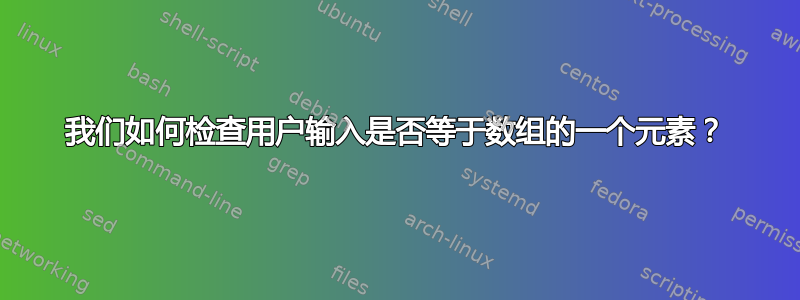
假设我们有一个这样的数组:
declare -a array=( "one" "two" "three" "four" "five" )
for i in "${all[@]}"; do
echo "$i"
done
我们可以提供用户的输入,如下所示:
read -p "Enter a number: " number
我需要一些东西来检查用户输入是否与此数组的某个元素匹配。如果不匹配,则应运行此代码:
echo "Try again: "
应重复此过程,直到用户输入与数组元素之一匹配。
答案1
您可以将数组变成关联数组。
#!/bin/bash
declare -A array=([one]=1 [two]=1 [tree]=1 [four]=1 [five]=1)
m="Guess? "
while true; do
read -r -p "$m" i
[[ ${array[$i]} == 1 ]] && break || m="Guess again? "
done
echo "Good guess!"
答案2
您无需循环遍历数组来检查某个值是否属于它。此外,在这种情况下,我将使用函数的递归调用而不是另一个循环。正如我所理解的,您正在搜索类似这样的内容:
#!/bin/bash
array=( "one" "two" "three" "four" "five" )
function get_input() {
read -p "${1}: " number
if [[ " ${array[*]} " == *" ${number} "* ]]
then
echo 'true';
else
get_input 'Try again' # a recursive call of the function
fi
}
get_input 'Enter a number' # the initial call of the function
随着[[
测试命令我们寻找==
一个字符串(左侧)和一个正则表达式(右侧)之间的精确匹配。
该表达式
" ${array[*]} "
将数组扩展为一个字符串,由两个空格包围,因此每个元素(甚至第一个和最后一个)都会被空格包围——尝试echo '^'" ${array[*]} "'$'
。正则表达式
*" ${number} "*
将匹配由变量的值组成的任何字符串$number
,由空格包围,由任何其他字符包围*
。
我们可以用其他工具来执行这样的测试grep
(使用抑制 stdout -q
,并搜索精确匹配\<...\>
)。这是一个有趣的版本:
#!/bin/bash
array=( "one" "two" "three" "four" "five" )
function get_input() {
read -p "${1}: " nm
echo "${array[*]}" | grep -q "\<${nm}\>" && echo 'true' || get_input 'Try again'
}
get_input "Enter a number"
如果我们想调用数组中每个元素对应的数字,而不是插入像''one''或''two''这样的字眼,我们该怎么做?例如:输入数字:2
#!/bin/bash
array=( "one" "two" "three" "four" "five" )
# Get the lenght of the array
array_lenght="${#array[*]}"
function get_input() {
read -p "${1}: " number
# Test whether the variable $number has a value &and&
# test whether as integer this value is less or equal to the $array_lenght
if [[ ! -z ${number} ]] && (( number <= array_lenght && number > 0 ))
then
# Outpit the value of the cerain array element
# Note the first array element has number 0
echo "${array[$(( number - 1 ))]}"
else
get_input 'Try again'
fi
}
get_input 'Enter an integer number'
答案3
可能所有其他答案都比这个更有效,但我认为这对初学者更友好:
declare -a all=( "one" "two" "three" "four" "five" )
read -p "Enter a number: " number
while true; do
for i in "${all[@]}"; do
if [[ "$number" = "$i" ]]; then
exit 0
fi
done
read -p "Try again: " number
done
答案4
#!/bin/bash
declare -a array=("one" "two" "three" "four" "five")
number="wrong"
while [[ ! "${array[@]}" =~ "${number}" ]]; do
[[ "${number}" != "wrong" ]] && echo "Incorrect"
read -p "Insert number: " number
done