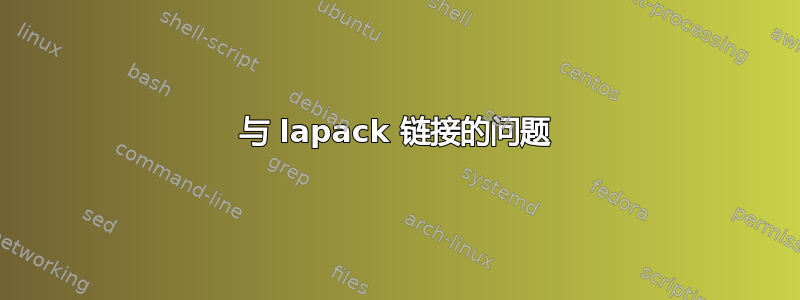
我在新的 ubuntu 机器上使用 lapack 时遇到问题(编辑:Ubuntu 20.04.5 LTS)。我安装liblapack-dev
并libblas-dev
使用了apt-get
。我使用了以下代码,该代码在不同的机器上正常运行。
#include <stdlib.h>
#include <stdio.h>
/* DGESV prototype */
extern void dgesv( int* n, int* nrhs, double* a, int* lda, int* ipiv,
double* b, int* ldb, int* info );
/* Auxiliary routines prototypes */
extern void print_matrix( char* desc, int m, int n, double* a, int lda );
extern void print_int_vector( char* desc, int n, int* a );
/* Parameters */
#define N 5
#define NRHS 3
#define LDA N
#define LDB N
/* Main program */
int main() {
/* Locals */
int n = N, nrhs = NRHS, lda = LDA, ldb = LDB, info;
/* Local arrays */
int ipiv[N];
double a[LDA*N] = {
6.80, -2.11, 5.66, 5.97, 8.23,
-6.05, -3.30, 5.36, -4.44, 1.08,
-0.45, 2.58, -2.70, 0.27, 9.04,
8.32, 2.71, 4.35, -7.17, 2.14,
-9.67, -5.14, -7.26, 6.08, -6.87
};
double b[LDB*NRHS] = {
4.02, 6.19, -8.22, -7.57, -3.03,
-1.56, 4.00, -8.67, 1.75, 2.86,
9.81, -4.09, -4.57, -8.61, 8.99
};
/* Executable statements */
printf( " DGESV Example Program Results\n" );
/* Solve the equations A*X = B */
dgesv( &n, &nrhs, a, &lda, ipiv, b, &ldb, &info );
/* Check for the exact singularity */
if( info > 0 ) {
printf( "The diagonal element of the triangular factor of A,\n" );
printf( "U(%i,%i) is zero, so that A is singular;\n", info, info );
printf( "the solution could not be computed.\n" );
exit( 1 );
}
/* Print solution */
print_matrix( "Solution", n, nrhs, b, ldb );
/* Print details of LU factorization */
print_matrix( "Details of LU factorization", n, n, a, lda );
/* Print pivot indices */
print_int_vector( "Pivot indices", n, ipiv );
exit( 0 );
} /* End of DGESV Example */
/* Auxiliary routine: printing a matrix */
void print_matrix( char* desc, int m, int n, double* a, int lda ) {
int i, j;
printf( "\n %s\n", desc );
for( i = 0; i < m; i++ ) {
for( j = 0; j < n; j++ ) printf( " %6.2f", a[i+j*lda] );
printf( "\n" );
}
}
/* Auxiliary routine: printing a vector of integers */
void print_int_vector( char* desc, int n, int* a ) {
int j;
printf( "\n %s\n", desc );
for( j = 0; j < n; j++ ) printf( " %6i", a[j] );
printf( "\n" );
}
但使用以下命令进行编译:
gcc -Wall test.c -lm -lblas -llapack
导致以下问题:
/usr/bin/ld: /tmp/ccTfUyXO.o: in function `main':
test.c:(.text+0x2e3): undefined reference to `dgesv'
collect2: error: ld returned 1 exit status
我在使用不同 lapack 例程的其他更复杂的代码中遇到了完全相同的问题。
知道什么可能导致问题吗?我还尝试在 -llapack 之后添加包含 lapack 文件的目录的 -L 标志,但没有帮助。
答案1
问题似乎是,dgesv
尽管Fortran函数,它在 C/C++ API 中暴露为dgesv_
。因此
$ gcc -Wall test.c -lm -lblas -llapack
/usr/bin/ld: /tmp/ccp4Lcye.o: in function `main':
test.c:(.text+0x2e3): undefined reference to `dgesv'
collect2: error: ld returned 1 exit status
失败;
$ sed -i 's/dgesv/&_/' test.c
$
$ gcc -Wall test.c -lm -lblas -llapack; echo $?
0
成功,并且生成的可执行文件成功运行:
$ ./a.out
DGESV Example Program Results
Solution
-0.80 -0.39 0.96
-0.70 -0.55 0.22
0.59 0.84 1.90
1.32 -0.10 5.36
0.57 0.11 4.04
Details of LU factorization
8.23 1.08 9.04 2.14 -6.87
0.83 -6.94 -7.92 6.55 -3.99
0.69 -0.67 -14.18 7.24 -5.19
0.73 0.75 0.02 -13.82 14.19
-0.26 0.44 -0.59 -0.34 -3.43
Pivot indices
5 5 3 4 5
或者,您可以考虑安装该lapacke-dev
包并使用LAPACKE_dgesv
。
如需进一步阅读,请参阅在 C/C++ 中使用 BLAS 和 LAPACK。