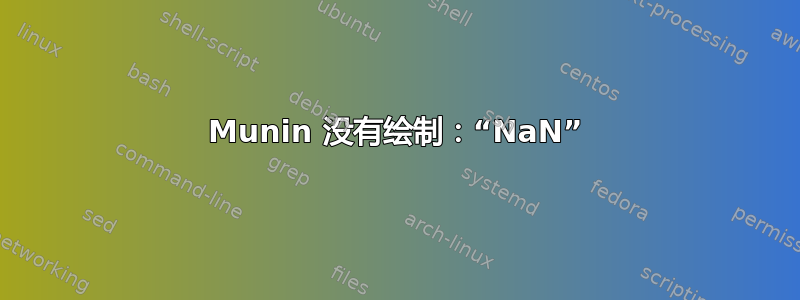
我为 munin 编写了一个插件,它基本上从 .log 文件中提取一些数字并计算这些值的平均值。
当我使用 munin-cron 或直接像这样运行它时:
# munin-run latency_news
latency.value 0.1
# ./latency_news
latency.value 0.1
它运行得很好。但在我的 html munin 页面中,我只看到图表中的所有值都是“Nan”:
(该图片仅供展示,并非我的实际插件)
这是插件的代码:
#!/usr/bin/env php
<?php
class LatencyLog
{
private $fname;
private $fd;
private $pname;
private $pos;
function __construct($page)
{
$this->fname = '/var/log/time_'.$page.'.log';
if (!($this->fd = @fopen($this->fname, 'r')))
{
throw new Exception('Unable to read ' . $this->fname);
}
$this->pname = $this->fname;
$this->pos = 0;
if ($pd = @fopen($this->pname, 'r'))
{
$pos = (int) fgets($pd);
fclose($pd);
fseek($this->fd, 0, SEEK_END);
if ($pos <= ftell($this->fd))
{
$this->pos = $pos;
}
}
fseek($this->fd, $this->pos);
}
function __destruct()
{
$pos = ftell($this->fd);
if ($pos != $this->pos)
{
if (!($pd = @fopen($this->pname, 'w')))
{
throw new Exception('Unable to write ' . $this->pname);
}
fprintf($pd, "%d\n", $pos);
fclose($pd);
}
fclose($this->fd);
}
function get_line()
{
return fgets($this->fd);
}
}
class LatencyMonitor
{
private $query_log;
private $page;
function __construct()
{
$this->page = explode('_', $_SERVER['SCRIPT_FILENAME']);
$this->query_log = new LatencyLog($this->page[1]);
}
function check($what)
{
$queries = 0;
$results = 0;
$times = 0;
while ($line = $this->query_log->get_line())
{
$url = explode('=', $line);
$page = $url[0];
if ($page == $this->page[1])
{
$queries++;
$times += (float) $url[1];
}
}
if ($queries > 0)
$times /= $queries;
echo "$what.value ".str_replace(',', '.', round($times, 2))."\n";
}
}
$tmp = explode('_', $_SERVER['SCRIPT_FILENAME']);
$name = $tmp[1];
$label = 'average time per query';
$what = 'latency';
if (isset($argv[1]))
{
if ($argv[1] == 'config')
{
$title = $name;
echo "graph_title $title\n";
echo "graph_category perfs\n";
echo "graph_vlabel $label\n";
echo "$what.label $what\n";
echo "$what.type GAUGE\n";
echo "$what.min 0\n";
exit;
}
}
$smm = new LatencyMonitor();
$smm->check($what);
?>