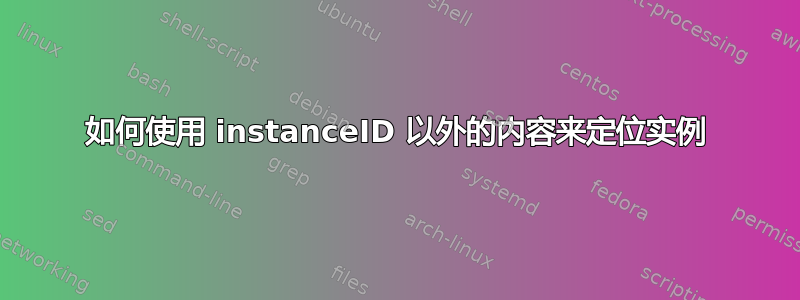
我试图实现的目标是,我有大约 8 个测试实例,我想在下班时停止它们以节省成本。然后当我回到工作岗位时重新启动它们。
我有一个功能可以实现我需要的功能
import boto3
region = 'cn-north-1'
instances = ['i-xxxxxx', 'i-xxxxxxx', 'i-xxxxxx', 'i-xxxxxx', 'i-xxxxxxxx']
ec2 = boto3.client('ec2', region_name=region)
def lambda_handler(event, context):
ec2.stop_instances(InstanceIds=instances)
print('stopped your instances: ' + str(instances))
问题是我在所有实例上都有不可变的部署策略,因此它instances
会不断变化,我必须每天更新该功能。
有没有什么方法可以通过标签或其他更通用的方法进行定位,这样我就不必每天更新功能了?
我查看了文档,但还是不太清楚 https://boto3.amazonaws.com/v1/documentation/api/latest/reference/services/ec2.html
答案1
大多数人应该使用实例调度程序,但在中国地区却不能使用。
替代解决方案
互联网上有几十个示例可以帮助你实现这一点。其中之一是这里。基本上,您无需对实例 ID 进行硬编码,而是使用 boto3 API 通过标签查找实例。
此功能还允许您使用标签更改开始/停止时间。您可以截取此代码的部分内容并修改您的代码(来自这里我认为)来将其纳入其中。
import boto3
import time
##
# First function will try to filter for EC2 instances that contain a tag named `Scheduled` which is set to `True`
# If that condition is meet function will compare current time (H:M) to a value of the additional tags which defines the trigger `ScheduleStop` or `ScheduleStart`.
# Value of the `ScheduleStop` or `ScheduleStart` must be in the following format `H:M` - example `09:00`
#
# In order to trigger this function make sure to setup CloudWatch event which will be executed every minute.
# Following Lambda Function needs a role with permission to start and stop EC2 instances and writhe to CloudWatch logs.
#
# Example EC2 Instance tags:
#
# Scheduled : True
# ScheduleStart : 06:00
# ScheduleStop : 18:00
##
#define boto3 the connection
ec2 = boto3.resource('ec2')
def lambda_handler(event, context):
# Get current time in format H:M
current_time = time.strftime("%H:%M")
# Find all the instances that are tagged with Scheduled:True
filters = [{
'Name': 'tag:Scheduled',
'Values': ['True']
}
]
# Search all the instances which contains scheduled filter
instances = ec2.instances.filter(Filters=filters)
stopInstances = []
startInstances = []
# Locate all instances that are tagged to start or stop.
for instance in instances:
for tag in instance.tags:
if tag['Key'] == 'ScheduleStop':
if tag['Value'] == current_time:
stopInstances.append(instance.id)
pass
pass
if tag['Key'] == 'ScheduleStart':
if tag['Value'] == current_time:
startInstances.append(instance.id)
pass
pass
pass
pass
print current_time
# shut down all instances tagged to stop.
if len(stopInstances) > 0:
# perform the shutdown
stop = ec2.instances.filter(InstanceIds=stopInstances).stop()
print stop
else:
print "No instances to shutdown."
# start instances tagged to stop.
if len(startInstances) > 0:
# perform the start
start = ec2.instances.filter(InstanceIds=startInstances).start()
print start
else:
print "No instances to start."
标准溶液
对于大多数人来说这是最好的解决方案。
使用AWS 实例调度程序, 按照本教程。它根据您定义的时间表,基于标签启动和停止实例。
我不会在这里复制粘贴文章,因为信息偶尔会发生变化,并且 AWS 会很好地保持其文档更新。