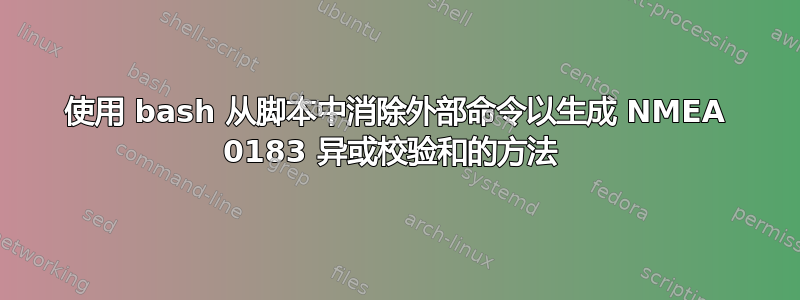
我最近需要使用 bash 生成和验证 NMEA 0183 校验和,但我找不到太多可以完成我所需要的内容。
NMEA 0183 句子以 $ 开头,以 * 结尾,以及两个字符,这两个字符是 $ 和 * 之间所有字节的十六进制异或。例子:
$INGGA,230501.547,2118.97946,N,15752.60495,W,2,08,1.1,5.17,M,,,0,0001*02
该实用程序会将字符串转换为十六进制并对其进行异或。它可用于验证已经存在的校验和,或为您正在生成的 NMEA 句子末尾生成校验和(它会从您提供的字符串中删除 $ 和 *..)。
#!/bin/bash
# =========================================================
# Reads a NMEA 0183 sentence and calculates the proper
# XOR checksum for the end.
# Will accept a string with or without a checksum on
# the end or $ on the front and calculate what the checksum
# should be.
# Sentence can be read as an argument but must be single quoted
# or preceded by a \ or the shell will try to interpret the
# talker as a variable and the result will be incorrect.
# Examples:
# xor '$INHDT,207.7,T*27'
# xor \$INHDT,207.7,T*27
# xor INHDT,207.7,T
# If run with no arguments, will prompt user for data. No
# quotes or backslash is needed then.
# Depends: xxd sed
# ===T.Young 09/2016=======================================
set -o pipefail
set -o errexit
set -o nounset
# Functions
# =========
depcheck() { # Checks that necessary external commands are present
# and executable
local DEPENDS="sed xxd"
for PROG in $DEPENDS; do
[[ -x "$(command -v $PROG)" ]] || {
echo "$PROG MISSING! Exiting."
exit 0
}
done
}
x_or() { # Here is where the magic happens
# The next two lines strip out $ characters, or an
# * and anything after it (checksum)
HEAD="${SENTENCE%\**}"
TAIL="${HEAD//\$}"
# Convert ASCII string into hex and read into an array.
# Each element in the array gets preceded by "0x"
HEXVAL="$(xxd -pu <<< ${TAIL})"
HEXARRAY=($(printf '%s' "${HEXVAL%0a}" | sed -e 's/../0x& /g'))
# Loop through the array and do the xor, initially start $XOR at 0
for (( x=0; x<"${#HEXARRAY[@]}"; x++ )); do
XOR=0x$(printf '%02x' "$(( ${XOR:-0} ^ ${HEXARRAY[$x]} ))")
done
# Strip off the 0x from the result
CLEAN=${XOR#0x}
printf '%s\n' "${CLEAN^^}"
}
main() {
case "${1:-}" in
"") # No input specified, read from stdin
depcheck
read -r SENTENCE
x_or
;;
*) # Input was provided, use that
depcheck
SENTENCE="$1"
x_or
;;
esac
}
# Main
# ====
main "$@"
当编写 shell 脚本时,我总是试图找到方法来消除外部程序的使用,甚至是像 sed 或 xxd 这样常见的程序。如果有人知道仅使用 shell 内置函数执行上述操作的方法,请插话。
更新:这是一个考虑佐藤方法的新函数。它允许完全消除外部程序调用以及上面关联的 depcheck 函数。
x_or() { # Create a hex XOR checksum of all the bytes
# Clean the line of $ character and anything before it
TAIL="${SENTENCE##*$}"
HEAD=${TAIL%\**}
LEN=${#HEAD}
# Loop through the string and do the xor
# initially start $XOR at 0
XOR=0
for (( x=0; x<$LEN; x++ )); do
(( XOR^=$(printf '%d' "'${HEAD:$x:1}'") ))
done
printf '%02X\n' "${XOR}"
}
使用“LC_CTYPE=C”调用该函数。这里可能还可以做更多的事情,但这相当简洁。
答案1
就我个人而言,我会这样做:
#! /usr/bin/env bash
log() {
{
printf '%s: ' "${0##*/}"
printf "$@"
printf '\n'
} >&2
}
cksum() {
tot=${#1}
let len=tot-4
let res=0
while [ $len -gt 0 ]; do
let res^=$( LC_CTYPE=C printf '%d' "'${1:$len:1}'" )
let len--
done
let ptr=tot-2
if [ x"$( printf '%s' "${1:$ptr}" | tr a-f A-F )" != x"$( printf '%02X' $res )" ]; then
log '%s: invalid checksum (found %02X)' "$1" $res
fi
}
check () {
if expr "$2" : '\$.*\*[0-9a-fA-F][0-9a-fA-F]$' >/dev/null; then
cksum "$2"
else
log 'invalid input on line %d: %s' "$1" "$2"
fi
}
let cnt=0
if [ $# -ne 0 ]; then
while [ $# -gt 0 ]; do
let cnt++
check $cnt "$1"
shift
done
else
while read -r str; do
let cnt++
check $cnt "$str"
done
fi
shebang 线声称bash
,但它应该仍然适用于ksh93r
和zsh
。不依赖于xxd
.也没有声称是可遵循的脚本风格的示例。 :)