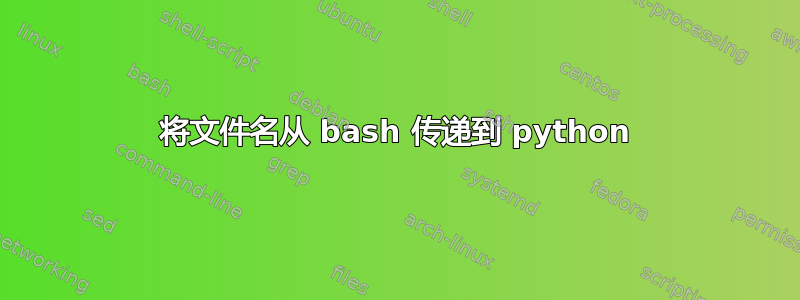
假设我的文件夹中有 1000 个具有随机名称的 csv 文件test
。并且我有一个 python 脚本script.py
。我想编写一个 bash 脚本,它将传递文件夹中每个文件的名称然后script.py
运行它,因此 bash 脚本应该运行script.py
1000 次,每次使用不同的文件名,例如,第一次运行:
with open('records.csv','r') as in_file
下一轮
with open('vertigo.csv','r') as in_file
我知道我可以用 python 来做这件事,而不需要像这样编写 bash 脚本
import glob
for filename in glob.glob('*.csv'):
# script here
但我正在做一些测试,因此我想在 bash 中进行
答案1
使用sys.argv[1]
以便从参数中获取文件名:
import sys
with open(sys.argv[1],'r') as in_file
然后,您可以使用各种方法将文件名作为参数传递。例如,使用find
:
find test/ -type f -name '*.csv' -exec /path/to/script.py {} \;
答案2
替代方法sys.argv
是使用argparse
。如果您需要更多命令行参数解析,这将非常有用。
#!/usr/bin/env python2
import argparse
def main(files):
for f in files:
print "Would do something with", f
if __name__ == "__main__":
parser = argparse.ArgumentParser()
parser.add_argument('file', nargs='+', help='path to the file')
args_namespace = parser.parse_args()
args = vars(args_namespace)['file']
main(args)
使用 Bash 扩展我们的文件参数列表来运行它:
$ ./script.py *.csv
Would do something with file1.csv
Would do something with file2.csv
Would do something with file3.csv
并免费提供内置帮助:
$ ./script.py --help
usage: script.py [-h] file [file ...]
positional arguments:
file path to the file
optional arguments:
-h, --help show this help message and exit
答案3
您必须在 Python 脚本中检索文件名才能使其正常工作:
#!/bin/bash
for f in *.csv; do
python script.py "$f"
done
一句话:
for f in *.csv; do python script.py "$f"; done
答案4
使用sys.argv
as 列表,提供所有单独的文件作为参数,并让您的 shell(例如 Bash)为您扩展它。
script.py
好像:
#!/usr/bin/env python2
import sys
def main(files):
for f in files:
print "Would do something with", f
if __name__ == "__main__":
files = sys.argv[1:]: # slices off the first argument (executable itself)
main(files)
文件树如下:
.
├── file1.csv
├── file2.csv
├── file3.csv
└── script.py
0 directories, 4 files
然后使其可执行:
chmod +x script.py
使用 shell 扩展的参数运行它:
./script.py *.csv
输出:
Would do something with file1.csv
Would do something with file2.csv
Would do something with file3.csv