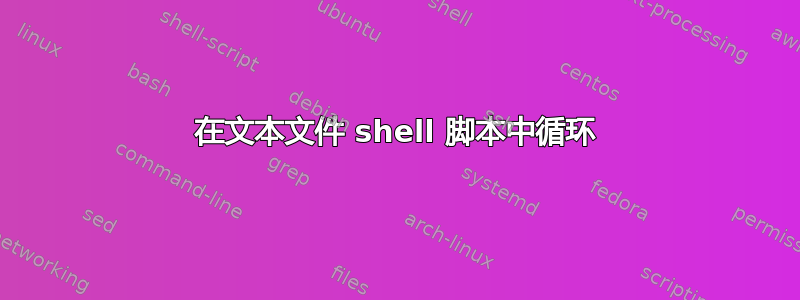
我有一个目录中的文件列表和与另一个目录中的每个文件对应的一组 jpeg。我需要循环所有文件,并为每个文件名确定目标目录。
例如,如果我有三个名为foo.txt
、bar.txt
和baz.txt
in 的文本文件/home/userA/folder/
,则相应的 jpeg 将位于/home/userA/folder2/foo/
、/home/userA/folder2/bar/
和 中/home/userA/folder2/baz/
。
我编写了一个脚本,应该循环遍历所有 txt 文件并获取相应的目标目录,但它给了我一个错误:
bash: /home/userA/folder/File1.txt: syntax error: operand expected (error token is "/home/userA/folder/File1.txt")`
我的脚本:
#!/bin/bash
FILES=/home/userA/folder/*.txt
for i in $FILES
do
str1=$i | cut -d'/' -f5 #to get the name of the file
echo /home/userA/folder2/$i_filename #I want to include the .txt filename in the output to be like this /home/userA/folder2/Text1_filename
done
我怎样才能解决这个问题?
答案1
如果您想要的只是获取文件名并使用它来获取正确的目标目录,您可以执行以下操作:
#!/bin/bash
for i in /home/userA/folder/*.txt
do
## Get the file name
str1="${i##*/}"
## Get the target directory
dir="/home/userA/folder2/${str1%.txt}/"
done
这是使用 shell 的本机字符串操作特征。从 的开头${var##pattern}
删除 的最长匹配项,并从 的结尾删除 的最短匹配项。因此,从文件名中删除直到最后一个(路径)的所有内容,并从其末尾删除字符串。pattern
$var
${var%pattern}
pattern
$var
${i##*/}
/
${i%.txt}
.txt
答案2
使用find
:
#!/bin/bash
path="/home/userA/folder"
find "$path" -maxdepth 1 -type f -name "*.txt" -print0 | while read -d $'\0' file; do
a="$path/$(basename $file)/a_%06.jpg"
echo "$a
done
答案3
您只是忘记了echo
这一行的反引号和字段号
str1=`echo $i | cut -d'/' -f5 `#to get the name of the file
但basename
可能是更好的选择。
str1=`basename $i` #name of the file
像这样
#!/bin/bash
FILES=/home/userA/folder/*.txt
for i in $FILES
do
str1=`basename "$i"` #to get the name of the file
echo $str1
ls -l "`dirname "$i"`/$str1"
done
有关处理 for 循环和名称中带有空格的文件的良好答案,请参阅这个答案
答案4
如果你确实想将其放入变量中,可以使用 bash 数组:
#!/bin/bash
FILES=(/home/userA/folder/*.txt)
for i in "${FILES[@]}" # double qouting pervents extra word splitting
do
bn="$(basename "$i")" # to get the name of the file
a="/home/userA/folder2/$bn/a_%06d.jpg"
done
或者您可以简单地使用for i in /home/userA/folder/*.txt
.