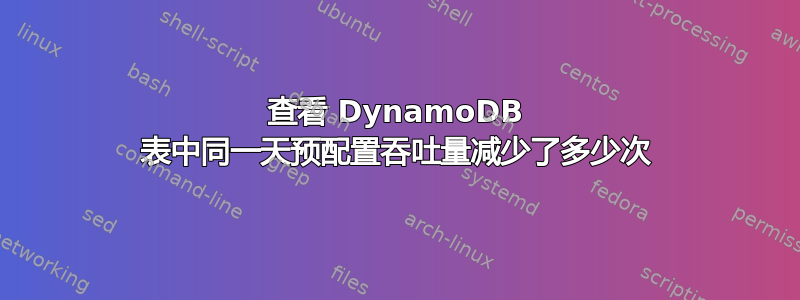
有没有办法查看 DynamoDB 表中同一天(UTC)内预配置吞吐量减少了多少次?
当增加预配置吞吐量时,我总是担心无法在同一天内降低它,因为同一天 (UTC) 内不能将 DynamoDB 表中的预配置吞吐量降低 4 次以上。
监控图的精度似乎不足以查看同一天发生的所有先前的预置吞吐量变化。例如,此表中的预置吞吐量减少了 4 次,但在图表上看不到:
显然,如果我们增加预配置吞吐量但不允许将其减少回来,亚马逊不会给出任何警告。
答案1
答案2
如果这可以帮助任何人,我编写了一个 Python 函数来查看每个 DynamoDB 表在同一天内配置的吞吐量减少了多少次:
import boto
import operator
MY_ACCESS_KEY_ID = 'copy your access key ID here'
MY_SECRET_ACCESS_KEY = 'copy your secret access key here'
def get_number_of_decreases_today():
'''
Scan all DynamoDB tables, and returns a dictionary with table name as key, NumberOfDecreasesToday as value.
'''
dynamodb_conn = boto.connect_dynamodb(aws_access_key_id=MY_ACCESS_KEY_ID, aws_secret_access_key=MY_SECRET_ACCESS_KEY)
table_names = dynamodb_conn.list_tables()
table_number_of_decreases_today = {}
for table_name in table_names:
dynamodb_table_description = dynamodb_conn.describe_table(table_name)
table_number_of_decreases_today[table_name] = dynamodb_table_description['Table']['ProvisionedThroughput']['NumberOfDecreasesToday']
#print('NumberOfDecreasesToday is {0} for table {1}'.format(table_number_of_decreases_today[table_name], table_name))
return table_number_of_decreases_today
def main():
table_number_of_decreases_today = get_number_of_decreases_today()
# Print table names and NumberOfDecreasesToday with alphabetically ordered table names
for key, value in sorted(table_number_of_decreases_today.items(), key=operator.itemgetter(0)):
print('{0}\t{1}'.format(key,value))
# Print table names by ascending NumberOfDecreasesToday
for key, value in sorted(table_number_of_decreases_today.iteritems(), key=lambda (k,v): (v,k)):
print('{0}\t{1}'.format(key, value))
if __name__ == "__main__":
main()
#cProfile.run('main()') # if you want to do some profiling