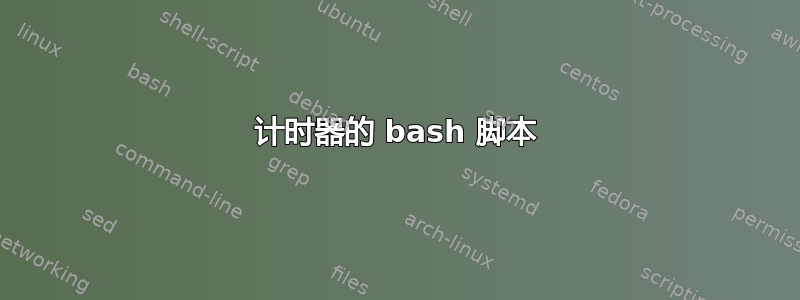
我想编写一个 bash 脚本来运行一个 30 秒的计时器,30 秒后,它会在接下来的 30 秒内保持,然后该计时器会在接下来的 30 秒内启动并继续执行。
for ((i=30; i>0; i--)); do
sleep 1 &
print "$i \r"
wait
done
使用上面的代码,我可以让计时器运行 30 秒,但我需要保持接下来的 30 秒,然后再次运行接下来的 30 秒的计时器。
我怎样才能做到这一点?
我可以用这个代码在 java 中做同样的事情
import java.util.Timer;
import java.util.TimerTask;
public class TestClass {
static int count = 0;
public static void main(String[] args) {
final TestClass test = new TestClass();
Timer timer = new Timer();
timer.schedule(new TimerTask() {
@Override
public void run() {
test.doStuff();
}
}, 0, 1000);
}
public void doStuff() {
if (count == 30) {
try {
Thread.sleep(30000);
count = 0;
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.println(++count);
}
}
答案1
将循环包装成无限循环(IE一个while
无条件的循环),并sleep 30
在这个外层循环中添加一个。
while :
do
### < your
### timer
### here >
sleep 30
done
我建议您也删除该wait
指令和&
来自“ sleep 1 &
”的。另外,print
这不是写入终端的正确程序,请改用echo
。
while :
do
for ((i=30; i>0; i--))
do
sleep 1
echo -n "$i "
done
sleep 30
echo ""
done
请注意,此计时器并不准确,因为评估循环指令需要花费(少量但不为零)时间。使用以下解决方案date
会更好:例如,参见此处的倒计时函数:https://superuser.com/a/611582。
答案2
我认为你正在寻找这样的东西:
#!/bin/bash
## Infinite loop
while :; do
## Run a sleep command for 30 seconds in the background
sleep 30 &
## $! is the PID of the last backgrounded process, so of the sleep.
## Wait for it to finish.
c=30
while [[ -e /proc/$! ]]; do
printf '%s\r' "$(( --c ))"
## For more precision, comment the line below. This causes the
## script to wait for a second so it doesn't spam your CPU.
## If you need accuracy, comment the line out. Although, if you
## really need accuracy, don't use the shell for this.
sleep 1
done
done
或者,使用date
:
#!/bin/bash
waitTime=30;
## Infinite loop
while :; do
startTime=$(date +%s)
currentTime=$(date +%s)
c=$waitTime;
while [[ $((currentTime - startTime)) -lt $waitTime ]]; do
printf '%s\r' "$(( --c ))"
## For more precision, comment the line below. This causes the
## script to wait for a second so it doesn't spam your CPU.
## If you need accuracy, comment the line out. Although, if you
## really need accuracy, don't use the shell for this.
sleep 1
currentTime=$(date +%s)
done
c=0
echo "";
done
为了获得更高的精度,不要将日期存储在变量中:
#!/bin/bash
waitTime=4;
while :; do
startTime=$(date +%s)
c=$waitTime;
while [[ $(($(date +%s) - startTime)) -lt $waitTime ]]; do
printf '%s\r' "$(( --c ))"
sleep 1
done
c=0
done