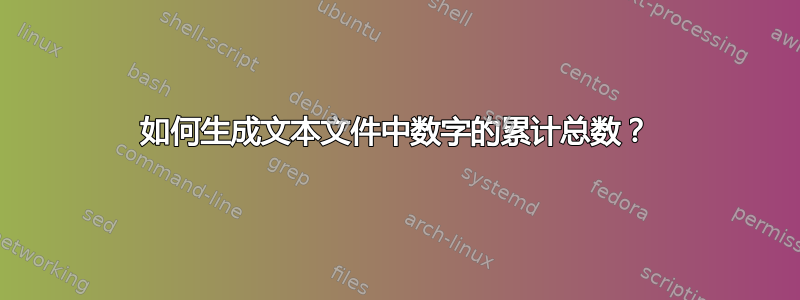
我有一个包含 200 万行的文本文件。每行都有一个正整数。我正在尝试形成频率表之类的东西。
输入文件:
3
4
5
8
输出应该是:
3
7
12
20
我该如何做呢?
答案1
和awk
:
awk '{total += $0; $0 = total}1'
$0
是当前行。因此,对于每一行,我将其添加到total
,将该行设置为新的total
,然后尾随1
是 awk 快捷方式 - 它为每个真条件打印当前行,并1
作为条件评估为真。
答案2
在 Python 脚本中:
#!/usr/bin/env python3
import sys
f = sys.argv[1]; out = sys.argv[2]
n = 0
with open(out, "wt") as wr:
with open(f) as read:
for l in read:
n = n + int(l); wr.write(str(n)+"\n")
使用
- 将脚本复制到一个空文件中,另存为
add_last.py
使用源文件和目标输出文件作为参数运行它:
python3 /path/to/add_last.py <input_file> <output_file>
解释
该代码相当易读,但详细地说:
打开输出文件以写入结果
with open(out, "wt") as wr:
打开输入文件进行读取每行
with open(f) as read: for l in read:
读出各行,并将新行的值添加到总数中:
n = n + int(l)
将结果写入输出文件:
wr.write(str(n)+"\n")
答案3
只是为了好玩
$ sed 'a+p' file | dc -e0 -
3
7
12
20
这有效A附加+p
到输入的每一行,然后将结果传递给dc
计算器
+ Pops two values off the stack, adds them, and pushes the result.
The precision of the result is determined only by the values of
the arguments, and is enough to be exact.
然后
p Prints the value on the top of the stack, without altering the
stack. A newline is printed after the value.
该-e0
参数被推送到堆栈0
上dc
以初始化总和。
答案4
要打印标准输入中给出的整数的部分和(每行一个):
#!/usr/bin/env python3
import sys
partial_sum = 0
for n in map(int, sys.stdin):
partial_sum += n
print(partial_sum)
如果由于某种原因命令太慢;您可以使用 C 程序:
#include <stdint.h>
#include <ctype.h>
#include <stdio.h>
int main(void)
{
uintmax_t cumsum = 0, n = 0;
for (int c = EOF; (c = getchar()) != EOF; ) {
if (isdigit(c))
n = n * 10 + (c - '0');
else if (n) { // complete number
cumsum += n;
printf("%ju\n", cumsum);
n = 0;
}
}
if (n)
printf("%ju\n", cumsum + n);
return feof(stdin) ? 0 : 1;
}
要构建并运行它,请输入:
$ cc cumsum.c -o cumsum
$ ./cumsum < input > output
UINTMAX_MAX
是18446744073709551615
。
对于生成的输入文件,C 代码比我的机器上的 awk 命令快几倍:
#!/usr/bin/env python3
import numpy.random
print(*numpy.random.random_integers(100, size=2000000), sep='\n')