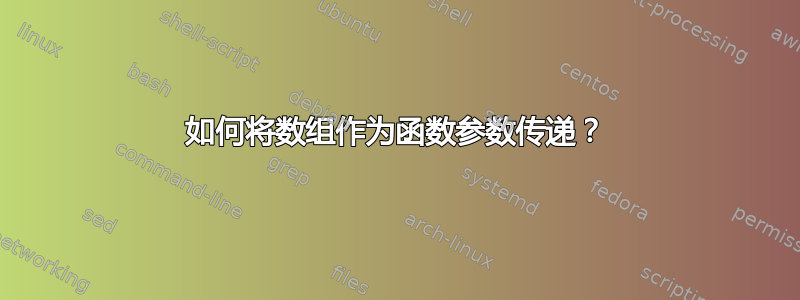
尝试了一段时间,尝试将数组作为参数传递,但不管用。我尝试了以下方法:
#! /bin/bash
function copyFiles{
arr="$1"
for i in "${arr[@]}";
do
echo "$i"
done
}
array=("one" "two" "three")
copyFiles $array
如果有解释的话答案就更好了。
编辑:基本上,我最终会从另一个脚本文件调用该函数。如果可能的话,请解释一下限制。
答案1
扩展没有索引的数组只会给出第一个元素,使用
copyFiles "${array[@]}"
代替
copyFiles $array
使用 she-bang
#!/bin/bash
使用正确的函数语法
有效变体是
function copyFiles {…} function copyFiles(){…} function copyFiles() {…}
代替
function copyFiles{…}
使用正确的语法获取数组参数
arr=("$@")
代替
arr="$1"
所以
#!/bin/bash
function copyFiles() {
arr=("$@")
for i in "${arr[@]}";
do
echo "$i"
done
}
array=("one 1" "two 2" "three 3")
copyFiles "${array[@]}"
输出是(我的脚本有名称foo
)
$ ./foo
one 1
two 2
three 3
答案2
如果你想传递一个或多个参数和一个数组,我建议对@AB 数组的脚本进行这样的更改
应该是最后的论点和只有一个可以传递数组
#!/bin/bash
function copyFiles() {
local msg="$1" # Save first argument in a variable
shift # Shift all arguments to the left (original $1 gets lost)
local arr=("$@") # Rebuild the array with rest of arguments
for i in "${arr[@]}";
do
echo "$msg $i"
done
}
array=("one" "two" "three")
copyFiles "Copying" "${array[@]}"
输出:
$ ./foo
Copying one
Copying two
Copying three
答案3
您还可以将数组作为引用传递。即:
#!/bin/bash
function copyFiles {
local -n arr=$1
for i in "${arr[@]}"
do
echo "$i"
done
}
array=("one" "two" "three")
copyFiles array
但请注意,对 arr 的任何修改都将对数组进行修改。
答案4
下面是一个稍微大一点的例子。有关解释,请参阅代码中的注释。
#!/bin/bash -u
# ==============================================================================
# Description
# -----------
# Show the content of an array by displaying each element separated by a
# vertical bar (|).
#
# Arg Description
# --- -----------
# 1 The array
# ==============================================================================
show_array()
{
declare -a arr=("${@}")
declare -i len=${#arr[@]}
# Show passed array
for ((n = 0; n < len; n++))
do
echo -en "|${arr[$n]}"
done
echo "|"
}
# ==============================================================================
# Description
# -----------
# This function takes two arrays as arguments together with their sizes and a
# name of an array which should be created and returned from this function.
#
# Arg Description
# --- -----------
# 1 Length of first array
# 2 First array
# 3 Length of second array
# 4 Second array
# 5 Name of returned array
# ==============================================================================
array_demo()
{
declare -a argv=("${@}") # All arguments in one big array
declare -i len_1=${argv[0]} # Length of first array passad
declare -a arr_1=("${argv[@]:1:$len_1}") # First array
declare -i len_2=${argv[(len_1 + 1)]} # Length of second array passad
declare -a arr_2=("${argv[@]:(len_1 + 2):$len_2}") # Second array
declare -i totlen=${#argv[@]} # Length of argv array (len_1+len_2+2)
declare __ret_array_name=${argv[(totlen - 1)]} # Name of array to be returned
# Show passed arrays
echo -en "Array 1: "; show_array "${arr_1[@]}"
echo -en "Array 2: "; show_array "${arr_2[@]}"
# Create array to be returned with given name (by concatenating passed arrays in opposite order)
eval ${__ret_array_name}='("${arr_2[@]}" "${arr_1[@]}")'
}
########################
##### Demo program #####
########################
declare -a array_1=(Only 1 word @ the time) # 6 elements
declare -a array_2=("Space separated words," sometimes using "string paretheses") # 4 elements
declare -a my_out # Will contain output from array_demo()
# A: Length of array_1
# B: First array, not necessary with string parentheses here
# C: Length of array_2
# D: Second array, necessary with string parentheses here
# E: Name of array that should be returned from function.
# A B C D E
array_demo ${#array_1[@]} ${array_1[@]} ${#array_2[@]} "${array_2[@]}" my_out
# Show that array_demo really returned specified array in my_out:
echo -en "Returns: "; show_array "${my_out[@]}"