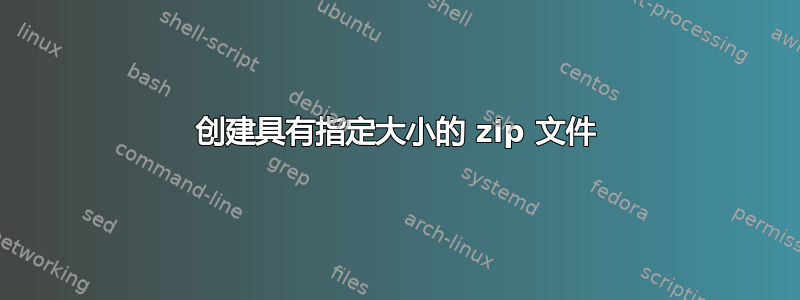
我的问题与创建多个互不依赖的 zip 文件?但我的想法是将文件添加到 zip 中,直到达到所需大小,然后继续为其他文件添加一个新的 zip 文件,依此类推。
知道如何在 bas 脚本中执行此操作吗? 这里的脚本,https://superuser.com/questions/614176/creating-a-bash-script-zipping似乎很有帮助。不过需要一些定制。
答案1
基本脚本只会检查 zip 文件的大小并相应地切换 zip 文件。如下所示:
#!/usr/bin/env bash
## This counter is used to change the zip file name
COUNTER=0;
## The maximum size allowed for the zip file
MAXSIZE=1024;
## The first zip file name
ZIPFILE=myzip"$COUNTER".zip;
## Exit if the zip file exists already
if [ -f $ZIPFILE ]; then
echo $ZIPFILE exists, exiting...
exit;
fi
## This will hold the zip file's size, initialize to 0
SIZE=0;
## Go through each of the arguments given in the command line
for var in "$@"; do
## If the zip file's current size is greater than or
## equal to $MAXSIZE, move to the next zip file
if [[ $SIZE -ge $MAXSIZE ]]; then
let COUNTER++;
ZIPFILE=myzip"$COUNTER".zip;
fi
## Add file to the appropriate zip file
zip -q $ZIPFILE $var;
## update the $SIZE
SIZE=`stat -c '%s' $ZIPFILE`;
done
注意事项:
- 该脚本预期文件,而不是目录,如果您希望它在目录上运行,请添加
-r
到zip
命令中。但是,它不会检查文件大小,直到每个目录已被压缩。 - 检查 zip 文件的大小后每次压缩。这意味着您将获得大于限制的文件。这是因为很难猜测文件的压缩大小,因此我无法在将其添加到存档之前进行检查。
答案2
编辑:有没有关于优化以下脚本以适应大量文件的想法?
#!/usr/bin/env bash
format="%b-%m.zip" # the default format
# see http://unixhelp.ed.ac.uk/CGI/man-cgi?date
dateformat="%Y-%b-%d" # the default date format
zipsize=2048; #256901120 #245MB
zipfile="" # the zip file name to use.
if [[ $# -lt 1 ]]; then
echo "Usage: $0 directory [format] [zip size] [dateformat]"
echo
echo "Where format can include the following variables:"
echo " %f file"
echo " %b file with no extention"
echo " %e file extention"
echo " %c file created date (may be 1-Jan-1970 UTC if unknown)"
echo " %m file modified date"
echo " %t current date"
echo
echo "And dateformat uses the same format specifiers as the date command."
echo
echo " Example: $0 zip-1 %f-%m.zip %Y"
echo " Example: $0 zip-1 %f-%m.zip %Y-%b"
echo
echo "And zipsize is the maximum zip size allowed per zip file in bytes."
echo
echo " Example: $0 zip-1 256901120 %f-%m.zip %Y"
echo " Example: $0 zip-1 256901120 %f-%m.zip %Y-%b"
exit 1
fi
if [[ $# -ge 2 ]]; then
zipsize="$2"
fi
if [[ $# -ge 3 ]]; then
format="$3"
fi
if [[ $# -ge 4 ]]; then
dateformat="$4"
fi
dozip()
{
filepath=$1
parent_path=$(dirname "$filepath")
file=$(basename "$filepath")
ext=${file##*.}
body=${file%.*}
date=$(date +$dateformat)
mdate=$(date --date="@$(stat -c %Y "$filepath")" +$dateformat)
cdate=$(date --date="@$(stat -c %W "$filepath")" +$dateformat)
if [ -z "$zipfile" ]; then
zipfile=$(echo $format | sed -e "s/%f/$file/g" -e "s/%b/$body/g" -e "s/%e/$ext/g" -e "s/%t/$date/g" -e "s/%m/$mdate/g" -e "s/%c/$cdate/g")
else
size=`stat -c '%s' $zipfile`
if [[ $size -ge $zipsize ]]; then
zipfile=$(echo $format | sed -e "s/%f/$file/g" -e "s/%b/$body/g" -e "s/%e/$ext/g" -e "s/%t/$date/g" -e "s/%m/$mdate/g" -e "s/%c/$cdate/g")
fi
fi
pushd "$parent_path" > /dev/null
zip "$zipfile" "$file" > /dev/null
popd > /dev/null
}
#files=$(find $1 -type f)
files=$(find $1 -type f | sed -e '/zip$/d') # exclude zip files
IFS=$'\n';
for file in $files; do
dozip $file
done