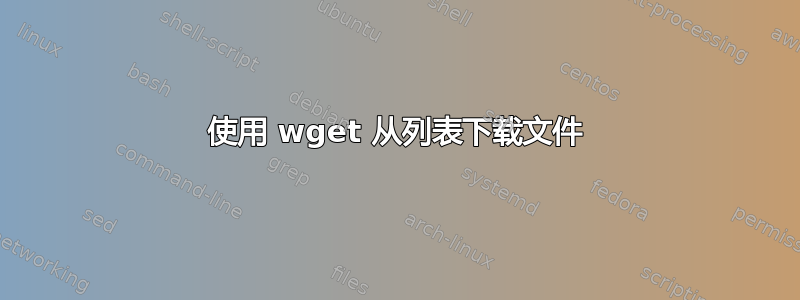
我有一个包含如下下载链接的文件:
Google.com/image2
Google.com/image3
Google.com/image4
Google.com/image5
Google.com/image6
我想使用脚本下载所有这些文件。如果名称以“s”开头,则将此文件下载到目录s
;如果名称以“b”开头,则将其移动到b
目录...
答案1
下载所有文件,然后使用 shell globs 移动它们:
#!/bin/bash
wget -i /path/to/download_list
mv s* ./s/
mv b* ./b/
-i
:从本地或外部文件读取 URL。
您可能会收到警告:
mv: cannot move 's' to a subdirectory of itself.
没关系,你可以忽略它,或者改用find
:
#!/bin/bash
wget -i /path/to/download_list
find -maxdepth 1 -iname "s*" -type f -exec mv "{}" ./s \;
find -maxdepth 1 -iname "b*" -type f -exec mv "{}" ./b \;
使用for
循环可以在所有字母上运行,脚本名称为script.sh
:
#!/bin/bash
wget -i /path/to/download_list
mkdir -p {a..z}
for l in {a..z};
do
find -maxdepth 1 -type f -iname "${l}*" -not -iname script.sh -exec mv "{}" "./${l}" \;
done
答案2
对@Ravexina 的精彩回答进行补充。
无循环的解决方案:
wget -i /path/to/download_list
mkdir -p {a..z}
# mv the files with rename tool
rename 's/^((.).+)$/$2\/$1/' *
# clean up empty directories
find . -maxdepth 1 -name '[a-z]' -type d -empty -delete
答案3
直接下载到所需文件夹的解决方案:
# expand file to list and iterate
for path in $(<"/path/to/download_list"); do
# get file part of path
name=$(basename "$path")
# use first character of name as dir
dir=${name:0:1}
# create dir is not exist
mkdir -p "$dir"
# download path directly to dir
wget "$path" -P "$dir"
done