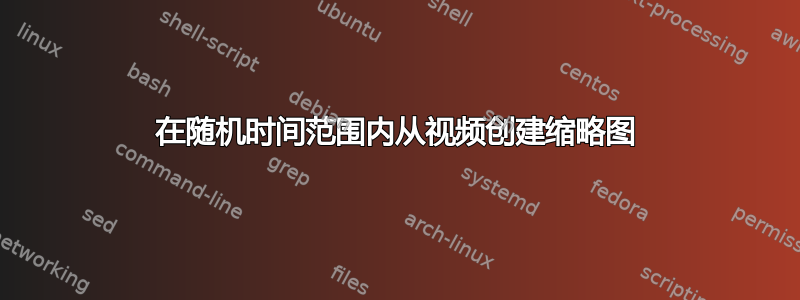
我正在使用这个 bash 脚本从视频生成缩略图:
#!/bin/bash
source_dir="."
output_dir="/output"
input_file_types=(avi wmv flv mkv mpg mp4)
output_file_type="jpg"
r1=$(( ( RANDOM % 10 ) + 5 ))
convert() {
echo "" | ffmpeg -ss 00:"r1":05 -y -i "$1" -an -f image2 -vframes 1 "$output_dir/$2"
}
for input_file_type in "${input_file_types[@]}"
do
find "$source_dir" -name "*.$input_file_type" -print0 | while IFS= read -r -d $'\0' in_file
do
echo "Processing…"
echo ">Input $in_file"
# Replace the file type
out_file=$(echo "$in_file" | sed "s/\(.*\.\)$input_file_type/\1$output_file_type/g")
# The above can be shortened to
# out_file="${in_file%.$input_file_type}.$output_file_type"
echo ">Output $out_file"
# Convert the file
convert "$in_file" "$out_file"
if [ $? != 0 ]
then
echo "$in_file had problems" >> handbrake-errors.log
fi
echo ">Finished $out_file\n\n"
done
done
echo "DONE CONVERTING FILES"
我想要的是计算总视频时间并从随机视频时间生成缩略图。
mediainfo myvideo.mp4 | grep Duration
Duration : 5mn 7s
Duration : 5mn 7s
Duration : 5mn 7s
如何集成grep Duration
到上面的 bash 脚本中,以便我可以根据总视频时间获取随机时间的缩略图?
答案1
尝试以秒为单位获取持续时间,因为这将使一切变得更容易:
您的转换函数最终可能是:
convert() {
# Get duration in milliseconds, then convert to seconds
duration=$(($(mediainfo --Inform="General;%Duration%" "${in_file}" ) / 1000 ))
# Calculate random time
random_time=$(date -u -d @$(shuf -i 0-${duration} -n 1) +"%T")
# Take screenshot
ffmpeg -ss ${random_time} -i "$in_file" -vframes 1 -q:v 2 "$output_dir/$output_file"
}
答案2
这个bash会做
#!/bin/bash
source_dir="."
output_dir="."
input_file_types=(avi wmv flv mkv mpg mp4)
output_file_type="jpg"
convert() {
echo "" | ffmpeg -ss $ss -y -i "$in_file" -an -f image2 -vframes 1 "$output_dir/$out_file"
}
for input_file_types in "${input_file_types[@]}"
do
find "$source_dir" -name "*.$input_file_types" -print0 | while IFS= read -r -d $'\0' in_file
do
echo "Processing…"
echo ">Input "$in_file
# Replace the file type
out_file=$(echo $in_file|sed "s/\(.*\.\)$input_file_types/\1$output_file_type/g")
echo ">Output "$out_file
# get video duration
fulltime=`ffmpeg -i "$in_file" 2>&1 | grep 'Duration' | cut -d ' ' -f 4 | sed s/,//`;
hour=`echo $fulltime | cut -d ':' -f 1`;
minute=`echo $fulltime | cut -d ':' -f 2`;
second=`echo $fulltime | cut -d ':' -f 3 | cut -d '.' -f 1`;
seconds=`expr 3600 \* $hour + 60 \* $minute + $second`;
ss=`expr $seconds / 2`; # from the middle of video
# Convert the file
convert "$in_file" "$out_file"
if [ $? != 0 ]
then
echo "$in_file had problems" >> ffmpeg-errors.log
fi
echo ">Finished "$out_file "\n\n"
done
done
从电影中间创建缩略图。
答案3
视频时长:
DURATION=$(ffmpeg -i "$4" 2>&1 | grep "Duration"| cut -d ' ' -f 4 | sed s/,// | sed 's@\..*@@g' | awk '{ split($1, A, ":"); split(A[3], B, "."); print 3600*A[1] + 60*A[2] + B[1] }')
RES=$(ffmpeg -i "$4" 2>&1 | grep -oP 'Stream .*, \K[0-9]+x[0-9]+')
完整的缩略图生成器: https://github.com/romanwarlock/thumbnails/blob/master/thumbgen.sh