last,$this) = @_;
# IF-MIB::ifHCOutOctets.45 = Counter64: 262606806485
my %last = map { split /: /,$_ } grep /Counter/, @$last;
my %this = map { split /: /,$_ } grep /Counter/, @$this;
my %delta = map { $_ => undef_as_zero($this{$_}) - undef_as_zero($last{$_}) }
keys %last, keys %this;
return %delta;
}
sub undef_as_zero {
my $a = shift;
return $a ? $a : 0;
}
sub my_dump {
# Returns:
# ascii expression of object if Data::Dump(er) is installed
# error code otherwise
my @dump_this = (@_);
eval "use Data::Dump qw(dump);";
if ($@) {
# Data::Dump not installed
eval "use Data::Dumper;";
if ($@) {
my $err = "Neither Data::Dump nor Data::Dumper is installed\n".
"Not dumping output\n";
print $Global::original_stderr $err;
return $err;
} else {
return Dumper(@dump_this);
}
} else {
# Create a dummy Data::Dump:dump as Hans Schou sometimes has
# it undefined
eval "sub Data::Dump:dump {}";
eval "use Data::Dump qw(dump);";
return (Data::Dump::dump(@dump_this));
}
}
sub update_cache_file {
# Input:
# $switch = DNS name or IP address of switch
my $switch = shift;
my $tmpdir = "/tmp/switch-$ENV{USER}";
my $tmpfile = "$tmpdir/snmp_last_$switch";
mkdir $tmpdir;
chmod 0700, $tmpdir || die;
my @last = `cat $tmpfile 2>/dev/null`;
my @this = snmpget_io($switch);
open(OUT,">",$tmpfile) || die;
print OUT @this;
close OUT;
if(not @last) {
@last = @this;
}
return (\@last,\@this);
}
sub snmpget_io {
# Input:
# $switch = DNS name or IP address of switch
my $switch = shift;
# Requires snmp-mibs-downloader installed
return qx{ bash -c 'snmpget -v 2c -c public $switch IF-MIB::ifHCInOctets.{1..60}; snmpget -v 2c -c public $switch IF-MIB::ifHCOutOctets.{1..60};' }
}
sub max {
# Returns:
# Maximum value of array
my $max;
for (@_) {
# Skip undefs
defined $_ or next;
defined $max or do { $max = $_; next; }; # Set $_ to the first non-undef
$max = ($max > $_) ? $max : $_;
}
return $max;
}