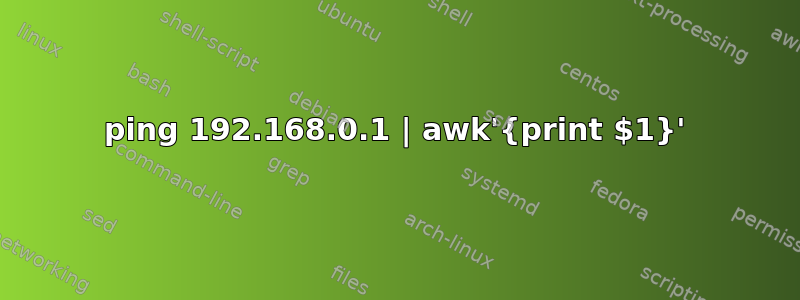
ping 192.168.0.1 | awk '{print $1}'
connect: Network is unreachable
我正在创建一个执行某些任务的脚本。因此,我想在无效主机名或 ping 命令生成的错误时显示适当的错误消息。
我想到修改使用上述命令生成的错误。我想做的是只打印错误而不是“ping”,但这并没有发生。谁能告诉我对此的补救措施。
答案1
如果您ping
只是用于确定给定主机名是否可达,那么您可以这样做:
if ping -c 1 "$otherhost" >/dev/null 2>&1; then
printf 'The host "%s" is reachable\n' "$otherhost"
else
printf 'The host "%s" is not reachable\n' "$otherhost"
fi
这会将单个ECHO_REQUEST
数据包发送到给定的主机,"$otherhost"
并使用 的退出状态来ping
确定实用程序是否设法执行其任务。所有普通输出都ping
被丢弃。
请注意,如果ping
无法查询另一台主机,这并不意味着该主机肯定已关闭或不可路由。它可能只是忽略该ECHO_REQUEST
数据包。
如果您想输出ping
失败时产生的实际错误,您可以通过以下方式执行此操作,以避免调用该实用程序两次(因为网络错误有时是暂时的,第二次调用ping
可能不会失败,或者可能会在一次调用中失败)不同的方式):
pingerr=$(mktemp)
if ping -c 1 "$otherhost" >/dev/null 2>"$pingerr"; then
printf 'The host "%s" is reachable\n' "$otherhost"
else
printf 'The host "%s" is not reachable\n' "$otherhost"
if [ -s "$pingerr" ]; then
echo 'ping error:'
cat "$pingerr"
fi
fi
rm -f "$pingerr"
这会将所有诊断消息保存在ping
临时文件中,如果ping
失败,该文件稍后会输出(如果其中有任何内容,这就是-s
测试的测试内容)。该文件将始终被创建(即使它保持为空),因此我们在if
- 语句之后将其删除。
使用此方法,您还可以以任何您想要的方式解析错误输出文件。例如:
pingerr=$(mktemp)
if ping -c 1 "$otherhost" >/dev/null 2>"$pingerr"; then
printf 'The host "%s" is reachable\n' "$otherhost"
else
printf 'The host "%s" is not reachable\n' "$otherhost"
if [ -s "$pingerr" ]; then
printf 'ping says'
sed 's/^[^:]*//' <"$pingerr"
fi
fi
rm -f "$pingerr"
在这里,我们不只是输出 的原始消息,而是在打印之前ping
删除第一个消息之前的位。:
答案2
它可能有助于定义和捕获您想要作为变量使用的所有内容:
$CMD_PING
- 您想要评估的命令(作为变量,更容易执行它并根据需要捕获结果)$CODE_EXIT
$CMD_PING
-评估时返回的退出代码(将不是0
发生错误时返回的代码)
例子:
#!/bin/bash
CMD_PING="ping -c1 does-not-exist"
EXEC_PING="$( $CMD_PING >/dev/null 2>&1 )" # STDOUT and STDERR go to /dev/null
CODE_EXIT="$?"
if [ ! 0 -eq "$CODE_EXIT" ]; then
# echo error only (or capture error for parsing)
# capture example: EXEC_PING="$( $CMD_PING >/dev/null )"
$CMD_PING >/dev/null # STDOUT goes to /dev/null, only STDERR prints
exit 1
fi
echo "success"
答案3
您的想法可行的唯一方法是将错误输出(stderr)与 stdout 交换,以将错误值发送到 awk (使用不存在的地址):
$ ping -c4 invalid.local. 3>&2 2>&1 1>&3 | awk '{print "Error ==> " $0}'
Error ==> ping: unknown host
但退出状态会变成awk的退出,通常应该是成功(0)。
如果您只想接收错误并根据 ping 的退出状态采取行动,您可以使用:
$ ping -c4 invalid.local. 2>&1 1>&- ; echo $?
ping: unknown host
1
$ ping -c4 127.0.0.1 2>&1 1>&- ; echo $?
0
所以,测试可以是:
if ping -c3 -w5 domain.com 2>&1 1>&- ; then
echo "ping was successful"
else
echo "ping failed with code $?"
fi