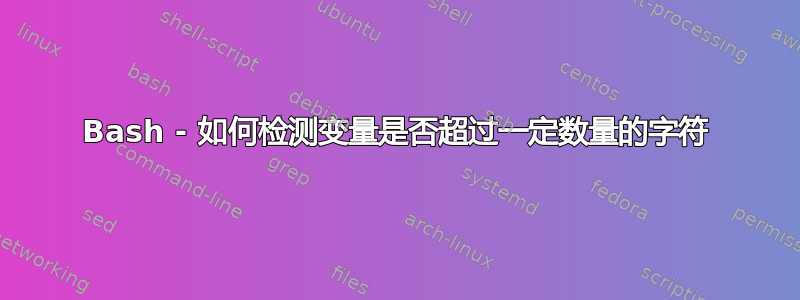
#/bin/bash
echo "This message may not be more than 8 characters"
read detect
我如何检测字母的数量?会是 IF 语句吗?
if #detect var
then
else
fi
编辑:如何让它重复直到用户输入正确的消息?
答案1
您可以使用如果-那么-否则-fi像这样:
if [[ ${#detect} -gt 8 ]] ; then
echo "Error message..."
exit 1
else
echo "Good to go..."
exit 0
fi
exit 1
通常表示失败,exit 0
通常表示成功。无论哪种情况,遇到时脚本都会立即结束,exit
因此请适当使用它们。
您还可以使用隐含如果-那么这对于 shell 语言来说相当独特:
[[ ${#detect} -gt 8 ]] && { echo "Error message..." ; exit 1 ; }
# successful code here
exit 0
如果您不需要错误消息,{ ... }
则单个命令不需要括号,例如:
[[ ${#detect} -gt 8 ]] && exit 1
可以用来表示“如果名为 detect 的变量 > 8 则退出”。
改善流程
一般来说,只给用户一次输入字符串的机会被认为是不礼貌的。礼貌的方法是告诉用户字符串长度不得超过 8 个字符,然后再次询问。例如:
echo "Enter character string 1 to 8 characters long or press <CTRL>+C to exit."
while True ; do
read StringVar
[[ ${#StringVar} -ge 1 ]] && [[ ${#StringVar} -le 8 ]] && break
echo "Sorry that string is ${#StringVar} long. Please try again."
done
# successful code here
在这种情况下,程序会不断请求输入,直到StringVar
获得变量,并且大于或等于 1 和这是小于或等于 8此时,while
外观会通过命令中断break
。
或者用户可以按Ctrl+C/kbd> 来终止 bash 脚本。
简洁的线条:
[[ ${#StringVar} -ge 1 ]] && [[ ${#StringVar} -le 8 ]] && break
echo "Sorry that string is ${#StringVar} long. Please try again."
... 可以像这样变得非常冗长:
if [[ ${#StringVar} -ge 1 ]] ; then
if [[ ${#StringVar} -le 8 ]] ; then
break
else
echo "Sorry that string is ${#StringVar} long. Please try again."
continue
fi
else
echo "Sorry that string is ${#StringVar} long. Please try again."
continue
fi
虽然这并不错误,但它浪费了程序员和系统的时间。
答案2
我假设您想反复要求用户输入,直到输入的长度小于 8 个字符。如果是这样,您可以使用while
循环而不是if-else
语句。这样,循环将无限次继续,直到输入的长度小于 8 个字符。只有当“输入”的长度小于 8 个字符时,脚本才会继续。代码将类似于:
#!/bin/bash
echo -n "Enter message with less than 8 characters: "
read detect
while [[ ${#detect} -gt 8 ]]
do
echo -n "Length of the message was greater than 8. Try again...: "
read detect
done
echo $detect
#and the rest of the code continues
此外,如果您从根()文件夹运行脚本/
,那么shebang#!bin/bash
可能会起作用(您在原始帖子),但如果您从其他地方运行它,请包含解释器的绝对路径,即/bin/bash
,因此将shebang修改为#!/bin/bash
。