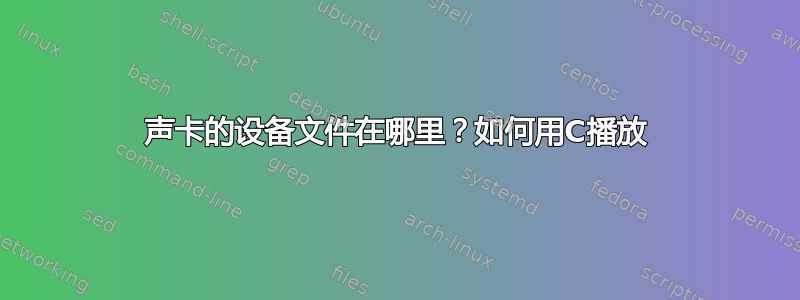
在 中/dev
,我只找到/dev/console
,而不是/dev/dsp
,/dev/audio
等,这是朋友告诉我的Linux中声卡的设备文件。
现在,我想在 Ubuntu 14.04 中使用 C 播放音乐并更改音量。这是我的程序,但我失败了。我想知道如何以正确的方式控制声音设备。
#include <fcntl.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/ioctl.h>
#include <sys/types.h>
#include <linux/kd.h>
/* set default value */
#define DEFAULT_FREQ 440 /*set freq */
#define DEFAULT_LENGTH 1000 /*200 ms*/
#define DEFAULT_REPS 1 /* no repeat by default */
#define DEFAULT_DELAY 100 /* by ms*/
/* define a struct to save data*/
typedef struct {
int freq; /* Hz */
int length; /* duration */
int reps; /* repeat times */
int delay; /* the interval */
} beep_parms_t;
/* print the help message and exit */
void usage_bail ( const char *executable_name ) {
printf ( "Usage: \n \t%s [-f frequency] [-l length] [-r reps] [-d delay] \n ",
executable_name );
exit(1);
}
/* analysis the args, the meanings are:
* "-f < the freq by Hz >"
* "-l < duration by ms >"
* "-r < repeat times >"
* "-d < the interval by ms >"
*/
void parse_command_line(char **argv, beep_parms_t *result) {
char *arg0 = *(argv++);
while ( *argv ) {
if ( !strcmp( *argv,"-f" )) { /*freq*/
int freq = atoi ( *( ++argv ) );
if ( ( freq <= 0 ) || ( freq > 10000 ) ) {
fprintf ( stderr, "Bad parameter: frequency must be from 1..10000\n" );
exit (1) ;
} else {
result->freq = freq;
argv++;
}
} else if ( ! strcmp ( *argv, "-l" ) ) { /*duration*/
int length = atoi ( *(++argv ) );
if (length < 0) {
fprintf(stderr, "Bad parameter: length must be >= 0\n");
exit(1);
} else {
result->length = length;
argv++;
}
} else if (!strcmp(*argv, "-r")) { /*repeat times*/
int reps = atoi(*(++argv));
if (reps < 0) {
fprintf(stderr, "Bad parameter: reps must be >= 0\n");
exit(1);
} else {
result->reps = reps;
argv++;
}
} else if (!strcmp(*argv, "-d")) { /* delay */
int delay = atoi(*(++argv));
if (delay < 0) {
fprintf(stderr, "Bad parameter: delay must be >= 0\n");
exit(1);
} else {
result->delay = delay;
argv++;
}
} else {
fprintf(stderr, "Bad parameter: %s\n", *argv);
usage_bail(arg0);
}
}
}
int main(int argc, char **argv) {
int console_fd;
int i; /* loop counter */
/* default value */
beep_parms_t parms = {DEFAULT_FREQ, DEFAULT_LENGTH, DEFAULT_REPS,
DEFAULT_DELAY};
/* analysis the args */
parse_command_line(argv, &parms);
printf("after param\n");
/* open the device file*/
if ( ( console_fd = open ( "/dev/console", O_WRONLY ) ) == -1 ) {
fprintf(stderr, "Failed to open console.\n");
perror("open");
exit(1);
}
/* start to play */
for (i = 0; i < parms.reps; i++) {
int magical_fairy_number = 1190000/parms.freq;
ioctl(console_fd, KIOCSOUND, magical_fairy_number); /* start */
usleep(1000*parms.length); /*wait... */
ioctl(console_fd, KIOCSOUND, 0); /* stop */
usleep(1000*parms.delay); /* wait... */
} /* repeat */
return EXIT_SUCCESS;
}